React Native: Hello World app
In this tutorial, we are going to start with our first React Native mobile app. As according to tradition, we must start with a “Hello World” app.
We won’t waste too much of our time getting ready—let’s just dive right in!
Installing React Native CLI
To start, we must install the React Native CLI utility which will help you setup your project quickly.
The above image shows you the command with which you can easily install react-native-cli globally.
The simple command to install CLI is:
npm i -g react-native-cli
Project Setup
Now that we have React Native CLI utility setup on our system globally, we can start by making a project. Making a React Native project is very easy with the CLI support.
Just that simple command and we can get started with a project. The command above can take a little time to complete.
You just have to run the command:
react-native init <project-name>
Once the above process is completed, you can use any editor like Visual Studio code to work on the app. That is what we’ll use here.
Project Structure
When we open the project in Visual Studio code, we can see a basic structure for our project which contains various folders and files, some of them specifically for Android and iOs platforms.
My project structure looks like image above. Don’t feel worried if this isn’t the case with you. Understanding what we’re working with and what everything means is always great. Let’s look at the important files:
- android/ — This is the directory where all of the native Android code lives. If you dive in there you’ll find .gradle files, .java files, and .xml files. This is the directory you would open with Android Studio. You’ll rarely have to work in this directory.
- ios/ — Like the android directory this is where all of your native iOS code lives. You’ll find your xcode project in there, .plist files, .h files, .m files, etc. So if you want to open your project in xcode you would open ios/<PROJECT_NAME>.xcodeproj. You’ll rarely have to work in this directory.
- index.ios.js — This is the entry point for your ios app into the React Native code. It’s where you’ll want to register your app (via AppRegistry).
- index.android.js — Same as index.ios.js just for Android. You’ll notice that it’s exactly the same code as the ios one, we’ll work on that in the next section.
- Everything else — You probably won’t have to worry about the other stuff — it’s mostly configuration for React Native and the rest is your standard node files/directories (node_modules/ and package.json).
Running the project
Now, things have been easy but exciting. It would be real fun to see the default app running in a simulator in iOs (or an emulator in Android).
We will start by running the app in an iOs simulator first. Open the project in Finder and you will find this:
Inside the iOs directory, there is a ‘.xcodeproj’ file which you can run to start the XCode to come up and provide you with an option to run the project.
As soon as you hit the Run button, the iOs simulator will load your app. We can select one of the simulators from the many options we have.
Running an app might take time for every time you open the simulator. You will see a screen like:
Just wait and the screen will appear. Let’s see how the app looks like.
Now that’s interesting, where does that text comes from? Oops, I made some tweaks. You can see your default screen when you run the app. I am going to show you the changes I made.
Making a new File
I started by making a new file in the root directory of the project called Splash.js.
Here is the code for the file:
import React, { Component } from 'react';
import { View, Text, StyleSheet } from 'react-native';
export default class Splash extends Component {
render() {
return (
<View style={styles.wrapper}>
<Text>Hello DiscoverSDK</Text>
</View>
);
}
}
const styles = StyleSheet.create({
wrapper : {
backgroundColor : 'green',
flex : 1,
justifyContent: 'center',
alignItems: 'center'
}
});
Let’s go over all what we did in the file above:
- Splash is a component. Each component in React can be rendered in different files. This way, we can make reusable components like this one which can be used with different styling and make the code shorter.
- render() method contains what needs to be rendered on the screen. Here, a View UI element is made with simple text. The View element is quite similar to an HTML element and is very clear to understand.
- Lastly, the styles const defines reusable style which can be used with more UI elements. Making the style components separately instead of inline style makes it more readable and reusable.
Now, let’s look at the index.ios.js which we modified. Here is the code in the file:
import React, { Component } from 'react';
import {
AppRegistry,
StyleSheet,
Text,
View
} from 'react-native';
import Splash from './Splash';
export default class SplashSample extends Component {
render() {
return (
<Splash />
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
welcome: {
fontSize: 20,
textAlign: 'center',
margin: 10,
},
instructions: {
textAlign: 'center',
color: '#333333',
marginBottom: 5,
},
});
AppRegistry.registerComponent('SplashSample', () => SplashSample);
Now the above file looks pretty much the same, the import statements, the component definition and using our js file Splash as <Splash /> which includes that component here.
AppRegistry is the JS entry point to running all React Native apps. App root components should register themselves with AppRegistry.registerComponent, then the native system can load the bundle for the app and then actually run the app when it's ready by invoking AppRegistry.runApplication.
Changing app’s title
Now, currently our app appears with a name of SplashDemo.
You can change the name attribute in package.json, run
react-native upgrade
and just let react overwrite the android/ios files. Don't overwrite your index files if there is unsaved code, however.
Then change your AppRegistry line from
AppRegistry.registerComponent(“SplashSample”, () => SplashSample);
To:
AppRegistry.registerComponent(“SplashDemo”, () => SplashDemo);
Conclusion
Now that we have played a lot with our application, it’s time to leave you here so that you can continue to enjoy the app architecture yourself, make and break some changes in the app.
Enjoy, it’s React Native.
Recent Stories
Top DiscoverSDK Experts
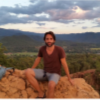
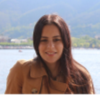
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment