Formatting Strings in Python 3
Text or string formatting is a daily task for Python programmers. Text formatting frees us from doing a lot of string concatenation with the help of the plus sign (+). String concatenation is not that bad when we have very small amounts of text and just a few variables to concatenate with other string literals, but concatenation can make our code a mess. It becomes hard to manage and hard to trace. String or text formatting frees us from this hassle.
In this guide, I will show you different ways to format strings in Python.
Before You Begin
This guide is for the beginners, but not for the absolute beginners. You should have some previous python knowledge to go along with this guide. Check the following list before you move forward.
- You must have basic knowledge of Python
- You must know different string operations
- You need to have Python 3 installed on your system
- You should have good familiarity working with the command line on your system
Creating a Working Environment
You should not just put your Python scripts here and there. You should use a certain directory of your file system and create a special directory for keeping your Python scripts. Inside your chosen directory create a file called format.py.
As code shown in this guide does not depend on any external library, we do not need to create a virtual environment.
You can write and edit code with the help of any plain text editor, code editor, or IDE. For Python I recommend PyCharm. A very minimal IDE is shipped with Python called IDLE; you can use that too.
The Old Way
Before going to the new way, let's remember what we had before and is still in existence. In the earlier version of Python, we used to format strings with the help of the % symbol. You need to put a string literal and then the "%" sign, and one or more values or objects that you want to send inside the string literal within their proper position with the appropriate data type. Inside the string literal you need to specify place holders with % symbol and a type specifier.
Let's have an example:
name = "John"
s = "Hey %s, how are you?" % name
print(s)
Running this you will get the following output.
Hey John, how are you?
The s after % told Python that we want the value converted to a string. So, let's try to put a floating point number there. The specifier for that will be f.
weight = 60
s = "I am just %f kg in weight" % weight
print(s)
You will see the following output:
I am just 60.000000 kg in weight
See? 60 became 60.000000. If you wanted an integer there you could put %d.
Let's try:
print("My weight is %d kg" % 55.55)
This outputs:
My weight is 55 kg
Notice that the decimal part has been discarded.
Now, what to do if we want to put multiple values? If we create multiple placeholders inside our string we can provide multiple values after % after the string. But to do that we need to enclose the values inside a tuple. Example below:
s = "My name is %s, I am %d year old and my weight is %f kg" % ("Kitty", 5, 25)
print(s)
This outputs:
My name is Kitty, I am 5 year old and my weight is 25.000000 kg
There are more things to do in the old way, but I’ll stop there and switch the discussion of the new way of string formatting.
The New Way
The old way is still valid and widely used—more than the new way even. Programmers from other languages including C are very familiar with the syntax of placeholder and the type specifier. Even experienced Python programmers have been using that for ages. Nowadays, it is recommended to use the new way of string formatting which uses the format() method on the string. But I won’t force you to take sides. Use whatever way you want.
In the new way of string formatting we format strings with the help of the format() method on strings. The placeholder for doing this is {}. You can leave that pair of curly braces empty and pass positional parameters to the format() method for filling that up. You can provide a number as an index inside those pair of curly braces and accordingly put positional arguments with format(). Until this, it’s all almost the same as we did in the old way. The added benefit and the way that I like most is naming those placeholders and providing values as keyword arguments to format(). In this way you can also specify types. Let's see everything one by one and replace our previous examples.
name = "John"
s = "Hey {}, how are you?".format(name)
print(s)
weight = 60
s = "I am just {0} kg in weight".format(weight)
print(s)
print("My weight is {} kg".format(55.55))
s = "My name is {name}, I am {age} year old and my weight is {weight} kg".format(name="Kitty", age=5, weight=25)
print(s)
This will output:
Hey John, how are you?
I am just 60 kg in weight
My weight is 55.55 kg
My name is Kitty, I am 5 year old and my weight is 25 kg
Wait, we have missed the types. How do we put types within the placeholders? We need an extra colon to do that and provide the type specifier. Example below:
name = "John"
s = "Hey {:s}, how are you?".format(name)
print(s)
weight = 60
s = "I am just {0:f} kg in weight".format(weight)
print(s)
s = "My name is {name:s}, I am {age:d} year old and my weight is {weight:f} kg".format(name="Kitty", age=5, weight=25)
print(s)
Outputs:
Hey John, how are you?
I am just 60.000000 kg in weight
My name is Kitty, I am 5 year old and my weight is 25.000000 kg
To know more about how the format specifier works, you need to read the official documentation on Format Specification Mini-Language in Python.
In our examples the floating point numbers are being printed up to six digits after the decimal point. To limit that we can put a dot and the number of digits we want after the decimal point before the type character. Example below:
s = "Give me 2 digits after decimal point: {0:.2f}".format(4.329)
print(s)
Outputs:
Give me 2 digits after decimal point: 4.33
Notice that the number is shown as desired and the number is also rounded.
Note: Unlike the old way if you want to convert floating numbers to decimals with the help of :d, an exception will be thrown.
Conclusion
To get the best out of string formatting in Python you need to know the Format Specification Mini-Language in detail. Read that from the official documentation. Also, some other features of string formatting are not shown here that I would like to discuss in detail in future lessons.
Recent Stories
Top DiscoverSDK Experts
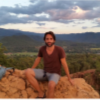
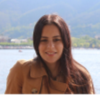
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment