ECMAScript 6 - Proxy
Another interesting feature of ECMAScript 6 is proxy. It’s a feature that’s largely ignored by many ES6 guides because support for it was quite limited in the past, even with TypeScript or Babel. This is also due to it begin tied to the somewhat opaque concept of Metaprogramming—programs the use other programs as their input.
But that’s enough fear mongering for now. Proxy in JavaScript is not really that complex. Long story short, we’re talking about a way to wrap object A in object B, and in doing so, control or monitor things that use it. In other words, we can use proxy to completely control a JavaScript object, including its methods and attributes.
Proxy has three terms related to it that we need to remember:
- Target - the goal or aim. This is the object that we are wrapping.
- Handler - the object that contains the operations that we want to catch in the handler. For example: get, set, and apply.
- Trap - this is the operation. We define get trap if we want to catch get for example.
OK, that’s enough explaining. Too much theory with no code is bad for our health. So here’s a little Hello World for you:
var data = {
name: 'Billy Bob',
age: 15
};
var handler = {
get(target, key, proxy) { //This is the trap
const today = new Date();
console.log(`GET request made for ${key} at ${today}.`);
}
}
var data = new Proxy(data, handler);
// This will execute our handler, log the request, and set the value of the `name` variable.
var name = data.name;
What’s going on here? First I define an object and call it data. This is the object I’m going to wrap. Next I create a handler that has a trap. The handler is a simple object the contains the traps. In this case I have just one which is the get. In other words, everytime something calls to any attribute in the original object, the get function in the handler is invoked.
The actual binding of the proxy is carried out in this line:
var data = new Proxy(data, handler);
In the last line I’m executing just some call to one of the attributes. If you run the browser, you’ll see that the get is invoked.
Let’s run another example with a different trap. For example, a set that is invoked every time something changes the object’s attributes.
var object = {};
var handler = {
set(target, key, value, receiver) { //This is the trap
console.log(`SET request made for ${key}, value ${value}`);
}
}
var object = new Proxy(object, handler);
object.someProp = 'Moshe'; //"SET request made for someProp, value Moshe"
Here I’m using a different trap and catching the set. That is to say, anytime something tries to change an attribute, any attribute, my proxy will know.
There are quite a few traps. We’ve seen now get and set, each having different arguments. There is also construct for example, which is invoked every time we have new. For instance:
var myFunc = function() {};
var handler = {
construct(target, argumentsList, newTarget) { //This is the trap
console.log('activated!')
console.log(argumentsList)
}
}
var myFunc = new Proxy(myFunc, handler);
var whatever = new myFunc('arg1', 'arg2'); //"activated!", ["arg1", "arg2"]
So here’s the question. What exactly do we do with proxy? Ah ha ha! Well, the first usage is for stealthy logging and debugging that won’t bother anything. If you have something you want to log, then it’s really easy with proxy. All kinds of testing and validations are also really easy with proxy.
What you shouldn’t try do with proxy is to make a class private. Even though proxy can simulate a kind of encapsulation in JavaScript, that’s not what it was built for and I would advise against it.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
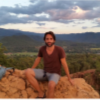
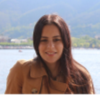
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment