React Native - State and Props
There are two types of data that reside in a component: props and state. Props are set by the parent and they are fixed throughout the lifetime of a component. For data which is expected or can change, we can use state.
States
The state is mutable while props are immutable. This means that state can be modified in the future while props can't.
So, we can initialize state in the constructor, and then call setState whenever we want to change it.
For example, let's say that we want to make text that blinks all the time. The text itself gets set once when the blinking component gets created, so the text itself is a prop. The "whether the text is currently on or off" changes over time, so that should be kept in state.
Simple Example
Let us construct a simple example where we set text to a textview field. This can be done using a state because the text is fixed.
import React, { Component } from 'react';
import {
Text,
View
} from 'react-native';
export default class MyContainerComponent extends Component {
constructor() {
super()
this.state = {
myText: 'Lorem ipsum.'
}
}
render() {
return (
<View>
<Text>
{this.state.myText}
</Text>
</View>
);
}
}
We can update the state of a textview. Let’s hide the text once the textview is clicked upon.
import React, { Component } from 'react'
import {
Text,
View
} from 'react-native'
export default class reactTutorialApp extends Component {
constructor() {
super()
this.state = {
myText: 'Lorem ipsum.'
}
}
deleteText = () => {
this.setState({myText: ''})
}
render() {
return (
<View>
<Text onPress = {this.deleteText}>
{this.state.myText}
</Text>
</View>
);
}
}
We will set state whenever we have new data arrive from the server, or from user input. We can also use a state container like Redux to control our data flow. In that case we will use Redux to modify our state rather than calling setState directly.
State works the same way as it does in React, so for more details on handling state, you can look at the React.Component API.
Props
Presentational components should get all data by passing props. Only container components should have state.
Most components can be customized when they are created, with different parameters. These creation parameters are called props.
For example, one simple React Native component is the Image. When we create an image, we can use a prop named source to control what image it shows. In this example, we will load an image over a network.
import React, { Component } from 'react';
import { AppRegistry, Image } from 'react-native';
class Bananas extends Component {
render() {
let pic = {
uri: 'https://upload.wikimedia.org/wikipedia/commons/d/de/Bananavarieties.jpg'
};
return (
<Image source={pic} style={{width: 193, height: 110}}/>
);
}
}
AppRegistry.registerComponent('Bananas', () => Bananas);
Notice that {pic} is surrounded by braces to embed the variable pic into JSX. We can put any JavaScript expression inside braces in JSX.
Our own components can also use props. This lets us make a single component that is used in many different places in our app, with slightly different properties in each place. We just need to refer to this.props in our render function. Let us look at an example:
import React, { Component } from 'react';
import { AppRegistry, Text, View } from 'react-native';
class Bike extends Component {
render() {
return (
<Text>Hello {this.props.bike}!</Text>
);
}
}
class LotsOfBikes extends Component {
render() {
return (
<View style={{alignItems: 'center'}}>
<Bike bike=Harley />
<Bike bike=Hudson />
<Bike bike=Bugati />
</View>
);
}
}
AppRegistry.registerComponent('LotsOfBikes', () => LotsOfBikes);
Using name as a prop lets us customize the Greeting component, so we can reuse that component for each of our greetings. This example also uses the Greeting component in JSX, just like the built-in components. The power to do this is what makes React so cool—if you find yourself wishing that you had a different set of UI primitives to work with, you just invent new ones.
The other new thing going on here is the View component. A View is useful as a container for other components, to help control style and layout.
With props and the basic Text, Image, and View components, you can build a wide variety of static screens.
Styling the components
With React Native, we don't use some other special language or syntax for defining styles. We just style our apps using JavaScript. All of the core components accept a prop named style. The style names and values mostly match how CSS works on the web, except names are written using camel casing, e.g paddingLeft rather than padding-left.
The style prop can be a plain old JavaScript object. That's the simplest and what we usually follow for sample code. We can also pass an array of styles—the last style in the array has precedence, so we can use this to inherit styles.
As a component grows in complexity, it is often cleaner to use StyleSheet.create to define several styles in one place. Here's an example:
import React, { Component } from 'react';
import { AppRegistry, StyleSheet, Text, View } from 'react-native';
class LotsOfStyles extends Component {
render() {
return (
<View>
<Text style={styles.red}>just red</Text>
<Text style={styles.bigblue}>just bigblue</Text>
<Text style={[styles.bigblue, styles.red]}>bigblue, then red</Text>
<Text style={[styles.red, styles.bigblue]}>red, then bigblue</Text>
</View> );
}
}
const styles = StyleSheet.create({
bigblue: {
color: 'blue',
fontWeight: 'bold',
fontSize: 30,
},
red: {
color: 'red',
},
});
AppRegistry.registerComponent('LotsOfStyles', () => LotsOfStyles)
One common pattern is to make our component accept a style prop which in turn is used to style subcomponents. We can use this to make styles "cascade" the way they do in CSS. This will also lead to making a modular design analogy which can be reused in many places with other components.
There are a lot more ways to customize text styles. Check out the Text component reference for a complete list.
Recent Stories
Top DiscoverSDK Experts
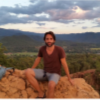
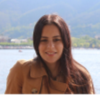
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment