Understanding JSON in JavaScript
JSON stands for 'JavaScript Object Notation'. It was initially created for JavaScript and it has become a de-facto standard for exchanging structured data over the web. All the modern languages and frameworks use it for various data exchanging over the web and for various other purposes.
JSON is not limited to exchanging data between servers and browsers. It is also used for storing structured data, used as the intermediate format of transforming data from one format to another, transferring data between servers and for a lot of things. JSON files are also used as configuration on various systems.
In this guide I am going to show you how to work with JSON in JavaScript. So, you need to have good working knowledge of JavaScript in order to follow along with this guide.
What JSON Looks Like?
As you already have worked with JavaScript you should know what JavaScript object literals look like, how arrays look, and how other primitive values look. JSON looks like them when there is only data seen from the perspective of JavaScript. The main difference is that JSON can only deal with data and JavaScript objects can hold functions and various other JavaScript objects. All JavaScript objects cannot be serialized and transformed over the network or stored in files. But JSON can be serialized and deserialized on almost any platform and in almost any language. The another thing you must remember is that what cannot be represented in plain text cannot be used in JSON. Do not confuse plain text with string objects.
The most commonly seen JSON consists of key-value pairs of data enclosed in curly braces. Here is an example:
{
"first_name" : "Sabuj",
"last_name" : "Sarker",
"email" : "md.sabuj.sarker@gmail.com",
"is_developer": true
}
But remember that JSON can be arrays, pure strings, or number literals too. Example below:
["Programming", "is", "fun"]
In this guide we will limit our discussion to that curly brace notation and the key value pairs.
Using JSON Inside HTML Files
JSON files are usually stored with the .json file extension. But JSON can be used directly inside .js files or inside script tags in HTML files. In .js files or inside the script block we represent JSON as JavaScript object literals. So, it can be represented like below:
var person = {
"first_name" : "Sabuj",
"last_name" : "Sarker",
"email" : "md.sabuj.sarker@gmail.com",
"is_developer": true
}
Warning: Though JSON looks like JavaScript object literal, they are not the same thing. So, to not treat use them both in the same way.
Note: You must remember that JSON is a data format and making something look something else does not make them the same. Data in certain data formats can be serialized and deserialized. Objects may or may not have that characteristics.
Difference Between JavaScript Objects and JSON
I mentioned a few differences above and now let's see some more with examples. I mentioned that only data can be stored inside JSON, but if it were a JavaScript object, we could add anonymous functions to it, like below:
{
"first_name" : "Sabuj",
"last_name" : "Sarker",
"email" : "md.sabuj.sarker@gmail.com",
"is_developer": true,
"display_name": function(){
console.log(this["first_name"]);
}
}
The above is not valid JSON data.
Again, in JavaScript object literals or objects we could omit the quotation mark like below:
{
first_name : "Sabuj",
last_name : "Sarker",
email : "md.sabuj.sarker@gmail.com",
is_developer: true
}
This is a valid JavaScript object but not valid JSON. Looks can be deceiving.
Also remember that keys in JavaScript can be quoted with single quotes but in JSON you should avoid that.
Remember: JSON data can be retrieved from external sources or put as serialized strings inside your JavaScript code. If it is directly put as an object literal inside you JavaScript code, we call it a JavaScript object—not JSON. But it is OK to think of them as the same until you put anything that is not a built-in data type in JavaScript.
Accessing and Manipulating JSON Data in JavaScript
Once the JSON data is deserialized in your JavaScript environment it becomes a pure JavaScript object. So, you can access the values with the help of keys with dot notation like person.first_name or with subscript notation like person["first_name"].
The same applies for adding more key values to the object. Example below:
person.github = "SabujXi"
person["age"] = 15
And the same applies for manipulating the existing values:
person.first_name = "Justt"
person["last_name"] = "Arifin"
Just remember not to include anything that is not data if you want to serialize it later, otherwise it is alright to do that. Also remember that adding or manipulating data shown in different ways above does not make it non-serializable.
If the JSON we are discussing is an array we have to access the values with index, manipulate and delete it with index:
var a =["Programming", "is", "fun"];
a[0];
a[0] = "Coding";
As you have prior JavaScript knowledge, you should well know what to do with other types of data.
Nested Objects and Arrays
You can put nested objects and arrays inside JSON. Here is an example:
{
"first_name" : "Sabuj",
"last_name" : "Sarker",
"email" : "md.sabuj.sarker@gmail.com",
"is_developer": true,
"social_medias": [
{"facebook": "https://facebook.com/SabujXiP"},
{"twitter": "https://twitter.com/SabujXi"}
]
}
Serializing JSON in JavaScript
Converting some data or objects into a text or binary format is called serializing. From JavaScript’s perspective, serializing is converting objects into strings. We need to serialize data if we want to send it to another system, save to file, send over the network. Unlike many other languages, serializing objects into JSON is very easy in JavaScript.
To serialize objects we need to call JSON.stringify() with the object as the parameter. Example below:
var person = {
"first_name" : "Sabuj",
"last_name" : "Sarker",
"email" : "md.sabuj.sarker@gmail.com",
"is_developer": true
}
var serialized_as_string = JSON.stringify(person);
Now you can send the string over the network, display to the user or if you are in a NodeJs environment, you can save it to file.
Deserializing JSON Strings into JavaScript Objects
Like serializing, deserializing is also as easy as a single function call. You need to pass the serialized JSON string to the JSON.parse() function to convert it to a plain JavaScript object.
Conclusion
This guide is an introductory guide to JSON in JavaScript. In some future guides I will try to explain with code to show how to send and receive data from browsers to servers and servers to browsers in JSON with the help of JavaScript. If you have come from another language you may be confused to see the similarity between JSON and JavaScript object literals. Check back soon for articles about how to work with JSON in other languages. You are advised to practice the code shown here and print the output on the console with console.log() to understand things better.
Recent Stories
Top DiscoverSDK Experts
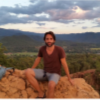
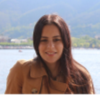
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment