Storage Options In Android
Almost no real life application is possible without some data persistence layer. Persistence layers are needed for saving preferences, storing structured data, saving and restoring serialized objects, storing static files and a lot of other things. In this article I am going to discuss various ways of storing data in Android. Android has following ways of storing data:
- Application Resource
- Preferences
- File System
- Database
- Network
Application Resource
Storing data as application resources is the easiest way of storing data. You can store any type of binary or text file as a resource of the application during application development. Usually, we store files in the 'res/raw' directory. You can store files in other locations too. Many programmers like to use the directory 'assets' for this purpose. The 'res/raw' directory has an advantage that the aapt tool will include the files in the R class and you will be able to refer to the files like R.raw.the_file. When you store files outside of res directory (e.g. assets), you will not be able to refer to them with R class as R.raw.the_file.
Storing files in this way has some disadvantages though. The biggest disadvantage is that you cannot modify the file included as a resource. That means, your files are read only. But, if you do not need to modify the files at runtime then this is a good option. Another disadvantage is that when you no longer need the files you cannot remove them to save some space. Resources also increase the application size. If the average size of files is not more than few hundred kilobytes then this is not a problem either, unless you use a very old device with a small amount of ROM.
How to use these files at runtime:
There is more than one way of accessing application resources or files. If you are using Java to develop android applications then assume that the files are being packed in a .jar file and you are going to access them with pure Java code.
If you want to refer to the files with R class identifiers, you need to call the method getResources() to obtain the resources object. If you want to open the file as InputStream, call openRawResource(R.raw.the_file) on the resources object. So, the code should look like the following:
InputStream inpStrm = getResources().openRawResource(R.raw.the_file);
If you put the files in the assets directory, you can call getAssets() to get the assets object and call open("path/to/file.ext") on the assets manager object to get an inputstream. So, our code should look like the following:
InputStream inpStrm = getAssets().open("path/to/file.ext");
Use your Java knowledge to use other methods to access the resources or static files.
Preferences/shared Preferences
By the name it can be thought that only preferences of applications can be stored in preferences, but this is not the case. You can use it for other purposes as well—the name is somewhat misleading. We usually call it "Shared Preferences", but it does not mean that data of shared preferences are shared among applications. It has a different semantic.
Preferences is a way of storing primitive data as key value pairs. The key must be a string and the values can be any of the Java primitive data types e.g. integer, double, boolean, string, etc. Though strictly speaking, string is not a primitive data type, a string can be stored as a value. The class SharedPreferences is responsible for working with data stored as shared preferences. Under the hood, preferences files are pure xml files. These files are unencrypted. So, do not store sensitive data with shared preferences.
If you need only one set of key value pairs of data, you can call getPreferences() on your activity. If you need more than one set of preferences then you can call getSharedPreferences() with a name as string. getPreferences() accept an integer mode parameter—you can pass predefined constants as the mode. getSharedPreferences() accepts name as the first parameter and mode as the second parameter. To put values in the preferences, you need to create an Editor object first. On the editor object invoke putString() to put string values—there are other methods for other types of values. You must invoke commit() to persist the data put into the editor object.
sharedprefs = getSharedPreferences("my_preferences1", Context.MODE_PRIVATE);
SharedPreferences.Editor editor = sharedprefs.edit();
editor.putString("name", "Md. Sabuj Sarker");
editor.putString("position", "Software Engineer");
editor.putString("email", "md.sabuj.sarker@gmail.com");
editor.commit();
Try this code in a real android application and keep practicing.
File System
It is no surprise that you can access and modify files in file systems in a sophisticated operating system as Android. If you have experience in working with files in Java, you can apply the same knowledge for android when you are developing android applications with Java. In android there are two types of storage where file systems reside:
- Internal Storage
- External Storage
The concept of Internal and External are virtual rather than physical here. By external it does not mean that they have to be an external SD card or an USB flash drive connected with OTG cable. They can be the same physical (flash) disk or can be different ones. Files can be saved directly on the device's internal storage. Files stored on the internal storage are private to the application by default. In a normal situation other applications cannot access those files. Also, usually they cannot be browsed by a file manager unless the device is rooted and a special file manager is in use. Storing files on the internal storage has a disadvantage that the files stored on the internal storage will be erased when the application is uninstalled. External storage is public. That means, any application can access the same files without any restriction. The files stored on the external storage will not be erased if the application is removed. You can access the files with other applications and any file manager.
Let's say, you want to store a file on the internal storage with the name of 'my_data.txt'. Calling openFileOutput() with the file name and file mode will return a FileOutputStream object.
String data = "Hello, I am just a single line of plain text";
FileOutputStream fos = openFileOutput("my_data.txt", Context.MODE_PRIVATE);
fos.write(data.getBytes());
fos.close();
Armed with your Java knowledge, try to work with external storage by yourself. Also, check out my article working with files in Java, which will help. I plan to write a dedicated article on working with internal and external storage in Android in future.
Database
Android provides full support for SQLite database. Android does not provide JDBC API for working with database. JDBC is a resource heavy system and is not suitable for mobile phone or similar devices with limited power and resources. That's why Android chose a lightweight method. Android also provides wrappers around SQLite database and its APIs.
Database in Android is a broad topic and I plan to write one or more dedicated articles on working with database in Android. Writing a small example here would just confuse you. So, I am not even providing an example in this section.
Network
There is nothing special in storing data over network as advertised in various android tutorials. If you can communicate over the the network with your code, you can read, write data over the network. Take a look at the article I wrote where I described how to work with HTTP(S) in Java. Another article describes how to go more deep and work with sockets in Java. You can also use various Android backend services found online. Also, you can make your own backend server or micro-service if you have some web application backend programming knowledge. In the future, I will provide you with some practical examples on how to store and retrieve data over the network or on the cloud. Let me remind you again that there is nothing special about storing data over the network or on the cloud as advertised in various tutorials, articles, or books. Actually, most of the time Android developers end up using some third party APIs from some third party service provider to store and retrieve data over the network. It is expected that you know Java better before coming to Android programming as the primary language of Android programming is Java. And thus, if you know how to interact with the network or cloud infrastructures with Java, you already know how to do that in Android. Still, some examples and explanation with Android can be useful and I will try to do that in future in separate articles.
Conclusions
This article is an overview of various ways of storing and retrieving data in the land of Android programming. Almost no section can be considered complete in this article as each requires special attention. For the same reason I plan to write different dedicated articles on different storage options in Android. But, going further in the journey, try to think about different options again and to understand them better to help choose which one you really need.
Stop by the homepage for the best SDKs, APIs, and other dev tools.
Recent Stories
Top DiscoverSDK Experts
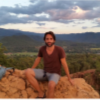
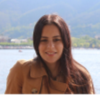
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment