Working With Hashes in Ruby
Hashes are collections of key value pairs, and unlike arrays, hashes are not ordered. Hashes are also called associative arrays. In some other languages these things are called hash table or dictionary. In hashes keys are unique and there is no such restriction on values. Like arrays, hashes are also dynamically sized. So, you can add as many elements as you want until the Ruby interpreter allows or until the system's memory is exhausted.
Before You Begin
- Make sure that you are already familiar with arrays in Ruby.
- Make sure that you have latest version of Ruby installed on your system.
- You should have basic knowledge of using command lines.
- A plain text editor or code editor is needed for writing Ruby code. You can use IDEs if you want.
- You should have read the previous posts on Ruby and practiced the examples properly.
Preparing Environment
- You should choose or create a directory where you want to put your Ruby files.
- Open command line and change the current working directory to this one.
- Create a file called hashes.rb in this directory.
- Run the Ruby file with command ruby hashes.rb to check if everything is alright.
Creating Hashes
A hash can be created by creating a new object from the Hash class.
a_hash = Hash.new
A hash can be formed by surrounding the key value pairs with a pair of opening and closing curly braces. The key-value pairs are separated by commas. The key and the value are separated by "=>". Here is an example:
ages = {"Person1" => 44, "Person2" => 24, "Person3" => 12}
There is an alternative syntax for creating a hash if all the keys in the hash are of type symbol.
a_hash = { company: "Google", type: "internet Company" }
Finding Length
Length is the number of elements a hash holds. It can be retrieved by invoking the length method on the hash.
ages = {"Person1" => 44, "Person2" => 24, "Person3" => 12}
len = ages.length
puts len
Outputs:
3
Accessing Elements
You can access elements (key-value pairs) of hashes like you do in arrays. The only difference is: for arrays you need to provide an index and for hashes you need to provide a key.
ages = {"Person1" => 44, "Person2" => 24, "Person3" => 12}
e = ages["Person1"]
puts e
Outputs:
44
Adding Elements
To add an element (key-value pair) to a hash you need to go with this syntax: hash_variable[key] = value. Let's try with an example:
ages = {"Person1" => 44, "Person2" => 24, "Person3" => 12}
ages["PersonX"] = 100
puts ages
Outputs:
{"Person1"=>44, "Person2"=>24, "Person3"=>12, "PersonX"=>100}
Removing Elements
Hashes are not ordered collections like arrays. So, for hashes you do not need to worry about whether you are removing a key value pair from the beginning, middle or from the end. We remove elements from hashes by invoking the delete method on them with the key as the parameter.
ages = {"Person1" => 44, "Person2" => 24, "Person3" => 12}
ages.delete "Person3"
puts ages
Outputs:
{"Person1"=>44, "Person2"=>24}
Clearing Hashes
Clearing a hash means that you delete all the key-value pairs from the hash and leave it empty. Without repeatedly deleting all the elements by keys from a hash, it is convenient to use the clear method.
ages = {"Person1" => 44, "Person2" => 24, "Person3" => 12}
ages.clear
puts ages
Outputs:
{}
Checking Emptiness
If the length of a hash is zero then we say that the hash is empty. But it takes more code to check the length of an array to say that is empty than just invoking the method empty? on a hash.
ages = {}
puts ages.empty?
Outputs:
true
Listing Keys and Values
We often may need to work with only the keys or only the values of a hash. To do this we have two convenient methods. The two methods are called keys and values. These two methods return an array of keys or values. Let's try with an example:
ages = {"Person1" => 44, "Person2" => 24, "Person3" => 12}
print ages.keys
print "\n"
print ages.values
Outputs:
["Person1", "Person2", "Person3"]
[44, 24, 12]
Sorting Hashes
As I mentioned before, hashes are not ordered collection of objects like arrays. But what we do if we need to sort them according to the keys? We need a two dimensional array for keeping the sorted hash. The inner arrays will be two element arrays where the first elements are keys and the second elements are values. Let's see an example:
ages = {"Person1" => 44, "Person2" => 24, "Person3" => 12}
print ages.sort
Outputs:
[["Person1", 44], ["Person2", 24], ["Person3", 12]]
Hash Iteration
Iteration means going over all the elements of a collection and optionally doing something with them. We can iterate over them with the each method and perform various operations in the code block following it:
ages = {"Person1" => 44, "Person2" => 24, "Person3" => 12}
ages.each do |key, value|
puts "Person name: #{key}"
puts "Person age: #{value}"
end
Outputs:
Person name: Person1
Person age: 44
Person name: Person2
Person age: 24
Person name: Person3
Person age: 12
and More
There are a lot of methods for hashes and in this post I have covered just the fundamental uses of hashes. You should read the official Hash class documentation to know more. I will demonstrate various uses of hashes in future guides.
Conclusion
Hash is a versatile data structure in Ruby. Where it is very difficult to create, implement and use hashes in some modern languages, hashes in Ruby are easy to create and use. Even in Java you need to write many lines of code to create a hash map with some elements. But in Ruby it is done all in one single line. To master hashes go through the examples again and again. Happy coding with Ruby.
Be sure to stop by the homepage to search and compare SDKs, Dev Tools, and Libraries.
Recent Stories
Top DiscoverSDK Experts
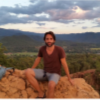
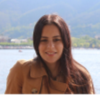
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment