Condition Variables as Events
#include <stdio.h>
#include <stdlib.h>
typedef struct
{
pthread_mutex_t mx;
pthread_cond_t cv;
int flag;
}event;
void wait_for_event(event *ev)
{
pthread_mutex_lock(&ev->mx);
while(flag==0)
{
pthread_cond_wait(&ev->cv,&ev->mx);
}
ev->flag = 0;
pthread_mutex_unlock(&ev->mx);
}
void send_event(event *ev)
{
pthread_mutex_lock(&ev->mx);
ev->flag=1;
pthread_cond_signal(&ev->cv);
//pthread_cond_broadcast(&ev->cv);
pthread_mutex_unlock(&ev->mx);
}
void init_event(event *ev)
{
ev->flag = 0;
pthread_mutex_init(&ev->mx,NULL);
}
void *thr1(void *p)
{
event *e1 = (event *)p;
//
//
wait_for_event(&e1);
//
}
void *thr2(void *p)
{
event *e1 = (event *)p;
//
send_event(&e1);
//
}
int main(void) {
event e1;
pthread_t t1,t2;
init_event(&e1);
pthread_create(&t1,NULL,thr1,&e1);
pthread_create(&t2,NULL,thr2,&e1);
return EXIT_SUCCESS;
}
Recent Stories
Top DiscoverSDK Experts
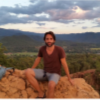
Mendy Bennett
Experienced with Ad network & Ad servers.
Mobile | Ad Networks and 1 more
View Profile
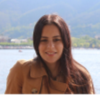
Karen Fitzgerald
7 years in Cross-Platform development.
Mobile | Cross Platform Frameworks
View Profile
X
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}Now comparing:
{{product.ProductName | createSubstring:25}} X
{{CommentsModel.TotalCount}} Comments
Your Comment