Meeting React Native
Welcome to our first article on React Native. Before we get into the major concepts of this framework, let’s look at a quote from the official documentation:
React Native lets you build mobile apps using only JavaScript. It uses the same design as React, letting you compose a rich mobile UI from declarative components. With React Native, you don't build a mobile web app, an HTML5 app, or a hybrid app. You build a real mobile app that's indistinguishable from an app built using Objective-C or Java. React Native uses the same fundamental UI building blocks as regular iOS and Android apps. You just put those building blocks together using JavaScript and React.
React Native is very similar to React, but it uses native components instead of web components as building elements. So to understand the basic structure of a React Native app, you need to understand some of the basic React concepts, like JSX, components, state, and props. If you already know React, you still need to learn some React-Native-specific stuff, like the native components. This tutorial is aimed at all audiences, whether you have experience with React or not.
How it is different from PhoneGap and Titanium?
Over the years, there have been a lot of JavaScript frameworks to create native iOS and Android applications (such as PhoneGap or Titanium), so what makes React Native so special?
- Unlike PhoneGap, with React Native the application logic is written and runs in JavaScript, whereas the application UI elements are fully native. Therefore we have none of the downgrades typically associated with HTML5 UI.
- Additionally (unlike Titanium), React introduces a novel, radical and highly functional approach to constructing user interfaces. In brief, the application UI is simply expressed as a function of the current application state.
The key feature with React Native is that it looks to bring the power of the React programming model to mobile app development. It is not aiming to be a cross platform, write-once run-anywhere, tool. It is aiming to be learn-once write-anywhere. A key distinction to make. This tutorial only covers iOS, but once you’ve learned the concepts here you could port that knowledge into creating an Android app very quickly.
Features and Advantages
- Uses React: Framework for building web and mobile apps using JavaScript.
- Native: You can use native components controlled by JavaScript.
- Platforms: Supports IOS and Android platform.
- JavaScript: You can use existing JavaScript knowledge to build native mobile apps.
- Code sharing: You can share most of your code on different platforms.
- Community: Community around React and React Native is very large, and you will be able to find any answer you need.
Environment Setup
We will be using Mac OSX as our building platform. Let us setup React Native on our system and make the traditional Hello World app.
- Install Homebrew
Install Homebrew using the following command:
/usr/bin/ruby -e "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install)"
- Install Watchman
brew install watchman
- Install ReactNative
npm install -g react-native-cli
- Install Android Studio
- Install XCode
- Create First app
Run the following command to create a new app:
react-native init reactTutorialApp
- Run ReactNative packager
First we need to open the app folder in the terminal.
cd reactTutorialApp
react-native start
Keep the above window open.
- Run the app on iOs emulator
In another terminal, run the following command:
react-native run-ios
Hello World
According to the ancient traditions of the programming world, we must first build an app that does nothing except say "Hello world". Here it is:
import React, { Component } from 'react';
import { AppRegistry, Text } from 'react-native';
class HelloWorldApp extends Component {
render() {
return (
<Text>Hello world!</Text>
);
}
}
AppRegistry.registerComponent('HelloWorldApp', () => HelloWorldApp);
This will just show “Hello World” in a blank screen in the iOs Simulator.
Feel free to play around with the above code. You can also paste it into your index.ios.js or index.android.js file to create a real app on your local machine.
Once you are done playing around, be sure to run your app and feel good about having stepped where so many great programmers once were—on the Hello World milestone.
What’s with the code?
First of all, ES2015 (also known as ES6) is a set of advances to JavaScript that is now part of the official library, but not yet supported by all browsers, so often it isn't used yet in web development.
React Native ships with ES6 support, so we can use the ES6 without being worried about compatibility. import, from, class, extends, and the () => syntax in the example above are all ES2015 features. If you aren't familiar with ES2015, you can probably pick it up just by reading through sample code like this tutorial has. If you want, this page has a good overview of ES2015 features.
The other unusual thing in this code example is <Text>Hello world!</Text>. This is JSX - a syntax for embedding XML within JavaScript. Many frameworks use a special templating language which lets you embed code inside markup language. In React, this is reversed. JSX lets you write your markup language inside code. It looks like HTML on the web, except instead of web things like <div> or <span>, you use React components. In this case, <Text> is a built-in component that just displays some text.
Component and AppRegistry
So this code is making a sample Hello World App, a new Component, and it's registering it with the AppRegistry. When we're building a React Native app, we'll be making new components a lot. Anything you see on the screen is some sort of component.
A component can be pretty simple—the only thing that's required is a render function which returns some JSX to render. Whenever we see our components growing, we will break this components into smaller components.
The AppRegistry just tells React Native which component is the root component for the complete app. We won't be working with AppRegistry a lot; there will probably just be one call to AppRegistry.registerComponent in the complete app. It's included in these examples so you can paste the whole code into your index.ios.js or index.android.js file and get it running.
Conclusion
In this article and tutorial we met React Native, a Javascript framework from Facebook for building native apps using Javascript.
We also setup and ran our first app with React Native, a real milestone!
Recent Stories
Top DiscoverSDK Experts
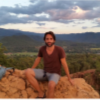
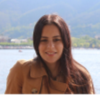
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment