Working with files in Ruby
In all the previous guides on Ruby, we displayed information on the command line screen. We did not save it anywhere and as a result it was all gone just after closing the window. Again, in the examples where we needed input data, we had to do it by hand, we could not take it from other external sources—just hard coded data inside source code.
In real life things do not work like this. After we close our application and reopen it sometime later, we want to keep things saved by our programs. We need to persist data somewhere to go back in time.
In this article I will show you how to read, write, and do other things with files in Ruby programming language.
Before You Begin
Check the following list to move forward with this article.
- You should have basic knowledge of working with Ruby. You should be familiar with its basic syntax.
- You should have read previous articles and tutorials in this series of Ruby programming.
- You should have Ruby installed on your system.
- You need to have some kind of plain text editor or code editor present in your system.
- You should have some familiarity working with command lines.
Preparing Working Environment
To get started with the examples shown in this article, you need to do the following:
- Create or choose a directory where you want to keep your Ruby files.
- Open the command line in this directory or point your command line's current working directory to this one.
- Create a file with name io.rb in this directory.
- Run the Ruby file by invoking ruby io.rb through command line to check if everything is alright. As we haven't yet written any code, we will not have anything printed on the screen.
Creating an Empty File
Let's start our work with files in Ruby by creating an empty file in our working directory. To create a file we need to use the File class. In the constructor we need to pass the file name as the first parameter and the mode in which we want to open the file as the second parameter.
f = File.new "readme.txt", "w"
Run io.rb. This will not show anything on the screen. Go to your working directory and you will see a new file there with file name readme.txt. Open this file with a text editor and you will see that it is empty.
Note:
The mode parameter "w" means write here.
This is not the end of the story - we still need a lot of things to do. But to keep it simple I am finishing this section with that one line of code.
Closing a File
Every open file eats up some limited system resource. If you open a lot of files and do not close them after performing some action on them then the system will fall short in significant amount of resource. Even operating systems has limitation on how many number of files it can keep open. So, if your application eats a lot of them then other application will not be able to get them as needed. To close a file you need to invoke the close method on file objects. So, from out previous example, we should have closed the file like below:
f = File.new "readme.txt", "w"
f.close
All open files are closed when the process exits. In our example only one line was executed and then the process exited. So, for such a simple thing there we no problem.
Also remember that if you open a file in writing mode and if you do not close that and the process continues to run, other programs will not be able to use that file.
Writing to a File
To write to a file we need to open it in either write or update mode. To write contents to the file we will have to invoke the syswrite method on the open file object. And as a good practice do not forget to close the file. Let's see an example:
f = File.new "readme.txt", "w"
f.syswrite "I am single line of text"
f.close
Run this and go to your working directory. Open the readme.txt file. You will see "I am single line of text" inside of it.
Reading from a File
To read from a file we need to open it with read mode. The mode string for that is "r". Let's open the same file we wrote to. We want to read the content we wrote there. To read contents from a file we need to invoke the sysread method. We need to pass a parameter to the method indicating the maximum length of content we want to read from there. In this case I have indicated maximum 100 characters.
f = File.new "readme.txt", "r"
content = f.sysread 100
f.close
puts content
Outputs:
I am single line of text
Renaming a File
Renaming a file in Ruby is as easy as just invoking a method. To rename a file we need to invoke the rename method on the File class. As the first parameter we need to provide the file name that we want to rename and as the second parameter we need to indicate the new name we want to give to it. Let's rename the readme.txt to readme2.txt.
File.rename "readme.txt", "readme2.txt"
Look at the working directory and you will see no existence of readme.txt there. The file is renamed to readme2.txt.
Deleting a File
Deleting a file is as easy as renaming it. delete method present on the File class is responsible for deleting files. Let's delete our renamed readme2.txt file.
File.delete "readme2.txt"
After executing this go to your working directory and you will see no existence of readme2.txt there.
Conclusion
There are a lot of methods on the File class and a numerous ways of interacting with the file system, files, and directories. Have a quick look at the official documentation of the File class. I will write more articles and tutorials on IO in future. Keep practicing.
Recent Stories
Top DiscoverSDK Experts
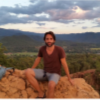
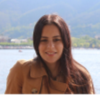
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment