Bundled Notifications in Android
In this tutorial, we will have a look at the Notifications API in Android and more importantly, latest features of the API which were added in Marshmallow (6.0) and Nougat (7.0).
Notifications
A notification is simply a message which is displayed to the user outside of the application's normal User Interface. When you tell the Android system to create a notification, initially it appears as an icon in the notification area (also, the status bar) like shown:
To see the details, the user can open the notification drawer. Both the notification area and the notification drawer are system-controlled areas that the user can access any time.
Notifications play very important role in providing a perfect (or worst) user experience in an application.
Creating a notification
While creating a Notification, we need to define some properties of which, some are mandatory to be provided and others are optional.
A Notification object must contain the following properties:
- A small icon, set by setSmallIcon() method.
- A title, set by setContentTitle() method.
- Detail text, set by setContentText() method.
Function of above methods are self-explanatory by the method name. Some optional properties you can set to provide a custom behavior are:
- addExtras(...) : Merge additional metadata into this notification.
- setAutoCancel(...) : This flag make sure that the notification is automatically canceled when the user clicks it in the notification area.
- setVibrate(...) : Set the vibration pattern to use.
To create a notification, simple follow the following process:
NotificationCompat.Builder mBuilder =
new NotificationCompat.Builder(context)
.setSmallIcon(R.drawable.notification_icon)
.setContentTitle("DiscoverSDK")
.setContentText("Content notification text for DiscoverSDK");
Intent resultIntent = new Intent(context, RootActivity.class);
PendingIntent resultPendingIntent = PendingIntent.getActivity(this, 0, resultIntent, PendingIntent.FLAG_ONE_SHOT);
mBuilder.setContentIntent(resultPendingIntent);
// Gets an instance of the NotificationManager service
NotificationManager mNotifyMgr =
(NotificationManager) context.getSystemService(Context.NOTIFICATION_SERVICE);
// Builds the notification and issues it.
mNotifyMgr.notify(mNotificationId, mBuilder.build());
Bundled Notifications
This article is not mainly about creating simple notifications. Rather, we will extend that type of notifications.
One notification trying to do everything, OR 10 notifications trying to take over the whole notification chain? OR why not the best of both worlds with BUNDLED NOTIFICATIONS ! By bundling notifications into a group, user will be able to act on the entire group at once or expand the group and act on the individual notifications in the group.
Step 1 : Make awesome Individual notifications
Now, before moving to actually making bundled notifications, you should first work on making individual and awesome notifications. Here, we’ll assume you have already done that and we will extend it further.
Step 2 : Bundle your notifications
Group similar notifications like:
- All chat messages
- All email for a particular account
NotificationCompat.Builder mBuilder = . . .
mBuilder.setGroup(GROUP_KEY_EMAIL);
// Optionally
// mBuilder.setSortKey(sortString);
As shown above, bundling notifications together is done by calling setGroup(...) for each notification you create. By default, notifications will be ordered by when they’re posted. But you can override it with setSortKey(...) and the system will sort with that key instead.
Step 3 : Build a summary notification
This is one additional part to bundled notifications, though : a summary notification. On Marshmallow & lower phones and tablets that don’t directly support bundled notifications, only the summary notifications will be displayed. So make sure it’s also a great notification !
The inbox style is a great candidate for these summary notifications to give users a quick overview of all the notifications they have. Make sure to call setGroup with same key and then call setGroupSummary true to denote that notification as your summary notification. Let’s write some code to describe it better:
mBuilder.setStyle(new NotificationCompat.InboxStyle()
.addLine("Alex : Hello")
.addLine("John : Hola")
.setBigContentTitle("2 new messages")
.setSummaryText("awesome@discoversdk.com"))
.setGroup(GROUP_KEY_EMAIL)
.setGroupSummary(true);
>>> Note, you’ll still want to build a summary notification on devices that support bundled notifications, as that’s used by the system to extract information from the header when the group is collapsed.
Direct reply from Notifications
This is another cool feature added to notifications in latest Android 7.0.
The RemoteInput API (just like in Android Wear) itself contains information like the key which will be used to later retrieve the input and the hint text which is displayed before the user starts typing.
// Where should direct replies be put in the intent bundle
private static final String KEY_TEXT_RESPOND = "key_text_respond";
// Create the RemoteInput specifying above key
String respondLabel = getString(R.string.respond_label);
RemoteInput remoteInput = new RemoteInput.Builder(KEY_TEXT_RESPOND)
.setLabel(respondLabel)
.build();
Once we have constructed the RemoteInput object, it can be attached to the Action via the addRemoteInput() method.
// Add to your action, enabling Direct Reply
NotificationCompat.Action mAction =
new NotificationCompat.Action.Builder(R.drawable.respond, respondLabel, pendingIntent)
.addRemoteInput(remoteInput)
.setAllowGeneratedReplies(true)
.build();
This is worth noticing that the pendingIntent being passed into the Action should be an Activity on Marshmallow and lower devices that don’t support Direct Reply (in that case, dismiss the lock screen, start an Activity, focus the input field to have the user type their response) and should be a Service or BroadcastReceiver on Android N devices so as to process the text input in the background even from the lock screen.
After you’ve processed the text, you must update the notification by calling notify() with the same id and tag (if used). This is the trigger which hides the Direct Reply UI and should be used as a technique to confirm to the user that their reply was received and processed correctly.
Too much work? It’s worth it !
Now, if you’re thinking that it is a lot of work to add group key, group summary, sounds like a lot of work ! There are 2 additional reasons you should do these type of notifications:
- There’s a lot of users out there with devices which support bundled notifications. Every Android wear user ! By using this API, you’re making a very good experience for all of them.
- Secondly, API 24 and higher devices are going to bundle your notifications for you if you post 4 or more notifications without any grouping and you will probably have a better idea what bundles and groups make sense for your own apps.
>>> Note : API 24 and higher devices will automatically bundle your notifications.
So, if you’re posting multiple notifications, use bundled notifications to help you build better devices and for a wider range of devices.
Conclusion
The notification system is one of the strongest points of Android. You have a lot of control, and you’re getting even more with latest Android release.
In this article, we studied about the Notifications API in Android and more importantly, the bundled notifications and how you can create an awesome and more personalised experience for your users across all devices including Android Wear.
Recent Stories
Top DiscoverSDK Experts
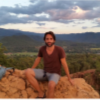
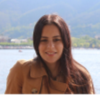
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment