Understanding Arrays in Ruby
The most basic way of storing data of some type and referring back to it in various places in Ruby code is with variables. We need to create variables by hand. If we are making employee management software, we have a changeable amount of employees. It would be not just hard, but really impossible to keep each employee’s details in variables.
We need some way to store them in a container and in this way we can refer to that container with a single variable. We also need ways to add, modify, delete records from that container. In this guide I am going to discuss different aspects of Arrays in Ruby.
Arrays are ordered collections of objects in Ruby. You can think of it like a list. Unlike many other languages arrays in Ruby are dynamically sized. Another great part is that you can put any type of data into arrays.
Arrays can be created by surrounding zero or more objects/values separated by a pair of square brackets. See an example below:
['a str', 100, 5.66, "anotherr string"]
Note:
Everything in Ruby is object. So, the things that stay in arrays are objects. In this article I will refer to them as objects, values or elements. So, do not be confused by different terms.
Before You Begin
Here is a list of what you need to have done before moving forward:
- Make sure you have Ruby installed on your system.
- You should be familiar with using the command line on your system.
- You must have a plain text editor or a code editor to write Ruby codes. You can also use IDEs if you like.
- You should have read previous posts on Ruby in this series.
Preparing Environment
- Choose or create a directory where you would like to put your Ruby files.
- Open your command line in that directory. That means, your command line's current working directory must be this one.
- Create a file named array.rb in this directory.
- Open the Ruby file with your preferred directory.
Creating an Array
In the introduction section I have already described the method of creating arrays in Ruby. Let's assign the same array to a variable and display that on the screen by running array.rb:
arr = ['a str', 100, 5.66, "anotherr string"]
puts arr
Outputs:
a str
100
5.66
anotherr string
To get a similar output of what we wrote in code use print:
arr = ['a str', 100, 5.66, "anotherr string"]
print arr
Outputs:
["a str", 100, 5.66, "anotherr string"]
An empty array can be created by opening and closing square brackets and no elements or objects inside of it:
[]
An array object can also be created by instantiating the Array class like below:
arr = Array.new
We can create an array of predefined number of elements by passing the number to the constructor of the Array class:
arr = Array.new 10
print arr
Outputs:
[nil, nil, nil, nil, nil, nil, nil, nil, nil, nil]
We did not provide any values for those 10 elements and thus it was populated with nil. We can provide a default value for each of those positions as the second parameter to the constructor:
arr = Array.new 10, "hello"
print arr
Outputs:
["hello", "hello", "hello", "hello", "hello", "hello", "hello", "hello", "hello", "hello"]
Array Size/Length
The length of an array indicates how many objects that array holds. As arrays in Ruby are dynamically sized this length changes at runtime by different mutating operations. To get the length of an array invoke the length method on it:
arr = ["hello", "hello", "hello", "hello", "hello", "hello", "hello", "hello", "hello", "hello"]
print arr.length
Outputs:
10
Note:
You can also use size method to find the length or size of an array.
Accessing Elements of Arrays
As I told before, arrays are an ordered collection. Each element or object in an Array has a position. These positions are integers. Arrays in Ruby are zero indexed. That means, the first element of an array has the position of 0. To access an element of an array, use this syntax: variable[index]. Let's try with an example:
arr = ["hello", "world", "I", "am", "a", "programmer"]
print arr[0]
Outputs:
hello
Modifying Array Elements by Index
You can modify the value of certain position of an array, the initial code is the same as accessing it. Let's say, you want to modify the value of the second position of an array. So, you need to access it via variable_name[index] and then you have to put the value after an equal sign.
arr = ["hello", "world", "I", "am", "a", "programmer"]
arr[1] = "alien"
print arr
Outputs:
["hello", "alien", "I", "am", "a", "programmer"]
Adding Elements to Arrays
We use arrays when we need to store more than a few values where using variables is not practical. So, there is no good point in using arrays if we cannot add new elements to them.
To add an element to the end of an array we use the push method with the object that we want to add as a parameter.
arr = [1, 2]
arr.push 3
print arr
Outputs:
[1, 2, 3]
Instead of using the push method, you can use the << operator to serve the same purpose.
arr = [1, 2]
arr << 3
print arr
Outputs:
[1, 2, 3]
To add elements at the beginning of elements, you need to use the unshift method.
arr = [1, 2]
arr.unshift 3
print arr
Outputs:
[3, 1, 2]
Deleting Elements
Deleting is as important and as easy as adding elements to arrays. There are more than one way of deleting elements in arrays. I am demonstrating a few here. We can delete elements by index with the help of delete_at method:
arr = [1, 2]
arr.delete_at 0
print arr
Outputs:
[2]
If you want to delete elements from the end of an array, use the pop method:
arr = [1, 2, 3, 4, 5]
arr.pop 0
print arr
Outputs:
[1, 2, 3, 4]
Conclusion
We are moving forward to advanced topics in Ruby programming day by day in this series. You must practice every example yourself to keep pace with these articles and tutorials. Next I am going to discuss about different array methods.
Be sure to stop by the homepage to search and compare SDKs, Dev Tools, and Libraries.
Recent Stories
Top DiscoverSDK Experts
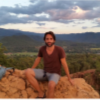
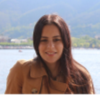
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment