Working With Date and Time in C
Almost all real life applications need to work with date and time. Date and time calculation is not just some addition, subtraction, multiplication and division of numbers—it more complicated than that. And while working with date and time is not a breeze, it’s not overly difficult either. You need to have some concepts clarified before working with date and time in C. The C standard library provides functions, types, structures, and macros for working with it. You need to include the time.h library for getting all the functions related to date and time.
Have you ever wondered, how your computer remembers the time of the day even when it is shut down? Have you ever wondered about how your CPU is aware of how long it is running? Do you know when time was started in your computer's view? You should ask yourself all these questions before working with date and time in any programming language.
Every modern computer system has a dedicated clock for remembering the time of the day. If you have a desktop computer, you can open its case to see a small circuit and a slim battery on the motherboard that helps your computer keep track of the time of the day and the date. Even when your computer is powered off, that clock keeps running to provide your computer or operating system the current date and time when it is brought to life. The microprocessor also has a clock in it. But this clock does not measure the time of human civilization. It counts the time of how long is it running. The clock embedded with microprocessor provides high precision of time difference. Using that clock we can also determine how much time some part of our code or program is taking for execution.
Epoch Time
The concept of epoch time is very important in understanding the internal working of date and time inside your operating system, software, etc. In a general sense, Epoch is a point of time in a human's life or in the history of human civilization when something notable happened. You can get better definition in a dictionary. I am not going to talk about what epoch is in a general sense. I am going to talk about the Epoch Time of computer systems. Epoch Time is the point of time from when computer systems think that time has started. If we think about us, the human race, to us the start of the time is different from different points of view. Some say that before the beginning of this universe the time was frozen at 00:00:00, some says that time has no beginning and no end. Scientist also have different opinions. But for our convenience we created a calendar system. We divided an year into months, month into days, days into hours, hours into minutes, minutes into seconds, and so on. Different calendar systems agreed to count time from different point of civilization.
But for computer systems we all had to agree on one point from where computer systems could think that the time started and thus, according to that we could convert time into different forms in different calendaring systems. So, computer gurus agreed to mark 00:00:00 of January 1, 1970. The clock used inside the computers keeps track of time in seconds (or sometime in milliseconds). So, the clock inside our computer thinks that 00:00:00 of January 1, 1970 as 0 seconds and counts forwards. Epoch time is also known as Unix time and and POSIX time.
Getting Started with Coding for Date and Time
To get started with date and time programming in C you can choose any code editor, IDE or compatible C compiler. The time.h is in the standard C library, so you do not need to worry about its availability. Every C runtime is obliged to implement the functionality of the time library. We are also going to output the results on the screen, so we need to include the stdio.h. Our bare bones initial code should look like the following:
#include <stdio.h>
#include <time.h>
int main(){
return 0;
}
Hit compile and run to see if everything is alright.
Types Related to Date and Time
There are four very important types related to date and time functions. They are clock_t, time_t, size_t, and tm. If you have gone through the beginner stage of C programming, you should already have knowledge about size_t. time_t is nothing but a long to represent time in seconds. tm is a C structure. It can hold a lot of data related to date and time in C.
The tm Structure
The tm structure is a very important structure in the time.h library that can contain information about day, week day, year, month, day of the month, hour, minute, second and the information about daylight saving time. The declaration of the structure looks like the following:
struct tm {
int tm_sec;
int tm_min;
int tm_hour;
int tm_mday;
int tm_mon;
int tm_year;
int tm_wday;
int tm_yday;
int tm_isdst;
}
We will see usage of this structure in subsequent sections of this article.
Getting the Current Date and Time in Seconds
The current date and time can be represented as seconds. Other functions use that data to represent date and time in more humanly fashion. To get the current time in seconds since epoch time we have to use the time() function.
#include <stdio.h>
#include <time.h>
int main(){
time_t cur_time_in_sec;
time(&cur_time_in_sec);
printf("%i", cur_time_in_sec);
return 0;
}
Outputs:
1501954769
The second represents the date and time of GMT: Saturday, August 5, 2017 529 PM.
Getting the Current Date and Time Directly as String
There is a function called ctime() that accepts the current time in seconds and returns a string in the form of "day month year hours seconds". We can get the current time in second from time() function.
#include <stdio.h>
#include <time.h>
int main(){
char * time_as_str;
time_t cur_time_in_sec;
time(&cur_time_in_sec);
time_as_str = ctime(&cur_time_in_sec);
printf("%s", time_as_str);
return 0;
}
Outputs:
Sat Aug 05 23:47:11 2017
Getting UTC/GMT Date and Time as tm Structure
It is not always useful to get the time as second or in a human readable string format. Sometimes we need more information. Sometimes, we need every specific detail of the date and time. A tm structure with the current date and time info is returned by the gmtime() function. This function accepts time in seconds as the first parameter.
#include <stdio.h>
#include <time.h>
int main(){
struct tm * time_struct;
time_t cur_time_in_sec;
time(&cur_time_in_sec);
time_struct = gmtime(&cur_time_in_sec);
printf("%s : %i\n", "Current second of the current minute is", time_struct->tm_sec);
printf("%s : %i\n", "Current minute of the current hour is", time_struct->tm_min);
printf("%s : %i\n", "Current hour of the day is", time_struct->tm_hour);
printf("%s : %i\n", "The day of the month is", time_struct->tm_mday);
printf("%s : %i\n", "The month number counting from zero is", time_struct->tm_mon);
printf("%s : %i\n", "The year is", time_struct->tm_year);
printf("%s : %i\n", "The weekday no counting from Sunday is", time_struct->tm_wday);
printf("%s : %i\n", "The number of day since January 1 of this year is", time_struct->tm_yday);
printf("%s : %i\n", "Is DST set", time_struct->tm_isdst);
return 0;
}
The output looks like the following at the time I am executing it.
Current second of the current minute is : 7
Current minute of the current hour is : 16
Current hour of the day is : 18
The day of the month is : 5
The month number counting from zero is : 7
The year is : 117
The weekday no counting from Sunday is : 6
The number of day since January 1 of this year is : 216
Is DST set : 0
Getting Formatted Time According To Needs
It's not always convenient and useful to get time in seconds or as tm structure. We may have our specific use cases when we want the current date and time in a specific format. To do this we have a function strftime(). This function accepts four parameters. The first parameter is a pointer to a character array to which the result will be put after calling this function. The second parameter is the count of bytes that should be output to the array. The third parameter is a string that contains the format in which we want the date and time. The fourth parameter is an instance of the tm structure.
Let's say that we want to have the current date and time in the form of "sec hour, Date MonthName, Year".
#include <stdio.h>
#include <time.h>
int main(){
char time_str[200];
struct tm * time_struct;
time_t cur_time_in_sec;
time(&cur_time_in_sec);
time_struct = gmtime(&cur_time_in_sec);
strftime(time_str, 199, "%S:%M:%I, %d %B, %Y", time_struct);
printf("%s", time_str);
return 0;
}
The output is:
37:52:06, 05 August, 2017
The format we used for this output is "%S:%M:%I, %d %B, %Y". Please look at a reference guide of a C standard library to learn about which character or which symbol represents what part of date and time. I would like to discuss the formats and various uses of it in a separate article in future.
There are some other functions in the standard C library about date and time. Try to have a look at the standard C library reference guide you have it in your shelf to know the use of various functions. I will write another article on date and time in C to discuss in more depth.
Interested in Java too? Check out Working with Date and Time in Java.
Recent Stories
Top DiscoverSDK Experts
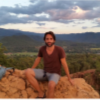
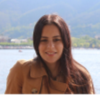
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment