Regular Expressions In Java
In a previous article I introduced you to the basic concepts of regular expressions. In this article I am going to teach you how to use regular expression in Java. That means you must have basic knowledge of Java and regular expressions to get the most out of this article.
Setting Up
To get started with regular expressions you must have JDK installed on your system. You are free to use any IDE or code editor you like. I am going to use IntelliJ IDEA from JetBrains. Create a class called JavaRegex with a main method in it in your project. Our initial code should look like the following:
public class JavaRegex {
public static void main(String[] args){
// Our code starts execution here.
}
}
Getting Started With An Example
Without just showing you the uses of different classes, let's get started with an example. We have to validate whether a string from an user's input is valid a email address or not. We need to import the Pattern class from java.util.regex package. We need a pattern string and compile it with the static method Pattern.compile(). Then invoke matcher() method on the pattern object with our input text —it will return a matcher object. To check whether the input text is a valid email address invoke the matches()* method on it.
To keep things simple I am going to define a simpler regular expression pattern and only accept mail from gmail.com
The rules and the pattern:
- The string ends with '@gmail.com'. So, the pattern looks like '@gmail\.com$'. Dot is a special character in regular expression and thus we need to escape that with a backslash. To indicate the end boundary we used dollar sign.
- The first part of the gmail address contains any number of characters from range a to z, A to Z, 0 to 9 and the dot. So, our pattern results in '^[a-zA-Z0-9.]+'. ^ indicates the starting boundary. [] represents character class. a-z means any small letter from the English alphabet. A-Z means any capital letter form the English alphabet. 0-9 means any digit from 0 to 9. + indicates that the character class can repeat one or more number of times.
- So, our final pattern looks like: ^[a-zA-Z0-9.]+@gmail.com$
- We can make our pattern matching case insensitive. But in this example we are going to make the string lowercase before working on that.
- It could be more complex pattern, but I am keeping it simple so that you can understand it well.
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class JavaRegex {
public static void main(String[] args){
String patStr = "^[a-zA-Z0-9.]+@gmail\\.com$";
String inputText = "md.sabuj.sarker@gmail.com"; // Put whatever you want here.
inputText = inputText.toLowerCase();
Pattern pat = Pattern.compile(patStr);
Matcher mac = pat.matcher(inputText);
if(mac.matches()){
System.out.println("The input string is a valid gmail address");
}else{
System.out.println("Invalid gmail address");
}
}
}
Compile and run to see the following output:
The input string is a valid gmail address
Just change the input text to some invalid email address like the following to see the output.
String inputText = "md.sabuj.sarker @gmail.com";
I have just added an extra space before the @ symbol. Recompile and run to see the following output:
Invalid gmail address
Hurrah! We have just programmed our first regular expression program perfectly. Now, it's time to move forward and learn about the important classes of regular expression in Java. I am not going to discuss each and every class and each and every method. I am going to discuss about the most important ones. In some future article I will write dedicated articles on various classes and methods.
Pattern Class
The java.util.regex.Pattern class cannot be instantiated directly. We have to call static methods on them to get an instance of it. Pattern instances represents the compiles regular expression pattern. The most important method in this class is the compile() method. This method accepts a regular expression pattern as a string and another overloaded version of it accepts flags for that specific pattern.
Matcher Class
The Matcher class is the most important class among all. It is the class of which instances we use to match, search, find, replace, split strings along with some other important operations. There are many methods on it.
matches():
The matches() method on the Matcher instance checks whether the string matches with the pattern. It's a whole string matching.
find():
The find() method on the matcher object looks for the occurrence of the pattern in the string passed to the matcher. You can call this method repeatedly. Every time you call it, it will look for the next occurrence in the string and remember that.
start() & end():
After the occurrence found in the string we need to know where the matching of the pattern starts and where does it end so that we can work on that portion of the string.
reset():
Every time you invoke the find() method on the matcher it advances one match forward and remember the last position it worked on. But what if we want to start fresh on the same matcher object? We have this convenient reset() method for that.
replaceAll():
This method is used to replace a substring inside the string according to the pattern of the matcher object. It accepts one parameter as a sub-string that will be used as a replacement for the pattern.
and more:
There are more methods of the matcher object. I do not think it fruitful to list them all in a single article and not use them in code. I would like to describe them in future articles dedicated to separate classes/methods/special-cases. For now, keep practicing with the methods described above and also try to learn more from the official documentation of Java.
Conclusion
Regular expression is a vast topic. It cannot be covered in a single article. In this article I just tried to get you introduced and set the gear up for you for starting to code with regular expression in Java. Hopefully we’ll have more articles in the future about RegEx in Java. You are advised to keep practicing and take a deeper look into the official Java reference guide on the classes and methods related to regular expression.
And check out the regular expression category on DiscoverSDK.
Recent Stories
Top DiscoverSDK Experts
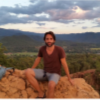
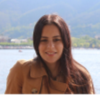
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment