Working With Strings In C
Bytes are for machines and strings are for humans. Coding a real world application without strings is impossible. Internally a string is nothing but an array of bytes, but the abstracted representation provides us with a way to act and write like a human, instead of a machine.
In C there is no type called string. In C, strings are null terminated character array. That means, any array of character that has a null byte at the end of it is considered as a string. If a character array has 100 cells and it has a null byte (represented as '\0' as a character) at 50th cell then character from index 0 to index 50 (excluding 50) is collectively a string.
Though there is no string type, we can write strings literally by putting them inside double quotation marks, instead of writing each character separately in a character array.
Getting Started With Code
To start writing and practicing the code used in this article, you have to have a C compiler installed on your system. You can use any plain text editor, C supported code editor or IDE for writing and editing code. I am going to use GCC as the compiler and CodeBlocks as the IDE.
I am going to create a file called str_test.c for writing our code. You are free to name it whatever you like. Our initial bare bone code looks like the following:
#include <stdio.h>
int main(){
return 0;
}
Hit compile and run to check if everything is alright.
To perform various string operations, we need to include the 'string.h' library.
#include <stdio.h>
#include <string.h>
int main(){
return 0;
}
Declaring And Creating Strings In C
Strings in C can be created literally or dynamically. The type of strings that we write manually or by hand in the source code are the literal string. To create a literal string, surround characters inside two double quotation marks in one line.
"I am a string"
There is no string data type in C. Then how do we declare a string as string? As I mentioned before that a string in C is an array of characters. So, we declare the string variable as an array of characters.
char str[] = "I am a string";
So, our complete code looks like this:
#include <stdio.h>
#include <string.h>
int main(){
char str[] = "I am a string";
printf("%s", str);
return 0;
}
Hit compile and run to see if everything is alright. You will see "I am a string" printed on the screen.
Note: An array variable is a pointer to the first element of the the array in reality. So, we can declare a string as a pointer to character.
char * str = "I am a string";
So, the complete code looks like the following:
#include <stdio.h>
#include <string.h>
int main(){
char * str = "I am a string";
printf("%s", str);
return 0;
}
Hit compile and run to see output as before.
Remember: A string is an array of characters, so we can create a string like below:
char str[13] = {'H', 'e', 'L', 'l', 'O', ' ', 'W', 'o', 'R', 'l', 'D', '!', '\0'};
Notice, that there is a null character at the end of the array.
So, our complete code becomes:
#include <stdio.h>
#include <string.h>
int main(){
char str[13] = {'H', 'e', 'L', 'l', 'O', ' ', 'W', 'o', 'R', 'l', 'D', '!', '\0'};
printf("%s", str);
return 0;
}
Outputs:
HeLlO WoRlD!
Creating string in other ways: Creating a string literally is not the only way to create strings in C. There are a lot of functions in standard and third party libraries that return a string as a result. You will learn about many of them in your journey to the land of C. You will even see some of them in this article.
String Length
The length of a string is the number of characters a string contains. But remember that this count does not include the null character at the end of the string. To get the length of a string you have to use the library function strlen().
#include <stdio.h>
#include <string.h>
int main(){
char * str = "Twinkle, twinkle, little star, How I wonder what you are!";
size_t len = strlen(str);
printf("The string contains %d number of characters", len);
return 0;
}
Outputs:
The string contains 57 number of characters
Note: It is alright if you use int in place of size_t.
Finding A Substring
It is a common task in programming to check whether a string contains another string (we call the second string a substring). Use the strstr() function to check it. This function accepts the string as the first argument and the substring as the second argument. It returns the pointer to the character of first occurrence inside the string.
#include <stdio.h>
#include <string.h>
int main(){
char * str = "Twinkle, twinkle, little star, How I wonder what you are!";
char * substr = "star";
char * res = strstr(str, substr);
if (res == NULL){
printf("The sub-string was NOT found");
}else{
printf("The sub-string was found");
}
return 0;
}
Outputs:
The sub-string was found
Note: strstr() returns NULL if the sub-string is not found.
Let's try a second example when the sub-string is not present within the string.
#include <stdio.h>
#include <string.h>
int main(){
char * str = "Now you see me, now you don't!";
char * substr = "star";
char * res = strstr(str, substr);
if (res == NULL){
printf("The sub-string was NOT found");
}else{
printf("The sub-string was found");
}
return 0;
}
Output:
The sub-string was NOT found
Remember: NULL represents the null pointer.
Comparing String Equality
Often we need to compare if two strings are equal or not. We use strcmp() to do this. It accepts two parameters as the two strings to be compared and returns an integer. If it returns 0 the the string are equal.
#include <stdio.h>
#include <string.h>
int main(){
char * str1 = "Star";
char * str2 = "Star";
char * res = strcmp(str1, str2);
if (res == 0){
printf("the strings are equal");
}else{
printf("the strings are not equal");
}
return 0;
}
Outputs:
the strings are equal
Note: It returns a negative or positive number when the strings are not equal. But in real life programming it would be rare that you need to see if a string is greater than or less than something. So, I am skipping that discussion here. If you need to compare length you can use strlen() function.
Adding Strings
In programming we call it concatenation instead of adding. So, if you want to concatenate two strings, you have to use the strcat() and strcpy() functions. strcpy() copies one string to another string and strcat() adds a string to the end of another one. You can do it alone with strcat() if you want. But I am showing here a combination of the two so that you can practice them both.
char str1[5];
char str2[10];
strcpy(str1, "Str 1");
strcpy(str2, "Str 2");
strcat(str2, str1);
printf("Final string : %s", str2);
So, our final program looks like:
#include <stdio.h>
#include <string.h>
int main(){
char str1[5];
char str2[10];
strcpy(str1, "Str 1");
strcpy(str2, "Str 2");
strcat(str2, str1);
printf("Final string : %s", str2);
return 0;
}
Outputs:
Final string : Str 2Str 1
And More
There are few other functions for working with strings in C. I have shown you the most important ones. It is not possible to explain all the functions in a single article. In some future articles I will discuss more advanced topics of string handling and also discuss a few other functions.
Conclusion
Life is impossible for a programmer without strings. Wherever you work, whatever you work on, mastery of strings will help you go a long way. So, practice it everyday.
Need more C? Check out Working With Date and Time in C.
And of course, visit the homepage for over 2,500 SDK, code libraries, and other development tools.
Recent Stories
Top DiscoverSDK Experts
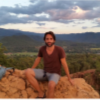
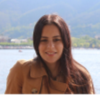
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment