Non-Mutator Array Methods In JavaScript
Non-mutator methods are the methods that do not directly changes the array. Non-mutator methods may return another array or other types of data on invocation. In a previous article we discussed mutator methods in detail.
Concatenating Two Arrays With concat()
We often need to add two arrays together. We can do this by creating an empty array and looping over the other two arrays to add those elements to the new empty array. But, we do not need to solve the common problems over and over again. We can invoke the concat() method on one array by passing the second array to it to get a new array where all the elements from the both arrays exists sequentially. The concat() method does not mutate the arrays, instead it returns a new array.
var arr1 = ["a", "b", "c"];
var arr2 = ["x", "y", "z"];
var arr3 = arr1.concat(arr2);
console.log(arr1);
console.log(arr2);
console.log(arr3);
Outputs:
[ 'a', 'b', 'c' ]
[ 'x', 'y', 'z' ]
[ 'a', 'b', 'c', 'x', 'y', 'z' ]
Look at the output that the arr1 and arr2 are unchanged and a new array was returned from the concat() method.
Joining Elements of an Array With join()
Sometimes we need to join all the data from an array to create a single string. Let's say that we have an array of a few city names in Texas. We need a string with all those city names separated by commas. We invoke the join method on an array with a string parameter by which we want to join the elements. Let's say that we want the first two cities from the following array of cities in Texas.
var arr = ["Houston",
"San Antonio",
"Dallas",
"Austin",
"Fort Worth",
"El Paso",
"Arlington",
"Corpus Christi",
"Plano"
];
var str = arr.join(",");
console.log(str);
The above code outputs the following result:
Houston,San Antonio,Dallas,Austin,Fort Worth,El Paso,Arlington,Corpus Christi,Plano
Extracting Part of an Array
Sometimes we may not need the whole string but rather, only part of it. The slice() helps us to extract a part of an array. slice() returns a new array after the extraction. This method accepts two parameters. The first one tells the method about where to start extracting from and the second method specifies the end boundary. If you want to start at some index and go to the end of the array, we need not specify the second parameter.
var arr = ["Houston",
"San Antonio",
"Dallas",
"Austin",
"Fort Worth",
"El Paso",
"Arlington",
"Corpus Christi",
"Plano"
];
var part = arr.slice(0, 2);
console.log(part);
Outputs:
[ 'Houston', 'San Antonio' ]
So, we have got what we wanted.
Remember: the second parameter is exclusive. That means that the element at that index will not be included in the result.
Searching Through Arrays With indexOf()
Searching through data is a daily life operation. In JavaScript programming it is easy to search through an array with the help of the indexOf() method. This method will take a value as the argument to the method. If the value is found in the element this method will return the index of that element inside the array. If it does not find it, it will return negative one (-1). Let's try to find "London" in our list of cities.
var arr = ["Houston",
"San Antonio",
"Dallas",
"Austin",
"Fort Worth",
"El Paso",
"Arlington",
"Corpus Christi",
"Plano"
];
var idx = arr.indexOf("London");
if (idx == -1){
console.log("The city called London was not found in the array");
}else{
console.log("The city was found at the index of " + idx);
}
Outputs:
The city called London was not found in the array
Using lastIndexOf()
The method lastIndexOf() does the same thing as indexOf() but it's search does not stop when it finds something. It has the knowledge that a value may occur multiple times inside an array. It returns the index of the last occurrence of the value. Like indexOf() it returns -1 on failure.
var arr = [1, 2, 3, 4, 5, 1, 1];
var idx = arr.lastIndexOf(1);
if (idx == -1){
console.log("1 was not found in the array");
}else{
console.log("Last occurance of 1 was found at the index of " + idx);
}
Outputs:
Last occurrence of 1 was found at the index of 6
Finding the Size of Arrays
Arrays in JavaScript are dynamic and do not have a fixed size. The size may change any time by adding new elements or removing elements from it. There is no method array object to find the size. Instead, it has an attribute called length that tells us about the size of the array.
var arr = [1, 2, 3, 4, 5, 1, 1];
console.log("There are " + arr.length + " number of elements in this array");
Outputs:
There are 7 number of elements in this array
Iterating Over Arrays With forEach()
We can iterate over an array with the conventional way of using a for loop starting at index 0 and continuing up to the length of the array, getting the value by the index. We have a far more better way of doing this actually. We can use the forEach() method on it and pass a callback function to serve our purpose.
var arr = ["Potato", "Banana", "Apple", "Orange"];
arr.forEach(function(elem){
console.log(elem);
});
Outputs:
Potato
Banana
Apple
Orange
Mapping to Other Values With map()
If we want to get different values against each values in an array, we use the map() method. This method returns a new array. It accepts a callback function as a parameter that will return a value against whatever we pass to it. Let's say that we have an array of number and string data. All that string data is actually numbers in string form. So, we need to convert them to the number data types. As a result of our code we should get a new array with only the number data.
var arr = [1, "2", 3, "4"];
var arrN = arr.map(function(e){
if (typeof e == "string"){
return Number(e);
}else{
return e;
}
});
console.log(arrN);
Outputs:
[ 1, 2, 3, 4 ]
Conclusions
In this article I tried to demonstrate some array methods with proper examples. You are advised to practice all the methods regularly and use them in your daily life programming. Check back soon for articles on more advanced operations with arrays. And check out the homepage for tons of JavaScript SDKs that you can use in your programs.
Recent Stories
Top DiscoverSDK Experts
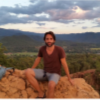
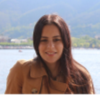
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment