Intro to Arrays in JavaScript
The array is one of the most important data structure in JavaScript without which almost no practical JavaScript application is possible. Arrays are objects that can store any type of JavaScript data in an ordered manner.
Creating a Problem to Understand Better
Let's first create a problem to understand why arrays are needed in JavaScript programming. Let's say that we need to work with some country names around the world. Let's take 5 countries for example.
var country1 = "Afghanistan";
var country2 = "Albania";
var country3 = "Algeria";
var country4 = "Andorra";
var country5 = "Angola";
Here, we are hard coding five country names as strings in our code. When we hard code these names we can use them inside our code easily. But even if you try to display them on the screen you need to write exactly five console.log() functions with the country variable names as the parameter to it (or you can concatenate them all with the + operator and use a single console.log()). You cannot even loop over a set of data just to avoid the hard coding.
To make things even worse, let's say that we need the data from user input and in different languages (for making our application multilingual). Now, we have different requirements for the data set. So, how do we store them in memory? We cannot create variables at runtime unless you think about that evil eval! Let's say, you can create variables at runtime—then how do you plan to code your program to use those dynamically created variables! Maybe with trying hard and with some evil tricks we can use them too—and to do the evil tricks we may need an array again. So, we cannot get rid of arrays.
To avoid hard coding, adding data to a collection dynamically, searching inside it, removing data from it, looping over the collection, and to solve a whole lot of other problems we need arrays. If we keep talking about how impossible it would be to create a practical JavaScript application this article would never end.
Solving the Problem With Arrays
Let's rewrite the code for the list of country names in an Array.
var countries = [
"Afghanistan",
"Albania",
"Algeria",
"Andorra",
"Angola"
];
Now, we can use the list with ease. We can add to it, we can remove from it, we can search through it, we can iterate over it. All will be easy and perfect and new ideas will be implemented with the help of it. Let's see what we can do with it.
Creating Arrays
Arrays can be created by putting values separated by commas inside square braces []. You may wish to create an empty array just by putting the opening and closing square braces with no values inside them. You can create it in another way. Create an array object with the new keyword followed by Array() and optional initial values inside the round braces separated by commas. We have seen how to create literal arrays in the previous example.
var countries = new Array("Afghanistan", "Albania", "Algeria", "Andorra", "Angola");
Many functions in JavaScript return arrays. Even some native code in the hosting environment can return JavaScript arrays.
Finding the Size of an Array
Arrays are dynamically sized in JavaScript and you may often need to know how many elements there are in an array at different points of time. To find how many elements an array is holding, just refer to the length attribute of the array object.
var arr = new Array("Afghanistan", "Albania", "Algeria", "Andorra", "Angola");
console.log(arr.length);
Executing the code outputs 5.
Accessing Elements of Arrays
Elements of an array are accessed by it's index. The first element of an array is indicated by index zero (0) and so on. So, if an array contains 5 elements and you need to access or read the last one, you need to use index 4. Let's read all the elements from an array and print them by looping over it.
var arr = new Array("Afghanistan", "Albania", "Algeria", "Andorra", "Angola");
for(var i=0; i < arr.length; i++){
console.log(i + " : " + arr[i]);
}
Outputs:
0 : Afghanistan
1 : Albania
2 : Algeria
3 : Andorra
4 : Angola
Adding Elements to Arrays
Unlike many other languages, arrays in JavaScript are not of fixed sizes. You can add as many elements you want until your computer's memory is exhausted. To add an element at the end of the array, invoke the push() method on it. Use unshift() to add at the beginning of the array.
var arr = [];
console.log("Initial Array: ", arr);
arr.push("One");
arr.push(2);
console.log("Array after pushing two elements: ", arr);
arr.unshift("Zero");
console.log("Array after adding one element at the beginning of the array: ", arr);
Outputs:
Initial Array: []
Array after pushing two elements: [ 'One', 2 ]
Array after adding one element at the beginning of the array: [ 'Zero', 'One', 2 ]
Removing Elements From Arrays
As with reading from an array and adding elements to it, removing elements from it carries equal importance. There are few ways you can remove elements from arrays.
To remove elements by their index, you need to use the splice() method. As the first argument, pass the index and as the second argument pass how many elements you want to remove.
var arr = new Array("Afghanistan", "Albania", "Algeria", "Andorra", "Angola");
arr.splice(0, 1);
console.log(arr);
Outputs:
[ 'Albania', 'Algeria', 'Andorra', 'Angola' ]
Look at the output—the first element is gone.
If you want to remove the very last element from the array, you can use splice() with the arr.length - 1 as the argument. But, we have a better way to do that. You can use pop() to remove the last element with the last element returned from the method. To remove the first element from the array we can use shift() with the first element returned after removing.
Conclusion
This article was just the basic of arrays in JavaScript. In future articles I will show you how to use different methods to carry out different operations. Note that you should be very comfortable using arrays in JavaScript before moving on to real life application development.
Recent Stories
Top DiscoverSDK Experts
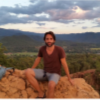
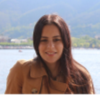
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment