Conditional Statements in Ruby
Programming was created to make free us from repetitive tasks and performing different tasks differently depending on various conditions. We code every program differently to do different kinds of things differently. We let our programs make decisions on our behalf.
Ruby is a very expressive language and expressing your conditional logic is easier in this language than most of the others. Instead of creepy code like many other languages, you can express conditions in a more English like manner.
Before You Begin
This is an introductory article and you do not need to know much about Ruby programming to move forward with this one. But make sure that you have Ruby knowledge of working with variables, gets, puts, strings, numbers, booleans, and also optionally arrays and hashes.
Also, make sure that you are comfortable working with the command line in your system. To edit Ruby code you need a plain text editor or a code editor. It's also OK to use IDEs but it’s not recommended for beginner articles. And if everything is alright, make sure that you have Ruby installed on your system.
Preparing Environment
Preparing and keeping consistent with your coding environment is a good habit. There is not much to do in the introductory level. Choose or create a directory of your choice where you want to put your Ruby files. Create a file named condition.rb in this directory. Open this file with your preferred editor. Point your command line's CWD to this directory. Run the file using ruby condition.rb on the command line.
If This is True Then Do That
The first thing we will be introduced is with the if statement in Ruby. If the expression following it evaluates to true, the following block of code will be executed. Anything that has a boolean equivalent of true evaluates to true, otherwise it is false. Let's have an example:
if 1 > 0 then
puts "I'm on the side of truth"
end
Outputs:
I'm on the side of truth
Things to Do on The Failure of if
In the previous example we just commanded what to do when certain condition are true. But we did not say anything if it were to evaluated to false. We can do that with the else block. Else blocks are only executed when the expression that follows if evaluates to false. Let's have another example:
if 1 > 1 then
puts "I'm on the side of truth"
else
puts "My dear friend is else"
end
Outputs:
My dear friend is else
1 is not greater than 1 and thus our code fell back to the else side.
Multiple Conditions
In the previous sections we saw a demonstration where one thing or other happenes. But, we did not think about other possibilities. In real life scenarios, we have many options and more than one way to go. We need better branching for that. To check more than one condition, we need to use an elsif block. It means else if. So, if the if condition does not evaluate to true, we can check one or more conditional expressions before giving up and falling back to else. Let's have a different example:
url = "ftp://example.com/file.ext"
if url.start_with? "http://"
puts "This is an http url"
elsif url.start_with? "https://"
puts "This is a secure version of http"
elsif url.start_with? "ftp://"
puts "Yeah, this is file transfer protocol"
else
puts "I have no idea what type of URL is this - maybe this is an invalid url"
end
Outputs:
Yeah, this is file transfer protocol
Note:
You should have noticed by now that the then part is optional.
Unless is the 'If Not'
As Ruby has a good reputation of being more like English, it has an unless statement along with if. Unless in Ruby means if not. So, if the conditional expression is false the enclosing block is executed. I am not writing an example for this one and leaving the exercise on you. Hurry up! Do not be late.
Case Switching
Sometimes if..elsif..else can get really messy. We need a cleaner way of condition checking in that case. We need to switch case to case to check the condition and execute the specified block.
no = 5
case no
when 0 .. 5
puts "0 to 5"
when 5 .. 10
puts "5 to 10"
when 10 .. 15
puts "10 to 15"
else
puts "no match"
end
Outputs:
0 to 5
Unlike other languages, we did not use switch statements in Ruby. We used case and when statements.
Note:
Case switching is limited in its functionality. Do not try to use it everywhere for its simplicity. Simplicity can be deceptive and your program may grow to be more complicated. Whenever you see that conditions are getting more complex than just simple values or ranges, move away from case switching and fall back to if..elsif..else.
Conditional Modifiers
Conditional modifiers make programming more human friendly. Without writing if, unless first, we can go for a cleaner solution. We can perform the task on the left if some condition evaluates to true for if and false for unless. Let's illustrate with examples.
i = 50
print "I am equal or more than 50" if i >= 50
Outputs:
I am equal or more than 50
You can also use the unless modifier. I am leaving this exercise on you to do something when the unless modifier is used.
Remark:
Conditional modifiers are used often for assigning or reassigning some values depending on some conditions. It is also frequently used to skip or execute single statements depending on conditions. Use modifiers with care. Many people, being are deceived by their simplicity, overuse them and make pretty buggy code.
Conclusion
This being a very simple guide on conditionals in Ruby I did not go deeper into the rabbit hole. This is more like making foundations for you to work on complex things in future articles, guides and tutorials.
Recent Stories
Top DiscoverSDK Experts
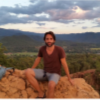
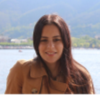
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment