Pointers and Strings Examples
Command line input:
#include <stdio.h>
int main(int argc,char *argv[])
{
int i;
for (i=0;i<argc;i++)
printf("%s ",argv[i]);
printf("\n");
return 0;
}
Another example
#include <stdio.h>
int main(int argc,char *argv[])
{
int i;
for (i=1;i<argc;i++)
printf("%s ",argv[i]);
printf("\nName of file is: %s\n",argv[0]);
printf("Number of parameters :%d\n",argc);
return 0;
}
Example function for copying a signed integer into a string;
void ITOS(long x, char *ptr)
{
/* Convert a signed decimal integer to a string */
long pt[9] = { 100000000, 10000000, 1000000, 100000, 10000, 1000, 100, 10, 1 };
int n;
/* Check sign */
if (x < 0)
{
*ptr++ = '-';
/* Convert x to absolute */
x = 0 - x;
}
for(n = 0; n < 9; n++)
{
if (x > pt[n])
{
*ptr++ = '0' + x / pt[n];
x %= pt[n];
}
}
return;
}
more useful example of pointers
#include <stdio.h>
main()
{
int n;
int a[25];
int *x;
/* x is a pointer to an integer data type */
/* Assign x to point to array element zero */
x = a;
/* Assign values to the array */
for(n = 0; n < 25; n++)
a[n] = n;
/* Now print out all array element values */
for(n = 0; n < 25; n++ , x++)
printf("\nElement %d holds %d",n,*x);
}
using strings pointers and functions
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
main()
{
char data[80];
char *p;
char name[30];
int age;
printf("\nEnter your name and age ");
/* Read in a string of data */
gets(data);
/* P is a pointer to the last character in the input string */
p = &data[strlen(data) - 1];
/* Remove any trailing spaces by replacing them with null bytes */
while(*p == ' '){
*p = 0;
p--;
}
/* Locate last space in the string */
p = strrchr(data,' ');
/* Read age from string and convert to an integer */
age = atoi(p);
/* Terminate data string at start of age field */
*p = 0;
/* Copy data string to name variable */
strcpy(name,data);
/* Display results */
printf("\nName is %s age is %d",name,age);
}
pointers to function example
#include <stdio.h>
#include <math.h>
double (*fp[7])(double x);
void main()
{
double x;
int p;
fp[0] = sin;
fp[1] = cos;
fp[2] = acos;
fp[3] = asin;
fp[4] = tan;
fp[5] = atan;
fp[6] = ceil;
p = 4;
x = fp[p](1.5);
printf("\nResult %lf",x);
}
changing const array by redirection
#include <stdio.h>
int funca(const int x[])
{
int *ptr;
int n;
/* This line gives a 'suspicious pointer conversion warning' */
/* because x is a const pointer, and ptr is not */
ptr = x;
for(n = 0; n < 100; n++)
{
*ptr = n;
ptr++;
}
}
main()
{
int array[100];
int counter;
funca(array);
for(counter = 0; counter < 100; counter++)
printf("\nValue of element %d is %d",counter,array[counter]);
}
string functions using pointers
**********************************************************************/
//function to calculate the length of string
/**********************************************************************/
int strlen(char *s)
{
char *p=s;
while (*p++);
return p-s-1;
}
/**********************************************************************/
//function to copy one string to another
/**********************************************************************/
void strcpy(char *d,char *s)
{
while(*d++ = *s++);
}
/**********************************************************************/
//function to add one string to the end of the other
/**********************************************************************/
void strcat(char *d,char *s)
{
while (*d++);
d--;
while (*d++ = *s++);
}
//stristr() function
#include<stdio.h>
#include<string.h>
char *stristr(char *s1, char *s2)
{
char c1[1000];
char c2[1000];
char *p;
strcpy(c1,s1);
strcpy(c2,s2);
strupr(c1);
strupr(c2);
p = strstr(c1,c2);
if (p)
return s1 + (p - c1);
return NULL;
}
void main()
{
char *a="Haifa Computers";
printf("%s\n",stristr(a,"haifa"));
}
String Library
/**********************************************************************/
//string library
/**********************************************************************/
#include<string.h>
#include<stdio.h>
#include<conio.h>
#include<io.h>
/**********************************************************************/
//this function print message in the center of the screen
/**********************************************************************/
int center(char *str,int row)
{
int len=strlen(str);
if (len>80)
return -1;
gotoxy((80-len)>>1,row);
write(1,str,len);
return 0;
}
/**********************************************************************/
//this function take n characters from the right side of the source
//string and copy it to the destination string
/**********************************************************************/
char *rightstr(char *d,char *s,int num)
{
char *temp;
int len=strlen(s);
if (len<num)
return NULL;
temp=d;
s+=len-num;
while (*d++=*s++);
return temp;
}
/**********************************************************************/
//this function copy part of string src to string dest
/**********************************************************************/
char *midstr(char *d,char *s,int start,int num)
{
char *temp;
int len=strlen(s),offset;
if (len<num+start) return NULL;
if (num==0)
num=len-start+1;
temp=d;
offset=start-1;
do{
*d++=*(s+offset);
}while(*s++ && num--);
*(d-1)='\0';
return temp;
}
/**********************************************************************/
//this function delete all the charecters c in string s
/**********************************************************************/
void strdelc(char *s,char c)
{
while (*s)
{
if(*s==c)
strcpy(s,s+1);
else
s++;
}
}
/**********************************************************************/
//this function delete the char. c from string s ,just n times
/**********************************************************************/
void strdelcn(char *s,int n,char c)
{
while(*s)
{
if (*s==c && n)
{
strcpy(s,s+1);
n--;
}
else
s++;
}
}
/**********************************************************************/
//this function replace all the charecters old to new
/**********************************************************************/
void strrep(char *s,char newc ,char oldc)
{
char *ptr;
int len=strlen(s);
while (len)
{
ptr=(char *)memchr(s,oldc,len);//func. memchr returns pointer to char old if found
if (ptr)
{
*ptr=newc;
len-=(ptr-s);
s=ptr+1;
}
else
return;
}
}
void main(void)
{
clrscr();
char l[35],p[]="hello world what a nice day";
strrep(p,'p','l');
center(p,5);
getch();
}
An example program to reverse all the characters in a string
#include <stdio.h>
#include <string.h>
char *strrev(char *s)
{
/* Reverses the order of all characters in a string except the null */
/* terminating byte */
char *start;
char *end;
char tmp;
/* Set pointer 'end' to last character in string */
end = s + strlen(s) - 1;
/* Preserve pointer to start of string */
start = s;
/* Swop characters */
while(end >= s)
{
tmp = *end;
*end = *s;
*s = tmp;
end--;
s++;
}
return(start);
}
void main()
{
char text[100];
char *p;
strcpy(text,"This is a string of data");
p = strrev(text);
printf("\n%s",p);
}
example of strtok() function
#include <stdio.h>
#include <string.h>
void main()
{
char data[50];
char *p;
strcpy(data,"RED,ORANGE,YELLOW,GREEN,BLUE,INDIGO,VIOLET");
p = strtok(data,",");
while(p)
{
puts(p);
p = strtok(NULL,",");
};
}
/* using for loop *************************************\
for(strtok(data,","); p; p = strtok(NULL,","))
{
puts(p);
};
\******************************************************/
strupr and strlwr examples using pointers
#include<stdio.h>
#include<ctype.h>
#include<string.h>
void strupper(char *source)
{
char *p;
p = source;
while(*p)
{
*p = toupper(*p);
p++;
}
}
void strlower(char *source)
{
char *p;
p = source;
while(*p)
{
*p = tolower(*p);
p++;
}
}
void main()
{
char name[50];
strcpy(name,"Servile Software");
printf("\nName equals %s",name);
strupper(name);
printf("\nName equals %s",name);
strlower(name);
printf("\nName equals %s",name);
}
Recent Stories
Top DiscoverSDK Experts
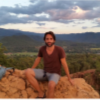
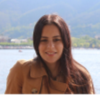
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment