OpenCV with Android Studio
OpenCV is an open sourced computer vision library. It is a machine learning software library used for image processing and computer vision techniques. OpenCV has more than 2500 optimized algorithms for Image Processing. It is mostly used for real time computer vision applications. In early days of OpenCV a few of its goals were described as
- Provide advancement in computer vision research and projects not only open but also customized projects and algorithms for basic vision infrastructure.
- Spread Computer vision knowledge by offering a common infrastructure where programmers can build better algorithms so code would be more efficient, readable and transferable.
- Customized and advanced vision based commercial algorithms by making an elegant code and logic available for free with a license that did not require to be open or free themselves.
The alpha version of OpenCV was released to the public in 2000 and five beta version were released between 2001 to 2005. The 1.0 version was released in 2006 and after 2008 OpenCV got cooperate support due to which it progressed quickly with updates with 2.0 being released in 2009. Major changes in 2nd version ware C++ interfaces, safe patterns, more functions, better performance and implementation for multi core systems. OpenCV deployed uses spanning the range from stitching street view images together, detecting intrusions in surveillance videos in Israel, monitoring mine equipment in China, helping robots navigate and pick up objects at Willow Garage, detection of swimming pool drowning accidents in Europe, running interactive art in Spain and New York, checking runways for debris in Turkey, inspecting labels on products in factories around the world and even rapid face detection in Japan. Some main features of OpenCV applications are
- Face Detection
- Object Detection
- Line detection
- Retina recognition
- Motion understanding
- Mobile robotics
- Motion tracking
- Hand gesture recognition
OpenCV is the leading global vision library and well established companies like Google, Yahoo, Microsoft, Intel, IBM, Sony, Honda and Toyota use it in their projects. Many famous and big startups make extensive use of OpenCV. The BSD-license of OpenCV makes it easy for any business, company or startup to utilize and modify any of its code.
Matlab is also used for vision applications but OpenCV has significant advantages over Matlab. One example of this is speed--OpenCV is faster than Matlab because OpenCV is based on native C while Matlab is built on Java. So when you run Matlab code it takes time to interpret the Java code into C which makes it slow. Another important factor for real time applications is portability. Matlab and OpenCV both run well on windows and Linux based Operating systems but OpenCV is more portable as any device which can run C can easily use OpenCV as compared to Matlab where Java is a must. Some other factors which make OpenCV preferable to Matlab are:
Memory Management, Development Environment, Ease of use, Resources and Cost. OpenCV can be used in many platforms like C++, Python, android and supports Windows, Linux, Android and Mac OS. But for use in each platform we must configure its related sdk in that project.
Today we will discuss use of OpenCV in android studio. We will also do some basic examples. First of all if we want to use OpenCV in Android then we need to configure it in the application. To do so we'll take the following steps.
Configuration Steps:
- Download Open CV android sdk from opencv.org
- Extract the downloaded zip folder anywhere on the system
- Now open android studio and build an empty project with blank activity.
- Go to "File --> New --> Import Module"
Now - Click on browse Go to "OpenCV- android- sdk --> sdk --> java “
- Select the java folder and click ok , next and finish
- You'll see OpenCV library added to your project in the project explorer
After that you will see lot of errors in your project
- Go to "Files --> Project Structure --> Under Modules select app and note the Compile sdk version and Built Tools Version OpenCV -->src-->gradle .build
- Now go to "Gradle Scripts--> build. gradle(Module: OpenCV)
- Replace the compile and build tool version with the one in project structure and Sync the project
- OpenCV android sdk is the wrapper for native OpenCV and in turn executes all the code in native library for optimization purpose
After that
- Go to Project Explorer and under your project
- Go to "app --> src --> main“
- Right click on main go to "New--> Directory " Enter name of directory " jniLibs " and click Ok
- Now open the OpenCV- android- sdk folder that was extracted
- Go to " sdk --> native --> libs" and copy all the folders present inside the libs folder
- Paste these copied folders in the ' jniLibs ' folder just created
Last but not least is module dependency. For module selection first
- Go to " File --> project structure --> select app under 'Modules' --> select Dependencies section “
- Click "+" button and then click on "3.Module Dependency".
- Select OpenCV and click Ok twice
- Add this line in onCreate Method of the MainActivity or any other activity you want to use opencv in “ System. loadLibrary ("opencv_java3");"
Now after OpenCV configuration in android application we are able to use OpenCV libraries and build in function so we will write our first program to convert an image from RGB to Gray scale.
Code Directions:
For that we will import some Image processing libraries in our application. Like
import org.opencv.core.Mat;
import org.opencv.core.Core;
import org.opencv.android.Utils;
import org.opencv.imgproc.Imgproc;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
After that we create one button and two ImageView’s one for original image (Loaded from resources) and second for gray scaled image.
ImageView imageView1, imageView2;
Button btn;
Then in onCreate function we include “opencv_java3” Main library.
super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); System.loadLibrary("opencv_java3");
After that we load the image from resources using Bitmap object and set the ImageView’s and onCLickListener.
Bitmap img = BitmapFactory.decodeResource(getResources(),R.drawable.pen);
imageView1=(ImageView)findViewById(R.id.imageView);
imageView2=(ImageView)findViewById(R.id.imageView2);
btn =(Button)findViewById(R.id.button); imageView1.setImageBitmap(img); btn.setOnClickListener(this);
Then the OnClick function starts where we create two Mat objects to store and process images. Mat is a multi-dimensional array which can store any size of image dynamically.
Mat source = new Mat(); Mat dest = new Mat();
After Mat objects we will convert our image from BitMaptoMat so we can process it. Utils.bitmapToMat(img, source);
Now our resourced image is stored in source. Next we will convert the image color from RGB to Gray scale. Imgproc.cvtColor(source, dest, Imgproc.COLOR_RGB2GRAY);
After image conversion we set its dimensions and convert it to bitmap to show in Image view.
Bitmap btmp = Bitmap.createBitmap(dest.width(),dest.height(),Bitmap.Config.ARGB_8888);
Utils.matToBitmap(dest,btmp);
imageView1.setImageBitmap(btmp);
Complete Code:
XML code:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools"
android: layout_width="match_parent" android: layout_height="match_parent"
android: paddingBottom="@dimen/activity_vertical_margin" android: paddingLeft="@dimen/activity_horizontal_margin" android: paddingRight="@dimen/activity_horizontal_margin" android: paddingTop="@dimen/activity_vertical_margin" tools: context="com.example.naveed.image.MainActivity">
<Button android: id="@+id/button1" android: layout_width="wrap_content" android: layout_height="wrap_content" android: text="Image"></Button>
<ImageView android: id="@+id/imageView1" android: layout_height="wrap_content" android: layout_width="wrap_content"></ImageView>
</RelativeLayout>
Java Code:
package com.example.opencv.myapplication;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.support.v7.app.AppCompatActivity; import android.os.Bundle;
import android.view.View; import android.widget.Button;
import android.widget.ImageView;
import org.opencv.core.Mat; import org.opencv.core.Core; import org.opencv.android.Utils;
import org.opencv.imgproc.Imgproc;
public class MainActivity extends AppCompatActivity implements View. OnClickListener { ImageView imageView1,imageView2;
Button btn;
@Override
protected void onCreate(Bundle savedInstanceState) { super. onCreate(savedInstanceState); setContentView (R.layout.activity_main);
System. loadLibrary ("opencv_java3");
Bitmap img = BitmapFactory.decodeResource(getResources(),R.drawable.pen); imageView1=(ImageView)findViewById(R.id.imageView); imageView2=(ImageView)findViewById(R.id.imageView2);
btn =(Button)findViewById(R.id.button); imageView1.setImageBitmap(img);
btn. setOnClickListener(this);
}
@Override
public void onClick(View v) {
Bitmap img = BitmapFactory.decodeResource(getResources(),R.drawable.pen); Mat source = new Mat();
Mat dest = new Mat();
Utils. bitmapToMat( img, source);
Imgproc. cvtColor(source, dest, Imgproc.COLOR_RGB2GRAY);
Bitmap btmp = Bitmap.createBitmap(dest.width(),dest.height(),Bitmap.Config.ARGB_8888); Utils. matToBitmap(dest,btmp);
imageView1. setImageBitmap (btmp);
}
}
Similarly if we want to take an image from the camera instead of Android resources then the following code will work.
XML code:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" Xmlns : tools="http://schemas.android.com/tools"
android: layout_width="match_parent" android : layout_height="match_parent"
android : padding Bottom="@dimen/ activity_vertical_margin" android : padding Left="@dimen/ activity_horizontal_margin" android : padding Right="@dimen/ activity_horizontal_margin" android : padding Top="@dimen/ activity_vertical_margin" tools : context="com. example. opencv.image.MainActivity">
<Button android : id="@+id/button1" android: layout_ width=" wrap_content " android:layout_ height="wrap_ content" android: text="Image"></Button>
<ImageView android: id="@+id/imageView1" android: layout_height="wrap_content" android: layout_width="wrap_content"></ImageView>
</RelativeLayout>
Java Code:
package com. example. opencv. image;
import android.content.Intent; import android.graphics.Bitmap;
import android.support.v7.app.AppCompatActivity; import android.os.Bundle;
import android.view.View; import android.widget.Button;
import android.widget.ImageView;
public class MainActivity extends AppCompatActivity { private static final int CAMERA_REQUEST = 1888;
private ImageView imageView;
@Override
public void onCreate(Bundle savedInstanceState) { super.onCreate (savedInstanceState); setContentView(R.layout.activity_main);
this.imageView = (ImageView)this.findViewById(R.id.imageView1); Button photoButton = (Button) this.findViewById(R.id.button1); photoButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent cameraIntent = new Intent( android. Provider .MediaStore.ACTION_IMAGE_CAPTURE); startActivityForResult(cameraIntent, CAMERA_REQUEST);
}
});
}
protected void onActivityResult (int requestCode, int resultCode, Intent data) { if (requestCode == CAMERA_REQUEST && resultCode == RESULT_OK) {
Bitmap photo = (Bitmap) data.getExtras().get("data"); imageView. setImageBitmap(photo);
}
}
}
Conclusion:
Here we covered the history of OpenCV and then its main features and few applications in real time. We also learned why OpenCV is preferred over Matlab. At the end section we configure OpenCV sdk in Android studio and then we access images from resource and changed its color through OpenCV library and we also learned image resizing in OpenCV. Hope this helps and good luck trying out the cool technology.
Recent Stories
Top DiscoverSDK Experts
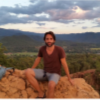
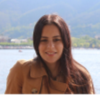
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment