Null And NullPointerException In Java Explained
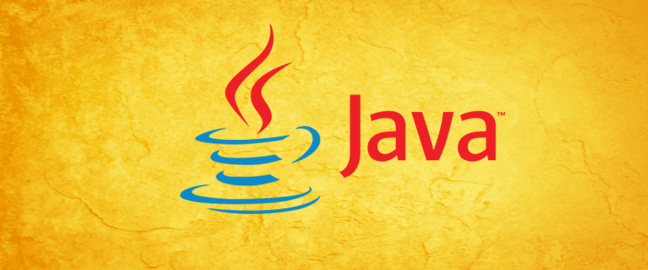
In Java, null and NullPointerExceptions are the names of two of your worst nightmares! Null is the name of a billion-dollar mistake as indicated by Tony Hoare. On a Sunday morning you will not be able to stay in bed because of it. Virtually an earthquake will take place in your office at the time of deployment because of it. You will have to browse through thousands of lines code through the whole night to fix a single bug. Unit tests will not be of much help—maybe test itself is suffering from a NullPointerException. Today, in this article, I am going to discuss such a issue and the dangerous idea of this billion-dollar mistake. Fasten your seat belts tight for a journey to the land of null.
What is null
In an ordinary sense, null is nothingness. But we are not dealing with something as simple and abstract as that. We are dealing with null in java—the name of a disaster. At the first you might say, "null is a keyword in Java." Wrong! if, while, etc. are keywords in java and they are responsible for some functionality in the language. But one fundamental characteristic they have is that they carry no value. So, null is something that carries a value—a value that represents nothingness. null is also a reserved word in java (reserved words and keywords are not the same). In java a value is either a scalar or a reference to an object. Every scalar variable has a default value, but every reference variable has a default value of null. That means an object reference can point to a real object of its type or point to null.
But, something that represents nothingness does not mean much from the programming perspective. null is a literal in java. But a different type of literal. Literally putting it somewhere will wreak havoc and will break your program with NullPointerException. A null pointer exception is the most common exception during runtime of a java program. I am going to explain these things with code later in this article.
What Is a NullPointerException
A NullPointerException is an exception that occurs due to an unwise use of null or due to some careless coding within your java program. Most of the time this exception takes place due to treating a null reference of an object like the object.
Getting Started With Coding
To get started with coding for this article you need to have JDK installed on your system. To write code you can use any plain text editor, code editor with java support or you can use a java IDE. I am going to use IntelliJ IDEA from JetBrains.
Create a project to start coding. Create a class named JavaNull with a public method in it. So, our initial code looks like the following.
public class JavaNull {
public static void main(String[] args){
// Our code stats here
}
}
Code That Does Not Compile Due To null
Let's write a very simple program that apparently seems alright, but will not compile due to null. I am not going to write something special—just a simple hello world type of program for our null.
public class JavaNull {
public static void main(String[] args){
System.out.println(null);
}
}
Hit build to see the following exception.
Error:(4, 19) java: reference to println is ambiguous
both method println(char[]) in java.io.PrintStream and method println(java.lang.String) in java.io.PrintStream match
It did not compile due to ambiguity. Along with primitive types System.out.println() accepts Object, Character Array and String—all these are of the reference type. That means null can be used in place of any of them. So, there are three overloaded version of println() that will accept null. Java compiler got confused and informed us that the the reference to println is ambiguous.
Then how do we make our java compiler to compile code that does not compile due to ambiguity? Remember that any reference type can hold null. So, we can cast null to any other object or reference type. Let's cast it to string.
System.out.println((String)null);
The complete code becomes:
public class JavaNull {
public static void main(String[] args){
System.out.println((String)null);
}
}
This time it compiles and when run it outputs 'null' on the console.
Code That Fails And Throws NullPointerException
Null is such a dangerous thing that can make your program fail at runtime instead of preventing you at compile time. Let's see how that happens.
public class JavaNull {
public static void main(String[] args){
String str = "";
str = null;
String lowerCaseStr = str.toLowerCase();
System.out.println(lowerCaseStr);
}
}
I initialized a String as an empty string and then set it to null. str variable is of type String and thus we can invoke toLowerCase() method on it. Let's try to compile/build it. There is nothing wrong at compile phase. Now, let's run it to see the following output.
Exception in thread "main" java.lang.NullPointerException
at JavaNull.main(JavaNull.java:5)
Ah! That's our beloved null pointer exception!
But how did that happen? Strings are of reference type and that means that they can hold null value. But when it holds a null value it is no longer a pure string. We cannot invoke toLowerCase() on null.
Most of the disasters happens due to such type of coding. Somehow our desired objects refer to a null and we invoke various method on that. Try this type of code with other types of objects to see NullPointerException thrown at runtime.
Get Rid Of NullPointerException
NullPointerException and null will always accompany you throughout your programming career. To save you and yourself from that deadly runtime exception you need to check whether some object refers to null or not and code accordingly. Let's say, we want to output an empty string when our string object refers to null and we want to output the lowercase version of the string when it is a real string.
public class JavaNull {
public static void main(String[] args){
String str = "";
str = null;
String lowerCaseStr = "";
if (str != null){
lowerCaseStr = str.toLowerCase();
}
System.out.println(lowerCaseStr);
}
}
Hit build and run to see that our code works perfectly this time. You can also check the existence of a null reference in more concise way like below.
String lowerCaseStr = str == null ? "" : str.toLowerCase();
Conclusion
To save you and yourself from the loophole of null you need to be cautious when you are coding in java. With some extra lines of code and a little awareness you can save the day. In future articles I will try to keep you aware of the loophole of null where possible. Keep practicing and try to come up with new ideas to defeat the NullPointerException.
Check out our guide to concurrency in Java and stop by the homepage to find the perfect software component for your next project.
Recent Stories
Top DiscoverSDK Experts
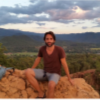
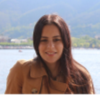
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
0 Comments
Your Comment