React - Components with Parameters
In our last React article, we learned about creating components. But our component was a bit basic, one that doesn’t take any parameters. We want a real component that takes parameters and everything! Why? Because just like how HTML elements have properties (think href or title in an a tag), React components also need parameters. Want an example? Of course you do. Let’s use the basic component we created in the last article. In that component, we printed ‘hello world.’ Let’s go ahead and make ‘world’ a parameter.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>My first react app and component</title>
<script src="https://unpkg.com/react@16/umd/react.development.js"></script>
<script src="https://unpkg.com/react-dom@16/umd/react-dom.development.js"></script>
<script src="https://unpkg.com/babel-standalone@6.15.0/babel.min.js"></script>
</head>
<body>
<div id="content"></div>
<script type="text/babel">
class Hello extends React.Component {
render() {
return <p>Hello {this.props.target}!!</p>
}
}
const target = document.getElementById('content');
ReactDOM.render(
<Hello target='world'/>,
target
);
</script>
</body>
</html>
We pass the parameter to our component, that is now called ‘hello’, just as we would pass any parameter to any component. Easy as could be. So how do we use the parameter? In this case I decided to call the parameter target, so I access it using this.props.target. But if I wanted to call it ‘name’, then I would access it using this.props.name. And what about those braces { }? They’re part of the JSX. Everything inside of them is rendered as if it were text. In this case, it’s everything inside of this.props.target. I could put anything I want in place of the ‘world’. If I put in {1+1} I’ll get 2. Any JavaScript expression will print there. Try it yourself. Just remember, if you see braces, whatever is there will run. It’s that easy.
Children
So what’s up with children? Or in other words, what if I have a component that wraps text? How can I access it? This too is a breeze—we simply use {this.props.children}. For example, I can take everything that the component wraps and use it in my component. It’s easier to understand with an example:
class Hello extends React.Component {
render() {
return <p>{this.props.children} {this.props.target}!</p>
}
}
const target = document.getElementById('content');
ReactDOM.render(
<div>
<Hello target="World"><strong>Hello</strong></Hello>
<Hello target="Mars"><strong>Goodbye</strong></Hello>
</div>,
target
);
What’s going on here? Using this.props.children I take everything that the component Hello wraps. In one case it’s <strong>Hello</strong> and in another it’s <strong>Goodbye</strong> and we use them in my component. In this case I just have to print it out next to target.
Here’s a CodePen you can mess around with:
Notice we’re talking about pure JavaScript here, so I can mess around with the children or the target as much as I want. The class can contain as many methods as I want and I can call them as needed. What’s really important with these components is what the render returns.
class Hello extends React.Component {
render() {
return <p>{this.getChildren();} {this.props.target}!</p>
}
getChildren() {
return this.props.children;
}
}
const target = document.getElementById('content');
ReactDOM.render(
<div>
<Hello target="World"><strong>Hello</strong></Hello>
<Hello target="Mars"><strong>Goodbye</strong></Hello>
</div>,
target
);
Of course there is no problem with one component going into another. In this example, the Hello component contains the World component:
class World extends React.Component {
render() {
return <span>World!</span>
}
}
class Hello extends React.Component {
render() {
return <div>{this.props.children} <World />!</div>
}
}
const target = document.getElementById('content');
ReactDOM.render(
<div>
<Hello>Hello</Hello>
<Hello>Goodbye</Hello>
</div>,
target
);
What we really have is a declaration on two components: the first being World and the second Hello. The Hello component calls World. Yes, yes, it’s really that simple. Once I’ve declared a component, I can use it anywhere, be it in the JSX of one component or directly in an application (that is to say in ReactDOM.render). In practice, many many components contain calls to other components, so it’s not esoteric or anything. It’s a significant part of the ‘lego blocks’ of React component architecture. Any component can contain another component, which in turn can contain another component, and so on. You should definitely mess around with this to get the feel for it:
In the next article, we’ll talk about component design. Till then.
Previous Article: React Components | Next article: React Component Desigh |
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
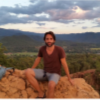
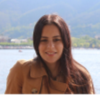
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment