Flow Of Control Examples
While Example
#include <conio.h>
#include <stdio.h>
void main()
{
clrscr();
int n1,n2 ;
int no_of_games,no_of_points,no_of_goals ;
float average;
no_of_games=no_of_points=no_of_goals=0;
while(1)
{
printf("Please enter first number==>");
scanf("%d",&n1);
printf("Please enter second number==>");
scanf("%d",&n2);
if (n1==-1 && n2==-1)
break;
no_of_games++;
no_of_goals +=n1;
if (n1 > n2)
no_of_points +=3;
else
no_of_points++;
}
printf("No of points = %d\n",no_of_points);
printf("no_of_games = %d\n",no_of_games);
average=float(no_of_goals)/float(no_of_games);
printf("Average no of goals per play = %2.1f",average);
}
The program gets long integer and check:
if num. of digits is even tells how many odd digits are
if num. of digits is odd tells how many even digits are
#include<stdio.h>
int main(void)
{
int even=0,odd=0,i;
long number;
printf(“Please enter the number\n”);
scanf(“%ld”,&number);
do
{
if(!(number%2))
++even;
else
++odd;
number/=10;
}while(number);
if(!((odd+even)%2))
for(i=1;i<=odd;++i)
putchar('*');
else
for(i=1;i<=even;++i)
putchar('?');
return 0;
}
A fine example of using the case condition:
This program gets an arithmetic problem containing the 4th operation + – * /
gives the result after =
when the result is zero or the user types only '=': the program stops.
wrong input causes an alert
#include <stdio.h>
#include <stdlib.h>
void main(void)
{
char c;
int last_op;
int error,was_dot;
double next,result,power;
do{
result=next=was_dot=0;
power=1; last_op='+';
do{
error=0;
switch (c=getch())
{
/*digit*/
case '0':
case '1':
case '2':
case '3':
case '4':
case '5':
case '6':
case '7':
case '8':
case '9': if(was_dot)
next+=(power/=10)*(c-'0');
else
next=next*10+(c-'0');
break;
/*dot*/
case '.': if(was_dot)
error=1;
else
was_dot=1;
break;
/*delim*/
case '+':
case '-':
case '*':
case '/':
case '=': switch (last_op)
{
case '+':
result+=next;
break;
case '-':
result-=next;
break;
case '*':
result*=next;
break;
case '/':
if(next)
result/=next;
else
error=1;
break;
}
if(!error)
{
next=was_dot=0;
power=1; last_op=c;
}
break;
default:
error=1;
}
if(error)
printf("\a");
else
putch(c);
}while (error || (c!='='));
printf("%g\n",result);
}while(result);
printf("Have a nice day.\n");
}
find max and min for a sequence of numbers
#include <limits.h>
#include <conio.h>
#include <stdio.h>
#define TRUE 1
#define EXIT -9999
void main()
{
clrscr();
int n,max,min;
max=INT_MIN; min=INT_MAX;
printf("Enter integer numbers in the range:" );
printf("%d %d",INT_MIN,INT_MAX);
printf("\nto exit enter ==> %d\n", EXIT);
do
{
printf("Enter next ==>");
scanf("%d",&n);
if( n==EXIT )
break;
if ( n > max )
max=n;
if ( n < min )
min= n;
}
while(TRUE);
printf("max=%d min=%d",max,min);
}
Fibonacci Numbers
#include <conio.h>
#include <stdio.h>
void main()
{
int i;
int n;
int an,an_1,an_2;
an=1;
an_1=0;
an_2=0;
clrscr();
printf("Enter an integer > 2===>");
scanf("%d",&n);
for (i=1;an<n;i++)
{
printf("a(%d) = %d\n",i,an);
an_2 = an_1;
an_1 = an;
an=an_1+an_2;
}
}
Another Example
#include <conio.h>
#include <stdio.h>
void main()
{
int n;
int BestCar;
int BestResult=-1;
int i,age,last_position,result;
char model;
clrscr();
printf("Enter no of cars in the race ==>");
scanf("%d",&n);
for(i=1; i<=n;i++)
{
printf("Enter (%d)'s car :age ,model,last position ->",i);
scanf("%d %c %d",&age,&model,&last_position);
if (age >10)
{ printf("illegal age-- try a number between 0 and 10\n");
i--;
continue;
}
if (model!='A' && model != 'B' && model != 'C')
{
printf("illegal model try A or B or C\n");
i--;
continue;
}
result=(10-age) +(3- (model - '@'))+(10-last_position);
/* notice the ascii letter before 'A' is '@' */
if (result > BestResult)
{
BestResult= result;
BestCar=i;
}
if (last_position<1 || last_position >10)
{
printf("illegal last position try 1 to 10\n");
i--;
continue;
}
}
printf("The best car is %d with the score of: %d",BestCar,BestResult);
}
Multiplication Table
#include <conio.h>
#include <stdio.h>
void main()
{
int i,j,k;
clrscr() ;
printf(" multiplication table\n \332");
for ( k=1; k<=10 ;k++)printf("\304\304\304\302");
printf("\n \263");
for(k=1;k<=10;k++) printf("%3d\263",k);
printf("\n ");
for ( k=1; k<=11 ;k++)printf("\304\304\304\305");
for (i=1; i<=10; i++ )
{
printf("\n%3d \263",i );
for (j=1; j<=10 ; j++)
printf("%3d\263",i*j);
printf("\n ");
for ( k=1; k<=11 ;k++)printf("\304\304\304\305");
}
}
Another Example:
#include <conio.h>
#include <stdio.h>
void main()
{
int i,j;
clrscr() ;
printf(" multiplication table\n");
printf
("----------------------------------------------\n");
printf
(" | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10| \n");
printf
("----------------------------------------------\n");
for (i=1; i<=10; i++ )
{
printf("%3d |",i );
for (j=1; j<=10 ; j++)
printf("%3d|",i*j);
printf("\n");
}
}
Yet Another Example
#include <conio.h>
#include <stdio.h>
#define HORIZONTAL_LINE 196
#define VERTICAL_LINE 179
#define PLUS 197
#define LEFT_UPPER_CORNER 218
#define RIGHT_UPPER_CORNER 191
#define LEFT_LOWER_CORNER 192
#define RIGHT_LOWER_CORNER 217
#define T 194
#define RIGHT_T 180
#define LEFT_T 195
#define OPPOSITE_T 193
void DrawLine(int no_of_columns,
int left_char,int middle_char,int right_char);
void main()
{
int no_of_columns;
int i,j,k;
clrscr() ;
printf("Enter no of columns( 3-11) ==>");
scanf("%d",&no_of_columns);
clrscr();
printf(" multiplication table\n\n ");
/*----------------------------first 3 lines ---------------*/
DrawLine (no_of_columns,LEFT_UPPER_CORNER,T,RIGHT_UPPER_CORNER);
printf("\n %c %c",VERTICAL_LINE,VERTICAL_LINE);
for(k=1;k<=no_of_columns;k++) printf("%3d%c",k,VERTICAL_LINE);
printf("\n ");
DrawLine (no_of_columns,LEFT_T,PLUS,RIGHT_T);
/*----------------------------table contents ----------------*/
for (i=1; i<=no_of_columns; i++ )
{
printf("\n %c%3d%c",VERTICAL_LINE,i,VERTICAL_LINE );
for (j=1; j<=no_of_columns ; j++)
printf("%3d%c",i*j,VERTICAL_LINE);
printf("\n ");
DrawLine (no_of_columns,LEFT_T,PLUS,RIGHT_T);
}
/*-------------------------correct last line----------------*/
printf("\r ");
DrawLine
(no_of_columns,LEFT_LOWER_CORNER,OPPOSITE_T,RIGHT_LOWER_CORNER);
}
void DrawLine(int no_of_columns,
int left_char,int middle_char,int right_char)
{
int k;
printf("%c",left_char);
for(k=1;k<= no_of_columns;k++)
printf("%c%c%c%c",
HORIZONTAL_LINE,HORIZONTAL_LINE,HORIZONTAL_LINE,middle_char);
printf("%c%c%c%c",
HORIZONTAL_LINE,HORIZONTAL_LINE,HORIZONTAL_LINE,right_char);
}
Recent Stories
Top DiscoverSDK Experts
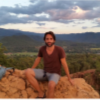
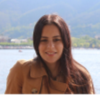
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment