All the ways to reverse a string in python
String is a very popular type in python. It has many method and its very easy to use but we need to remember that strings in python are immutable – that is you cant change the string value. When you do any change python creates a new string and change the reference to point to the new one. The old is deleted using the garbage collector
Consider the following example:
x='hello'
x+='M'
instead of adding one character to the string , python creates a new string and change the reference (x) to point the new one
if we do the same In a loop, it will cost much more CPU time and memory. Consider the following function:
def strtest1(num):
x='hello'
for i in range(num):
x+="M"
return x
On each loop iteration we create a new string
if we call the function with num=10000 it will create 10000 different strings with len ranging from 5 to 10005 and cost at least 50000000 characters - at least 100MB of memory for getting a 10005 characters string as a result
The garbage collector has a lot of work cleaning this memory
100MB to create 10000 chars string (around 20kb) !!!
it also takes too much time:
In [14]: %%timeit
...: strtest1(10000)
...:
1000 loops, best of 3: 1.19 ms per loop
In [15]: %%timeit
...: strtest1(10000000)
...:
1 loop, best of 3: 1.5 s per loop
when using a list we have a reverse method because list is mutable. Reversing a string is a costly task (memory and time) so its not exists as a simple method
If we still want to reverse a string lets see how can we do that:
Trivial way:
loop over all characters from end to start and concat to a new string
def revstring1(str1):
res=""
for i in range(len(str1)-1,-1,-1):
res+=str1[i]
return res
In [21]: %%timeit
...: revstring1("hello"*1000)
...:
1000 loops, best of 3: 731 µs per loop
Using slicing (the winner)
def revstring2(str1):
return str1[::-1]
In [26]: %%timeit
...: revstring2("hello"*1000)
...:
100000 loops, best of 3: 11.4 µs per loop
this is a little tricky.
we can slice a string using indexing in the format [start:end:jump], if we use it with negative jump value, it will slice the string in reverse order.
Note the big difference from the trivial way
Using reduce (python 2.7 only)
def revstring3(input):
return reduce(lambda x, y: y + x, input)
In [26]: %%timeit
...: revstring3("hello"*1000)
...:
100000 loops, best of 3: 1.4 ms per loop
The reduce function uses lambda experssion starting from the first character and the rest and each iteration with:
This is the slowest way.
converting to a list
def revstring4(str1):
ls=list(str1)
ls.reverse()
return "".join(ls)
In [30]: %%timeit
...: revstring4("hello"*1000)
...:
10000 loops, best of 3: 94.4 µs per loop
in this approach, we first convert the string to a list, then revers the list and convert it back to a string. this way is also efficient but not the best
Conclusions
python is a very easy to use programming language and gives the programmer many tools and ways to implement his tasks but a good python developer should learn all the ways to find the best
Recent Stories
Top DiscoverSDK Experts
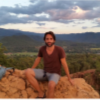
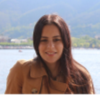
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment