React Native Basic Mobile Components
In this lesson, we will give a detailed overview of the most basic components in React Native: <View>, <Image>, and <Text>. Then we’ll discuss how touch and gestures factor into React Native components and how to handle touch events. Next we will cover higher-level components, such as the <ListView>, <TabView>, and <NavigatorView>, which allow you to combine other views into standard mobile interface patterns.
HTML Elements and Native Components
When developing for the Web, we make use of a variety of basic HTML tags. These include <div>, <span>, and <img>, as well as organizational elements such as <ol>, <ul>, and <table>.
HTML |
React Native |
<div> |
<View> |
<ul>, <li> |
<Listview>,. Child items |
<span>, <a> |
<Text> |
<img> |
<Image> |
Although these elements serve roughly the same purposes, they are not interchangeable.
The Text Component
Rendering text is a deceptively basic function; nearly any application will need to render text somewhere. However, text within the context of React Native and mobile development works differently from text rendering for the Web.
When working with text in HTML, you can include raw text strings in a variety of elements. Furthermore, you can style them with child tags such as <strong> and <em>. So, you might end up with an HTML snippet that looks like this:
<p>The quick <em>brown</em> fox jumped over the lazy <strong>dog</strong>.</p>
In React Native, only <Text> components may have plain text nodes as children. In other words, this is not valid:
<View>
Text doesn't go here!
</View>
Instead, wrap your text in a <Text> component:
<View>
<Text>This is OK!</Text>
</View>
When dealing with <Text> components in React Native, you no longer have access to subtags such as <strong> and <em>, though you can apply styles to achieve similar effects through use of attributes such as fontWeight and fontStyle. Here’s how you might achieve a similar effect by making use of inline styles:
<Text>
The quick <Text style={{fontStyle: "italic"}}>brown</Text> fox
jumped over the lazy <Text style={{fontWeight: "bold"}}>dog</Text>.
</Text>
This approach could quickly become verbose. You’ll likely want to create styled components as a sort of shorthand when dealing with text, as shown in Example 4-1.
Example 4-1. Creating reusable components for styling text
var styles = StyleSheet.create({
bold: {
fontWeight: "bold"
},
italic: {
fontStyle: "italic"
}
});
var Strong = React.createClass({
render: function() {
return (
<Text style={styles.bold}>
{this.props.children}
</Text>);
}
});
var Em = React.createClass({
render: function() {
return (
<Text style={styles.italic}>
{this.props.children}
</Text>);
}
});
Once you have declared these styled components, you can freely make use of styled nesting. Now the React Native version looks quite similar to the HTML version.
Example 4-2. Using styled components for rendering text
<Text>
The quick <Em>brown</Em> fox jumped
over the lazy <Strong>dog</Strong>.
</Text>
Similarly, React Native does not inherently have any concept of header elements (h1, h2, etc.), but it’s easy to declare your own styled <Text> elements and use them as needed.
In general, when dealing with styled text, React Native forces you to change your approach. Style inheritance is limited, so you lose the ability to have default font settings for all text nodes in the tree. Once again, Facebook recommends solving this by using styled components:
you also lose the ability to set up a default font for an entire subtree. The recommended way to use consistent fonts and sizes across your application is to create a component MyAppText that includes them and use this component across your app. You can also use this component to make more specific components like MyAppHeaderText for other kinds of text.
React Native Documentation
The Text component documentation has more details on this.
You’ve probably noticed a pattern here: React Native is very opinionated in its preference for the reuse of styled components over the reuse of styles. We’ll discuss this further in the next lesson.
The Image Component
If text is the most basic element in an application, images are a close second for both mobile and for the Web. When writing HTML and CSS for the Web we include images in a variety of ways: sometimes we use the <img> tag, while at other times we apply images via CSS, such as when we use the background-image property. In React Native, we have a similar <Image> component, but it behaves a little differently.
The basic usage of the <Image> component is straightforward; just set the source prop:
<Image source={require('image!puppies')} />
How does that require call work? Where does this resource live? Here’s one part of React Native that you’ll have to adjust based on which platform you’re targeting. On iOS, this means that you’ll need to import it into the assets folder within your Xcode project. By providing the appropriate @2x and @3x resolution files, you will enable Xcode to serve the correct asset file for the correct platform. This is a nice change from web development: the relatively limited possible combinations of screen size and resolution on iOS means that it’s easier to create targeted assets.
For React Native on other platforms, we can expect that the image! require syntax will point to a similar assets directory.
It’s worth mentioning that it is also possible to include web-based image sources instead of bundling your assets with your application. Facebook does this as one of the examples in the UIExplorer application:
<Image source={{uri: 'https://facebook.github.io/react/img/logo_og.png'}}
style={{width: 400, height: 400}} />
When utilizing network resources, you will need to specify dimensions manually.
Downloading images via the network rather than including them as assets has some advantages. During development, for instance, it may be easier to use this approach while prototyping, rather than carefully importing all of your assets ahead of time. It also reduces the size of your bundled mobile application, so that users needn’t download all of your assets. However, it means that instead you’ll be relying on the user’s data plan whenever they access your application in the future. For most cases, you’ll want to avoid using the URI-based method.
Because React Native emphasizes a component-based approach, images must be included as an <Image> component instead of being referenced via styles. For instance, we wanted to use an image as a background for our weather application. Whereas in plain HTML and CSS you would likely use the background-image property to apply a background image, in React Native you instead use the <Image> as a container component, like so:
<Image source={require('image!puppies')}>
{/* Your content here... */}
</Image>
Styling the images themselves is fairly straightforward. In addition to applying styles, certain props control how the image will be rendered. You’ll often make use of the resizeMode prop, for instance, which can be set to resize, cover, or contain. The UIExplorer app demonstrates this well.
The <Image> component is easy to work with, and very flexible. You will likely make extensive use of it in your own applications.
Recent Stories
Top DiscoverSDK Experts
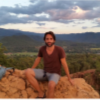
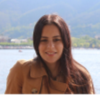
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment