ECMAScript 6 - Reflect
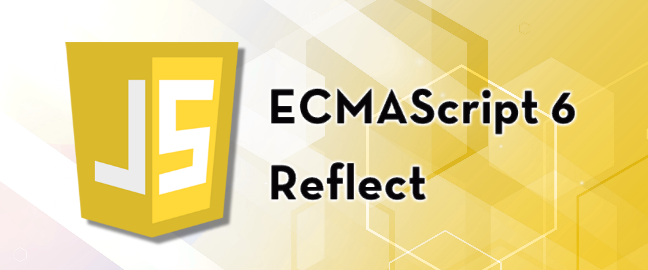
The reflect object is one of the most significant and important additions to ES6, and many don’t pay attention to it which is a real shame. Overall, we’re talking about a much better way to get information about an object.
I think I’ll start with an example, and then continue later with the chit-chat:
Object.getOwnPropertyDescriptor(1, 'foo')
getOwnPropertyDescriptor is a well known method through which we can get information about a particular attribute of an object. In this case, I passed a 1 inside the object. 1 is not really an object and I would expect to get an error. But in the case of object we get undefined. Despite this, if I use reflect, I’ll get a more normal error:
Reflect.getOwnPropertyDescriptor(1, 'foo')
One can see that the method name of Reflect is the same as that of Object. In general, the object becomes obsolete while Reflect takes its place as the main access provider for object operations—but not only. Here’s a nice example with apply:
var ages = [11, 33, 12, 54, 18, 96];
// Function.prototype style:
var youngest = Math.min.apply(Math, ages);
var oldest = Math.max.apply(Math, ages);
Here I’m finding the minimum and maximum with apply. But as mentioned, Reflect is supposed to contain everything about meta-programming—in other words operations carried on other software (like the apply function, for example). If I want to use Reflect, I can use apply in a very intuitive manner:
var ages = [11, 33, 12, 54, 18, 96];
// Reflect style:
var youngest = Reflect.apply(Math.min, Math, ages);
var oldest = Reflect.apply(Math.max, Math, ages);
Not so difficult, but indeed quite effective. The rationale behind Reflect is that it should concentrate all the operations that we are carrying out on a program (or part of one like apply, get, set, call, and the like) in one place, and theoretically prevent someone from changing your call, get, or other operation in prototype, and messing everything up for you.
Here’s another example of something a little more tangible: singleton. Before the era of Reflect, here’s how it looked:
class Greeting {
constructor(name) {
this.name = name;
}
greet() {
return `Hello ${name}`;
}
}
// ES5 style factory:
function greetingFactory(name) {
var instance = Object.create(Greeting.prototype);
Greeting.call(instance, name);
return instance;
}
We have here a class, that we learned about in the previous article, and a function with which I create the class. With ES5 I use object.create—despite this I can use Reflect.construct:
class Greeting {
constructor(name) {
this.name = name;
}
greet() {
return `Hello ${name}`;
}
}
// ES6 style factory
function greetingFactory(name) {
return Reflect.construct(Greeting, [name], Greeting);
}
One of the great things about Reflect is that you can delete an attribute, something that until now had to be done using the keyword delete:
//ES5
var myObj = { foo: 'bar' };
delete myObj.foo;
assert(myObj.hasOwnProperty('foo') === false);
//ES6
myObj = { foo: 'bar' };
Reflect.deleteProperty(myObj, 'foo');
assert(myObj.hasOwnProperty('foo') === false);
Let’s say, it’s not something that’s really gonna knock your socks off, but finally all the meta-programming operations (all those where your input is an object/function) are in one place—Reflect. A list of all the available attributes for Reflect can be found in MDN.
Reflect is supposed to get along well with proxy, which we covered last time. Actually, Reflect’s output is quite similar to the output of trap which matches proxy. If you use proxy, we can assume that you’ll need to return Reflect since it will be easier. Here’s an example using proxy and reflect:
var handler = {
get () {
return Reflect.get(...arguments)
}
}
var target = { a: '111' }
var proxy = new Proxy(target, handler);
console.log(proxy.a); //111
In this instance the trap is on get and I’m returning what I receive from Reflect.get, which is the same as trap.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
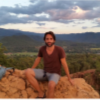
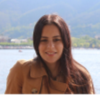
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
0 Comments
Your Comment