All About Symmetric Encryption
This article is part of a series that talks about the basics of cryptography. When I say basics, I mean just that—basics. These articles are intended to provide you with an understanding of the concepts.
In this article, we’ll talk about symmetric encryption. Last time we looked at obfuscation and specifically obfuscation of a program. But obfuscation can be for anything: texts, fields, secrets, whatever. At the end of the day, obfuscation just takes the original text and jumbles it up. But encryption is taking the original text and jumbling it up in a way that’s impossible to crack without the encryption key.
Encryption has probably been around since the dawn of civilization. For instance, there’s the famous ancient encryption know as Atbash used with the Hebrew alphabet. In it, the first letter is switched with the last letter, the second with the second to last, and so on. It goes like this:
Plain |
A |
B |
C |
D |
E |
F |
G |
H |
I |
J |
K |
L |
M |
N |
O |
P |
Q |
R |
S |
T |
U |
V |
W |
X |
Y |
Z |
Cipher |
Z |
Y |
X |
W |
V |
U |
T |
S |
R |
Q |
P |
O |
N |
M |
L |
K |
J |
I |
H |
G |
F |
E |
D |
C |
B |
A |
So, for instance, the word ‘nice’ would be ‘mrxv.’ In this case, the text I’m encrypting is ‘nice,’ the key is the Atbash, and the encrypted text is ‘mrxv.’ OK, so let’s say person A wants to encrypt the text ‘nice.’ They do the encryption using the table above and get ‘mrxv’ which they send to person B. Person B then uses the key (again, the table above) and converts the text back to ‘nice.’ If someone intercepts the text between them, they’ll only see the ‘mrxv’ and won’t be able to understand anything.
This is, of course, a pretty naive approach to security. The person intercepting the message will probably not simply cry in the corner and give up. They’ll likely try to crack the code and figure out that the encryption is pretty easy. This duel between encryption creators and encryption crackers is as old as encryption itself—along with Atbash there are many other old encryption systems. But with all these systems there is a text we want to encrypt, a key to use for the encryption, and the encrypted text that we open with the key. This is why it’s called symmetric encryption—the same key is used to encrypt and to open the text.
It goes without saying that in the real world we wouldn’t use Atbash encryption or any of the other simple, old systems. They are very easily cracked by brute force attacks, which is the main weakness of symmetric encryption. It’s the type of encryption where the stronger the computing power, the easier it is to crack. But, and it’s a big but, today’s symmetric encryptions take a long time to crack via a brute force attack, depending on the size of the key; the bigger the key, the longer it takes.
Part of the weakness of symmetric encryption lies in the fact that we use the same key for the encrypting and decoding. This makes cracking the code much easier. Take for example the Vigenère cipher which was considered pretty sophisticated for its time. Yet it was broken rather easily by examining the frequency of occurrence of the letters. For example, we know the letter E is the most frequently used letter in English which allows us to guess what the corresponding letter is in the encrypted text. This is why we have Initialization vectors in modern encryption which put in some randomization to the encryption.
Today’s standard symmetric encryption is known as Advanced Encryption Standard (AES), but I won’t get into a full explanation of how it works. It’s free and its license allows for anyone to use it even for commercial purposes. This encryption isn’t based on text but rather ‘blocks’ and its strength lies in the length of its keys. The key is basically repetitions of the encryption algorithm. The more repetitions there are, the longer it takes to crack but the more processing power is required. If we’re using 256 bit AES it would take a really long time to crack the encryption. Using a weaker key will be faster for your application but will be easier to crack. So which to use? That depends on the nature and details of your app.
OK, enough info and theory. How does all this look in practice—in actual code? You can find AES libraries in pretty much any language you can think of. We’ll look at an example in Node.js. Important note: this is just an example for demonstrating how AES works. Do not use this code in a production environment. If you need to build things from the ground up, you’ll need more than what’s in this article.
First, we choose the algorithm (in this case the strongest AES) and the length of its strength. Afterward, we pick our cipher mode: ECB, CBC, CTR, OFB, CFB. For hard drives we use XEX, XTS, LRW. So which to choose? Good question. The answer is something we can’t really say here—like with other issues it depends on the project. For our example’s sake, we’ll use the GCM mode, which is said to be good for general content.
After that, we’ll create our key. This can be any text like ‘Password used to generate key.’ We’ll use this same happy occasion to make the Initialization Vector. And a map? A map is just the simple usage of the crypto library that’s built into Node.
const crypto = require('crypto');
// choose Algorithm, there are several flavours
const algorithm = 'aes-256-gcm';
const password = 'Password used to generate key';
// 32 bytes (256 bits).
const key = crypto.scryptSync(password, 'SomeSalt', 32);
// Create Initialization vector
const iv = crypto.randomBytes(32); // Initialization vector.
function encrypt(text){
const cipher = crypto.createCipheriv(algorithm, key, iv);
let crypted = cipher.update(text,'utf8','hex');
crypted += cipher.final('hex');
return crypted;
}
function decrypt(text){
let decipher = crypto.createDecipheriv(algorithm, key, iv);
let dec = decipher.update(text,'hex','utf8');
return dec;
}
const encryptedText = encrypt('This is super secret text');
console.log(encryptedText)
// This is super secret text
console.log(decrypt(encryptedText));
If you check this for yourself, you’ll see that each time the encrypted text is different but the deciphering is the same. Why? This is because of the IV that also changes. If we use one set IV, we’ll get the same deciphered text.
➜ crypto node index.js
01afae88c98c0807faaa55
hello world
➜ crypto node index.js
49f8076a127f46240cc38953221d625547561aec448f988367
This is super secret text
➜ crypto node index.js
aa2c262cbcd270b918057a01b511800090e916a575af473c93
This is super secret text
➜ crypto node index.js
c50965bcfa0de5bd1c1e32d627cff8bfc78337d859fedf2e24
This is super secret text
So, to use a random IV or not? Or should we use 256 or 128 encryption? And which mode? Wow! These are all great and important questions which I could answer if I was a cryptographer. But I’m not. And that’s exactly why I hire a cryptographer if I to need build something like this from the ground up. But the examples above are great if you want to understand the basics of symmetric encryption.
To sum up, symmetric encryption is quick, fairly resistant to hacking (but not uncrackable) and to decipher it we need one key and perhaps an initialization vector. Its algorithm is dynamic and can be used in any language. In Node.js, there are countless libraries that allow us to implement symmetrically encrypted communications. It’s even used as part of HTTPS.
But, AES isn’t good enough for perfect encryption. In the next article in this series, we’ll talk about asymmetric encryption—one of the most important developments in the history of cryptography. See you then!
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
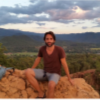
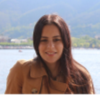
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment