Dynamic Memory Allocation
Simple Example
#include <conio.h>
#include <stdio.h>
#include <alloc.h>
void main()
{
int i,n;
int *parray,*p;
clrscr();
printf("Enter the size of a an array===>");
scanf("%d",&n);
parray=(int *)malloc(n*sizeof(int)) ;
if (parray==NULL)
{
printf("\nNot enough memory");
return;
}
for (p=parray,i=0; i<n ; i++)
scanf("%d",p++);
for (i=0; i<n; i++)
printf("\n%d",*(--p));
}
Memory Initialization
#include <conio.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <mem.h>
void main()
{
int i;
char *p;
clrscr();
p=(char *)malloc(50);
printf("Buffer after malloc:\n %s\n", p);
for(i=0;i<49;i++)
*(p+i)='*';
*(p+49)='\0';
printf("Buffer after loop:\n %s\n", p);
exit(0);
}
using memset
#include <conio.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <mem.h>
void main()
{
char *p;
clrscr();
p=(char *)malloc(50);
printf("Buffer after malloc:\n %s\n", p);
memset(p, '*',49); *(p+49)='\0';
printf("Buffer after memset:\n %s\n", p);
exit(0);
}
Dynamic sort of strings
/*----------------------------------------------------*/
/* T140.40 dynamic sort of strings */
/*----------------------------------------------------*/
#include <conio.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define LINES_SIZE 80
#define LINES_NO 500
void gen_line(char *line)
{
int i,len;
char ch;
len=rand()%(LINES_SIZE-1) +1;
for (i=0;i<len;i++)
{
ch =rand()%26 + 'A';
*line++=ch ;
}
*line='\0';
}
void main()
{
char *pline[LINES_NO];
char *swap_line;
int no_of_lines;
int i,j;
clrscr();
printf("Enter no of lines===>");
scanf("%d",&no_of_lines);
/*--------------------------------------
* generate and print lines before sort
*--------------------------------------*/
for (i=0;i<no_of_lines;i++)
{
pline[i]=(char *)malloc(LINES_SIZE);
if(pline[i]==NULL)
{ printf("Not enough memory");
exit(0);
}
gen_line(pline[i]);
puts(pline[i]);
}
/*-------------------------------------
* sort lines
*-------------------------------------*/
for(i=0;i<no_of_lines;i++)
{
for (j=i+1;j<no_of_lines;j++)
if(strcmp(pline[i],pline[j] )>0)
{ strcpy(swap_line,pline[i]);
strcpy(pline[i],pline[j]);
strcpy(pline[j],swap_line);
}
}
printf("\n the sorted output is:\n\n");
for (i=0;i<no_of_lines;i++)
{ puts(pline[i]);
free(pline[i]);
}
exit(0);
}
Simple malloc
#include <stdio.h>
#include <stdlib.h>
void main(void)
{
long nbr_students = 0;
long ctr;
char *student_name;
printf("\nHow many students will be entered? ==> ");
scanf("%D", &nbr_students);
fflush(stdin); /* clear out keyboard buffer */
student_name = (char *) malloc( 35*nbr_students);
if( student_name == NULL ) /* verify malloc() was successful */
{
printf( "\nError in line %3.3d: Could not allocate memory.",
__LINE__);
exit(1);
}
for( ctr = 0; ctr < nbr_students; ctr++ )
{
printf("\nEnter student %5.5d: ", ctr+1);
gets(student_name+(ctr*35));
}
printf("\n\nYou entered the following:\n");
for ( ctr = 0; ctr < nbr_students; ctr++ )
{
printf("\nStudent %5.5d:", ctr+1);
printf(" %s", student_name+(ctr*35));
}
/* this program does not release allocted memory! */
}
calloc example
#include <stdio.h>
#include <stdlib.h>
void main( void )
{
int nbr_grades = 0;
int total = 0;
int ctr;
int *student_grades;
while( nbr_grades < 1 || nbr_grades >= 10000 )
{
printf("\nHow many grades will be entered? ==> ");
scanf("%d", &nbr_grades);
fflush(stdin);
}
student_grades = (int *) calloc( nbr_grades, sizeof(int));
if( student_grades == NULL )
{
printf( "\nError in line %3.3d: Could not allocate memory.",
__LINE__);
exit(1);
}
for( ctr = 0; ctr < nbr_grades; ctr++ )
{
printf("\nEnter grade %4.4d: ", ctr+1);
scanf("%d", student_grades+ctr);
}
printf("\n\nYou entered the following:\n");
for ( ctr = 0; ctr < nbr_grades; ctr++ )
{
printf("\nGrade %4.4d:", ctr+1);
printf(" %d", student_grades[ctr]);
total += student_grades[ctr];
}
printf("\n\nThe average grade is: %d\n\n", (total/nbr_grades));
/* Free allocated memory */
free(student_grades);
}
matrix allocation
#include<stdio.h>
#include<stdlib.h>
#include<conio.h>
void main()
{
int **a,i,j;
clrscr();
a=(int **)malloc(sizeof(int *)*10);
for (i=0;i<10;i++)
a[i]=(int *)malloc(20);
for (i=0;i<10;i++)
for(j=0;j<10;j++)
a[i][j]=(i+1)*(j+1);
for (i=0;i<10;i++)
{
for(j=0;j<10;j++)
printf("%4d",a[i][j]);
printf("\n");
}
for (i=0;i<10;i++)
free(a[i]);
}
realloc example
#include <stdio.h>
#include <stdlib.h>
#define NAME_SIZE 35
void main(void)
{
long student_ctr = 0;
long ctr;
char *student_name = NULL;
while( (student_name = realloc( student_name, (NAME_SIZE * (student_ctr+1)))) != NULL )
{
printf("\nEnter student %5.5d: ", student_ctr+1);
gets(student_name+( student_ctr * NAME_SIZE));
if( student_name[student_ctr * NAME_SIZE] == NULL )
{
break;
}
else
{
student_ctr++;
}
}
printf("\n\nYou entered the following:\n");
for ( ctr = 0; ctr < student_ctr; ctr++ )
{
printf("\nStudent %5.5d:", ctr+1);
printf(" %s", student_name+(ctr*NAME_SIZE));
}
free(student_name);
}
Recent Stories
Top DiscoverSDK Experts
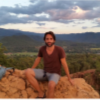
Mendy Bennett
Experienced with Ad network & Ad servers.
Mobile | Ad Networks and 1 more
View Profile
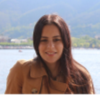
Karen Fitzgerald
7 years in Cross-Platform development.
Mobile | Cross Platform Frameworks
View Profile
X
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}Now comparing:
{{product.ProductName | createSubstring:25}} X
{{CommentsModel.TotalCount}} Comments
Your Comment