Working With Date and Time in Java
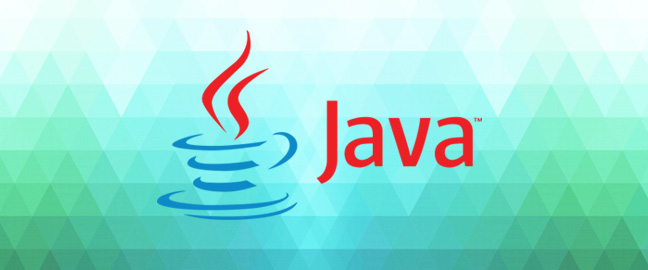
Date and Time are ingrained concepts in our lives. We need to keep track of time, we need to keep up with dates. Almost no application is practically usable if it has no sense of date and time. Whenever you are double clicking on a video file to watch it, your file explorer application is setting the last accessed time on it. When you are logging into your social account, it is keeping the record of your login time. You are saving your word document, the word processor saving that with a time stamp.
Working with date and time in other languages, for example C, can be grueling. But Java provides a Date class that makes working with date and time easy and fun.
Getting Started
To get started with coding for date and time you do not need anything special. You can use any IDE or code editor you want with JDK installed on your system. Create a project for working with date and time. Create a public class called JavaDateTime with a main function in it. You are free to name it anything else. So, now our initial code should look like the following:
import java.util.Date;
public class JavaDateTime {
public static void main(String[] args){
}
}
How Computer Counts Date and Time
Computers only understands 0 and 1. We make it understand other things and instructions by various types of abstraction. Computers do not keep track of date and time separately. They only keep track of a single number, which is the number of seconds or milliseconds from the epoch time or some other specific time in the timeline of human history. In our code we abstract that single number and represent that as hour, minute, second, year, month, day, etc.
In Java the most basic form of time is represented in milliseconds since epoch. The epoch time in Java is midnight, January 1, 1970.
Date Class
Don’t think on account of its name that this class only provides functionality for the date. It provides functionality for both date and time. The Date class from java.util package has two constructors. The parameter-less default constructor and another constructor that accepts one parameter. If you use the second constructor you need to pass time as milliseconds. Let's try this in code.
import java.util.Date;
public class JavaDateTime {
public static void main(String[] args){
Date d = new Date();
System.out.println(d);
}
}
Hit build and run to see the current time of your system displayed on the console.
Getting Time in Milliseconds
As discussed before, the most basic form of datetime in Java is in milliseconds. When we construct a Date object without a parameter it takes the current time of the system. To get the current time in milliseconds since midnight, January 1, 1970, we need to call the method getTime() on a Date object.
import java.util.Date;
public class JavaDateTime {
public static void main(String[] args){
Date d = new Date();
System.out.println(d.getTime());
}
}
Hit build and run to see the output as the current time as milliseconds since epoch.
Formatting Date and Time
It is not usually helpful to get the time as milliseconds or as the default string output with toString() on the Date object. For example, you may want to show the date and time in as bizarre a format as X seconds, Y hours, Z minutes of day A of Month B in Year C. To format date and time according to our needs, we need the class SimpleDateFormat from java.text package.
import java.util.Date;
import java.text.SimpleDateFormat;
public class JavaDateTime {
public static void main(String[] args){
Date d = new Date();
SimpleDateFormat sdf = new SimpleDateFormat("s 'seconds', h 'hours', m 'minutes' 'of' 'day' d 'of' 'Month' M 'in' 'Year' y");
System.out.println(sdf.format(d));
}
}
Hit build and run to see the desired output of the current time of your system.
Below I’ve listed different useful characters for formatting with SimpleDateFormat class.
- y : y represents year in four digits. For example, 1994.
- M : Capital M represents month number in the year. For example, it is 07 for July.
- d : d represents the day number in the month. For example, 11
- h : Hour in 12 hours clock.
- H : Hour in 24 hours clock.
- m : The minute in the hour.
- s : The second in the minute.
- S : The milliseconds in the second.
- E : The day of the week. For example, Saturday.
- D : The day in the year.
- F : Day of week in month 2 (second Wed. in July)
- w : The week number of the year. For example, 30.
- W : The number of week in the month. For example, 1.
- a : The AM/PM indicator. For example, PM.
- z : Time zone information.
- ' : Single quote escapes text inside format string.
- " : Double quote represents the single quote as the single quote is used for escaping normal text.
Parsing Date Time From String
It is not enough to just get date time information from the system with the help of the Date class. We often need to parse date & time from external sources as string and convert to Date object. The parse() method can be found on SimpleDateFormat.
import java.util.Date;
import java.text.SimpleDateFormat;
public class JavaDateTime {
public static void main(String[] args) throws Exception{
Date d;
SimpleDateFormat ft = new SimpleDateFormat ("yyyy-MM-dd");
String input = "2000-09-08";
d = ft.parse(input);
System.out.println(d);
}
}
Hit compile and run to see the following output:
Fri Sep 08 00:00:00 BDT 2000
Java has some other classes to work with date and time. Even Date and SimpleDateFormat has more functionality. All the aspects of datetime processing cannot be covered in a single article. I would cover more internal details in another article. You are advised to keep practicing and taking a look into the official documentation of Java to know more about the classes for handling date and time. Also check out this article on handling date and time in the C programming language.
Recent Stories
Top DiscoverSDK Experts
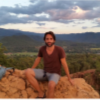
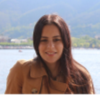
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
0 Comments
Your Comment