Test Driven Development
Test Driven Development is a new approach for developing software in which a developer first writes tests for the same amount of production level code to fulfill those test.
The primary goal of test driven development is specification and not validation. In other words, it's one way to think through the requirements before you write your functional code.
Getting Started
Sometimes, getting started is all that it takes to continue with it. Try following this approach when working on a project:
- Just do it. Write a test case for what you want to do, and then write code that should pass the test. If you pass your test, great, you can move on to writing cases where your code will always fail (2+2 should not equal 5, for example).
- Once all of your tests pass, write your actual business logic to do whatever you want to do.
- If you are starting from scratch make sure you find a good testing suite that is easy to use. If you use PHP for example, PHPUnit and SimpleTest work well. Almost all of the popular languages have some xUnit testing suite available to help build and automate testing.
Why to use TDD?
Here are three reasons that TDD might help a developer or team:
- Better understanding of what you're going to write
- Enforces the policy of writing tests a little better
- Speeds up development
One reason to write the tests first is to have a better understanding of the actual code before you write it. To everyone, this is the main advantage of test driven development. When you write the test cases first, you think more critically about the corner cases. It's then easier to address them when you write the code and ensure that they're accurate.
Another reason is to actually enforce writing the tests. Often when people do unit-testing without the TDD, they have a testing framework set up, write some new code, and then quit. They think that the code already works just fine, so why write tests? It's simple enough that it won't break, right? But now you've lost the advantages of doing unit-tests in the first place (completely different discussion). Write them first, and they're already there.
Writing these tests first could mean that there is no need to launch the program in a debugging environment (slow, especially for larger projects) to test if a few small things work. Of course there's no excuse for not doing so before committing changes.
Convincing yourself or other people to write the tests first may be difficult. You may have better luck getting them to write both at the same time which may be just as beneficial.
Benefits of TDD:
When a team starts to follow the TDD approach, it gains many advantages over the course of the project.
- Get immediate feedback if your code is working or not, so you can find bugs faster.
- By seeing the test go from red to green, you know that you have both a working regression test and working code.
- You gain confidence to refactor existing code, which means you can clean up code without worrying what it might break.
- At the end you have a suite of regression tests that can be run during automated builds to give you greater confidence that your codebase is solid.
Having said many good things about TDD approach, it doesn’t mean there are no downsides to it. Let’s look at them next.
Downsides of TDD
Several downsides of TDD regression approach are:
- Big time investment. For the simple case you lose about 20% of the actual implementation, but for complicated cases you lose much more.
- Additional Complexity. For complex cases, your test cases are harder to calculate. I'd suggest in cases like that to try and use an automatic reference code that will run in parallel in the debug version or test run, instead of the unit test of simplest cases.
- Design Impacts. Sometimes the design is not clear at the start and evolves as you go along which will force you to redo your test causing a big time lose. I would suggest postponing unit tests in this case until you have some grasp of the design in mind.
- Continuous Tweaking. For data structures and black box algorithms unit tests would be perfect, but for algorithms that tend to be changed, tweaked or fine tuned, this can cause a big time investment that one might claim is not justified. So use it when you think it actually fits the system and don't force the design to fit to TDD.
TDD is hard!
It is much harder than one might think. Often projects done with TDD end up with a lot of code that nobody really understands. The unit tests often test the wrong thing, the wrong way. And nobody agrees what a good test should look like, not even the so called gurus.
All those tests make it a lot harder to "change" (opposite to refactoring) the behavior of your system and simple changes just becomes too hard and time consuming.
If you read the TDD literature, there are always some very good examples, but often in real life applications, you must have a user interface and a database. This is where TDD gets really hard, and most sources don't offer good answers. And if they do, it always involves more abstractions: mock objects, programming to an interface, MVC/MVP patterns etc., which again require a lot of knowledge, and... you have to write even more code.
BDD vs TDD
BDD is termed as Behaviour Driven Development.
BDD provides a new vocabulary and thus focus for writing a unit test. Basically it is a feature driven approach to TDD.
There is no difference between BDD and TDD. BDD is TDD done right. TDD done right is BDD. The problem is that doing TDD right is hard, or more precisely learning how to do TDD right is hard. The reason is that TDD has absolutely nothing whatsoever to do with testing, but it is hard to understand that important fact when all the terminology is about testing. So, BDD literally is just TDD with all the testing terminology replaced with behavioral examples terminology. It's like that "try not to think of a pink elephant" thing.
Conclusion
So be careful... if you don't have an enthusiastic team and at least one experienced developer who knows how to write good tests and also knows a few things about good architecture, you really have to think twice before going down the TDD road.
Recent Stories
Top DiscoverSDK Experts
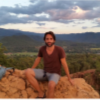
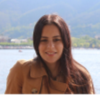
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment