Hibernate Annotations
In this article, we’ll take a look at Hibernate annotations because XML is so old now. Also, Java annotations make our code type-safe and make typos avoidable.
Hibernate is a complete solution for the persistence module in a Java project. It handles the application interaction with the database, leaving the developer free to concentrate more on business logic and solving complex problems.
Introduction
So far, we have seen XML in action whenever we wanted to provide Hibernate with metadata and information about the mappings in our entity classes.
Now, hibernate annotations are the newest solutions to provide mapping information and other metadata to Hibernate. The best thing is that all metadata is clubbed with the Java POJO class which means that all information related to an entity can be configured in a single file.
Using annotations as our configure method is just a convenient method instead of copying the endless XML configuration file.
If we are going to make our application portable to other EJB3 compliant ORM applications, we must use annotations to represent the mapping information. But if we still want greater flexibility then we should go with XML-based mappings.
Maven configuration
To start quickly, we will add the necessary Maven dependencies to our project.
Firstly, we will be making our project EJB3 compliant so we’ll also add the following dependency:
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>ejb3-persistence</artifactId>
<version>3.3.2.Beta1</version>
</dependency>
Next, let’s add the remaining two Hibernate dependencies.
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-annotations</artifactId>
<version>3.5.6-Final</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-commons-annotations</artifactId>
<version>3.3.0.ga</version>
</dependency>
When adding these dependencies, make sure you add the latest version of these dependencies.
If instead of using maven you want to add JAR files, we will need to install the Hibernate 3.x annotations distribution package, available from sourceforge (Download Hibernate Annotation) and copy hibernate-annotations.jar, lib/hibernate-commons-annotations.jar and lib/ejb3-persistence.jar from the Hibernate Annotations distribution to our CLASSPATH.
Annotated POJO class
As discussed in previous examples, we will be using the same class, Student with the following SQL schema:
create table Student (
id INT NOT NULL auto_increment,
first_name VARCHAR(20) default NULL,
last_name VARCHAR(20) default NULL,
grade INT default NULL,
PRIMARY KEY (id)
);
Finally, let’s have a look at our annotated class.
import javax.persistence.*;
@Entity
@Table(name = "Student")
public class Student {
@Id
@GeneratedValue
@Column(name = "id")
private int id;
@Column(name = "first_name")
private String firstName;
@Column(name = "last_name")
private String lastName;
@Column(name = "grade")
private int grade;
public Employee() {}
public int getId() {
return id;
}
public void setId( int id ) {
this.id = id;
}
public String getFirstName() {
return firstName;
}
public void setFirstName( String first_name ) {
this.firstName = first_name;
}
public String getLastName() {
return lastName;
}
public void setLastName( String last_name ) {
this.lastName = last_name;
}
public int getGrade() {
return grade;
}
public void setGrade(int grade) {
this.grade = grade;
}
}
Now that was easy when compared to XML mappings.
Let’s discuss all the annotations we used in the above example one by one:
- The @Id annotation is inherited from javax.persistence.Id, indicating the member field below is the primary key of the current entity. Hence our Hibernate and spring framework as well as we can do some reflect works based on this annotation.
- The @GeneratedValue annotation is to configure the way of increment of the specified column(field). For example when using Mysql, we may specify auto_increment in the definition of a table to make it self-incremental, and then use
in the Java code to denote that we also acknowledged to use this database server side strategy. Also, we may change the value in this annotation to fit different requirements.@GeneratedValue(strategy = GenerationType.IDENTITY)
- @Entity annotation defines that a class can be mapped to a table. And that is it, it is just a marker, like for example Serializable interface.
And why is @Entity annotation mandatory? Well, it is how JPA is designed. When we create a new entity we have to do at least two things:
- annotate it with @Entity
- create an ID field and annotate it with @Id
- The @Table annotation allows you to specify the details of the table that will be used to persist the entity in the database. It provides four attributes, allowing you to override the name of the table, its catalogue, and its schema, and enforce unique constraints on columns in the table.
- @Column allows us to specify the name of the column in the database to which the attribute is to be persisted. This annotation is not compulsory though. We can use column annotation with the following four commonly used attributes:
- name attribute permits the name of the column to be explicitly specified.
- length attribute permits the size of the column used to map a value particularly for a String value.
- nullable attribute permits the column to be marked NOT NULL when the schema is generated.
- unique attribute permits the column to be marked as containing only unique values.
Database configuration
Now let us create hibernate.cfg.xml configuration file to define database related parameters.
<?xml version="1.0" encoding="utf-8"?>
<!DOCTYPE hibernate-configuration SYSTEM
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<property name="hibernate.dialect">
org.hibernate.dialect.MySQLDialect
</property>
<property name="hibernate.connection.driver_class">
com.mysql.jdbc.Driver
</property>
<!-- Assume students is the database name -->
<property name="hibernate.connection.url">
jdbc:mysql://localhost/test
</property>
<property name="hibernate.connection.username">
root
</property>
<property name="hibernate.connection.password">
cohondob
</property>
</session-factory>
</hibernate-configuration>
Other annotations
Apart from the above configurations shown, there are other annotations as well. Let’s look at some of them here:
@Version
Control versioning or concurrency using @Version annotation.
@Entity
@Table(name = "company")
public class Company implements Serializable {
@Version
@Column(name = "version")
private Date version;
...
}
@OrderBy
Sort the data using @OrderBy annotation. In the example below, it will sort all contacts in a company by their first name in ascending order.
@OrderBy("firstName asc")
private Set contacts;
Conclusion
In this article, we largely studied Hibernate annotations and looked at various types of commonly used and other less important annotations.
In a later article, we will build upon these concepts to learn about Hibernate query language.
Recent Stories
Top DiscoverSDK Experts
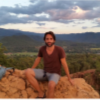
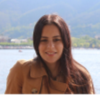
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment