Working With File Input and Output In C
The most common and familiar way of handling input and output in C is with the console. You can create a console based C application to take input from the user and display the output on the console. But working with I/O with the help of console is not always practical for all types of use cases. Most of the time we need to take input from files and write data to the file system. Output on the console is lost, but output to a file is persistent. So, input/output with the help of files is the most important way of I/O. There are other kind of I/O, for example, taking input from and providing output to network, database, etc. In this article we will learn how to take input from and save output to files.
Every operating system has its own way of interacting with the file system. There are different system calls on different operating systems to open files, to read files and to write files. With the help of the standard C library we do not need to think about different ways of reading/writing files. Standard C runtime provides us with some very convenient functions to interact with the file systems in a cross platform fashion.
To practice the code shown in this article, you can use any IDE or code editor you like. You can also use any compiler you like. The code shown here is not specific to any compiler. It can compile in C or C++ with almost any compiler and almost any dialect of the C programming language.
File I/O Functions and Types From stdio.h
The stdio.h library (or you can refer to it as a header file in the standard C library) contains the necessary functions, type declarations and macros for working with different aspects of file systems. The most important type we need to interact with files is FILES. When we open a file, C keeps track of that open file with the help of a pointer to FILE. It can be declared follows.
FILE *fp;
So, the initial code should look like the following:
#include <stdio.h>
int main(){
FILE *fp;
return 0;
}
Hit compile and run to see if everything is alright.
The other functions we need are:
- fopen
- fread
- fwrite
- fclose
We will discuss them in details in the following sections.
Opening A File
To work with a file we need to open the file. On success the function responsible for opening file will return a pointer to FILE type. To open a file we need to call the function fopen(). fopen() takes two parameters. The first parameter is the file name or the file path as string and the second parameter is the mode in which we want to open the file. The mode is represented by a string too. Mode "r" tells the fopen() function to open the file in read mode. "w" opens the file in write mode. In write mode, if the file already does not exist, then a new file with the name will be created.
Let's say we want to open a file in write mode. We are going to give the file name as my_file.txt. In the subsequent sections we will learn to put content in the file.
#include <stdio.h>
int main(){
FILE *fp;
fp = fopen("my_file.txt", "w");
return 0;
}
Hit compile and run to see if everything is alright. You will also see that a file has been created in the current working directory with the name my_file.txt.
Writing to a File
To persist data to a file we need to write data to it. The standard C library provides a function called fwrite() to write data to a file. This function accepts four parameters. The first parameter is a pointer to an array of data/elements to be written to the file, the second parameter is the size of each element in the array to be written and the third parameter is the number of such elements from that array to be written to the file. The fourth parameter is the pointer to the file, that is the pointer to FILE type returned by fopen() function.
Let's say we want to write a string to a file. A string is nothing but a collection of characters. So, the first parameter we want to pass to the fwrite() function is the pointer to the string or the pointer to the character array. We know that, every character has a size of one byte. So, the second parameter we are going to pass is the integer 1. We want to write the whole string to the file. So, the third parameter should be the length of the string. We are going to take the length of the string with the help of strlen() function. So, our code should look like the following.
#include <stdio.h>
int main(){
char * my_str = "Hello, I am string and I am going to be persisted to a file called my_file.txt";
FILE *fp;
fp = fopen("my_file.txt", "w");
fwrite(my_str, 1, strlen(my_str), fp);
return 0;
}
Hit compile and run. Open the file called my_file.txt with a text editor to see if the text in the string is saved there.
Closing a File
Every open file is a resource to the operating system. After doing work on the file we should close it to release the resource of the operating system. It is very easy to close a file in C. You just need to call the fclose() function with the file pointer as a parameter. So, our final code should look like the following.
#include <stdio.h>
int main(){
char * my_str = "Hello, I am string and I am going to be persisted to a file called my_file.txt";
FILE *fp;
fp = fopen("my_file.txt", "w");
fwrite(my_str, 1, strlen(my_str), fp);
fclose(fp);
return 0;
}
After closing the file, you will not be able to interact with the file anymore. If any data is buffered in the file buffer, the buffer will be flushed before the file is closed.
Reading Files
I have intentionally put the reading section at the end of the article. To take input from a file we need to read the file with the help of fread() function. The fread() function is similar to the fwrite() function. In this case the data is taken from the file and is put in the array. Let's read the file we already wrote with fwrite(). So, our code should look like the following:
#include <stdio.h>
int main(){
char my_str[200];
FILE *fp;
fp = fopen("my_file.txt", "r");
fread(my_str, 1, 78, fp);
my_str[78] = '\0';
fclose(fp);
printf("%s", my_str);
return 0;
}
Hit compile and run. If everything is alright then we should see the content of the file on the console. I have put 78 as the third parameter. The total length of the file or the number of characters of the file is 78. You do not need to put that value in that way. You can determine the number dynamically. We will learn about that in some future lesson.
C has many functions and some more mode to interact with files. But what I have shown here can be used in place of any other functions. Other functions provide abstraction over these fundamental functions. Also there are some other very fundamental functions called ftell(), fseek() etc. We will learn about them in future. In the meantime, you are advised to practice the code in this article and come up with some new ideas.
Recent Stories
Top DiscoverSDK Experts
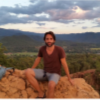
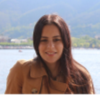
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment