Laravel Facade and Dependency injection
This article will continue where we left off in Part 6 of the Laravel tutorial series. Laravel is a powerful MVC PHP framework, designed for developers who need a simple and elegant toolkit to create full-featured web applications. Laravel was created by Taylor Otwell.
In this final article of the series, we will have a look at how to make facades and implement a Security layer in the framework which is an important skill to gain in being a PHP developer. So, let’s dive straight into the code.
Facades
Facades gives a "static" interface to classes that are available in the application's service container. Laravel "facades" serve as "static proxies" to underlying classes in the service container, providing the benefit of a terse, expressive syntax while maintaining more testability and flexibility than traditional static methods.
How to create Facade
The following are the steps to create Facade in Laravel.
- Create PHP Class File.
- Bind that class to Service Provider.
- Register that ServiceProvider to Config\app.php as providers.
- Create Class which is this class extends to lluminate\Support\Facades\Facade.
- Register point 4 to Config\app.php as aliases.
All of Laravel's facades are defined in the Illuminate\Support\Facades namespace. So, we can easily access a facade like so:
use Illuminate\Support\Facades\Cache;
Route::get('/cache', function () {
return Cache::get('key');
});
Throughout the Laravel documentation, many of the examples will use facades to demonstrate various features of the framework.
When to use Facades
Facades have many advantages. They provide a terse, memorable syntax that allows us to use Laravel's features without remembering long class names that must be injected or configured manually, taking more time. Furthermore, because of their unique usage of PHP's dynamic methods, they are very easy to test and identify bugs in.
However, some care must be taken when using facades. The primary danger of facades is class scope creep. Since facades are so easy to use and do not require injection, it can be easy to let them grow and use many facades in a single class. Using dependency injection, this potential is mitigated by the visual feedback a large constructor which tells us that our class is growing too large. So, when using facades, we need to pay special attention to the size of the class so that its scope of responsibility stays narrow.
Facades vs. Dependency Injection
One of the primary benefits of dependency injection is the ability to swap implementations of the injected class. This is useful during testing since we can inject a mock or stub and assert that various methods were called on the stub.
Typically, it might not be possible to mock or stub a truly static class method. However, since facades use dynamic methods to proxy method calls to objects resolved from the service container, we actually can test facades just as we would test an injected class instance. For example, given the following route:
use Illuminate\Support\Facades\Cache;
Route::get('/cache', function () {
return Cache::get('key');
});
We can write the following test to verify that the Cache::get method was called with the argument we expected:
use Illuminate\Support\Facades\Cache;
/**
* A basic functional test example.
*
* @return void
*/
public function testBasicExample()
{
Cache::shouldReceive('get')
->with('key')
->andReturn('value');
$this->visit('/cache')
->see('value');
}
Security
Security is one of the most important features while designing web applications and providing API endpoints to mobile and end users. It assures the users of the website that their data is secured and is not put in wrong hands.
Laravel framework provides various techniques to secure our apps and endpoints. Some of the features are listed below:
- Storing Passwords − Laravel provides a class called “Hash” class which provides secure Bcrypt hashing. The password can be hashed in the following way.
$password = Hash::make('secret');
make() function will take a value as argument and will return the hashed value. The hashed value can be checked using the check() function in the following way.
Hash::check('secret', $hashedPassword)
The above function will return a boolean. It will return true if the password matches, and false otherwise.
- Authenticating Users − Another main security feature in Laravel is authenticating user and perform an action. Laravel has made this task simple and we can do this by using Auth::attempt method in the following manner:
if (Auth::attempt(array('email' => $email, 'password' => $password))) {
return Redirect::intended('home');
}
The Auth::attempt method will take credentials as argument and will verify those credentials against the credentials stored in database and will return true if it is matched or false otherwise.
- CSRF Protection/Cross-site request forgery (XSS) − Cross-site scripting (XSS) attacks happen when attackers are able to place client-side JavaScript code in a page viewed by other users. To avoid this kind of attack, you should never trust any user-submitted data or escape any dangerous characters. You should favor the double-brace syntax ({{ $value }}) in your Blade templates, and only use the {!! $value !!} syntax, where you're certain the data is safe to display in its raw format.
- Avoiding SQL injection − SQL injection vulnerability exists when an application inserts arbitrary and unfiltered user input in an SQL query. By default, Laravel will protect you against this type of attack since both the query builder and Eloquent use PHP Data Objects (PDO) class behind the scenes. PDO uses prepared statements, which allows you to safely pass any parameters without having to escape and sanitize them.
- Cookies – Secure by default − Laravel makes it very easy to create, read, and expire cookies with its Cookie class. In Laravel all cookies are automatically signed and encrypted. This means that if they are tampered with, Laravel will automatically discard them. This also means that you will not be able to read them from the client side using JavaScript.
- Forcing HTTPS when exchanging sensitive data − HTTPS prevents attackers on the same network to intercept private information such as session variables, and log in as the victim.
Conclusion
Of course, there are plenty of other things we can do to further secure our Laravel app and API endpoints, such as ensuring browser-based error reporting is disabled so as to ensure sensitive application details aren’t exposed to a potentially malicious party. However, Laravel really does ensure a much more secure application by eliminating these three very common attack vectors.
In this final Laravel series article, we studied how to manage Facades and implement layered Security in Laravel. Hope you enjoyed the complete series!
Recent Stories
Top DiscoverSDK Experts
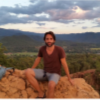
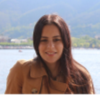
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment