Label Statements in JavaScript
If there’s one thing that always gives me trouble, it’s complex loops in JavaScript. (And recursion in JavaScript, which makes two things, but that’s a different story for a different article.)
It’s often difficult to keep track of things in nested loops. But in JavaScript, as you would expect, there are lots of features that most people don’t know about which can actually make our lives a lot easier. One of these magical features is what we call label statements which are used with loops. They can bring order to complicated looping.
Have a look at the following loop:
let str = '';
for (let i = 0; i < 10; i++) {
if (i === 1) {
continue;
}
str = str + i;
}
console.log(str); // 023456789
OK, you don’t really need to be a world-class JavaScript whiz to wrap your brain around this code. All we’ve got here is a plain old JavaScript loop that simply iterates over all the digits between 0–9 and adds them to str. But…. If the number is equal to 1, continue is executed before the loop adds the number which is why the result is 023456789 without the 1.
But, I can put a label on the loop like so:
let str = '';
myLoop:
for (let i = 0; i < 10; i++) {
if (i === 1) {
continue myLoop;
}
str = str + i;
}
console.log(str); //023456789
Take note of the two things that I added here. The first is the label called myLoop which was added in a line right above the loop itself. The second is the myLoop which comes right after continue. This makes it so that the continue is now connected to myLoop and only to myLoop—not to any other loop. If I make a typo here, I’ll get back an error along the lines of SyntaxError: Undefined label.
OK, that’s all well and good, but what’s it really good for? Well, it’s particularly useful when dealing with nested loops. It allows us to use break or continue on the outer loop from inside the inner loop. And that? Well, that pretty dern magical…
for (let outerLoop = 0; outerLoop < 4; outerLoop++) {
for (let innerLoop = 0; innerLoop < 4; innerLoop++) {
console.log(`outerLoop: ${outerLoop}. innerLoop: ${innerLoop}`);
}
}
Here, we’ve got two loops. The first, the outer loop, runs 4 times from 0 to 4. Every time it runs, it also runs the inner loop, which also runs 4 times from 0 to 4. The output of this whole thing looks like this:
outerLoop: 0. innerLoop: 0
outerLoop: 0. innerLoop: 1
outerLoop: 0. innerLoop: 2
outerLoop: 0. innerLoop: 3
outerLoop: 1. innerLoop: 0
outerLoop: 1. innerLoop: 1
outerLoop: 1. innerLoop: 2
outerLoop: 1. innerLoop: 3
outerLoop: 2. innerLoop: 0
outerLoop: 2. innerLoop: 1
outerLoop: 2. innerLoop: 2
outerLoop: 2. innerLoop: 3
outerLoop: 3. innerLoop: 0
outerLoop: 3. innerLoop: 1
outerLoop: 3. innerLoop: 2
outerLoop: 3. innerLoop: 3
But if I use a label statement, I can control the outer loop from within the inner loop. Let’s say I want it so that each time the inner loop gets to 2, the inner loop advances by one digit. Or in other words, continue. How can we do this? Glad you asked…
firstLoop:
for (let outerLoop = 0; outerLoop < 4; outerLoop++) {
secondLoop:
for (let innerLoop = 0; innerLoop < 4; innerLoop++) {
if (innerLoop === 2) {
// Use continue to avoid runs 4 and 5
continue firstLoop;
}
console.log(`outerLoop: ${outerLoop}. innerLoop: ${innerLoop}`);
}
}
Here, you can see how each time that there’s a condition in the inner loop, I can control the outer loop using continue firstLoop. The output will look like this:
outerLoop: 0. innerLoop: 0
outerLoop: 0. innerLoop: 1
outerLoop: 1. innerLoop: 0
outerLoop: 1. innerLoop: 1
outerLoop: 2. innerLoop: 0
outerLoop: 2. innerLoop: 1
outerLoop: 3. innerLoop: 0
outerLoop: 3. innerLoop: 1
What the loop is actually doing isn’t the important thing here. What’s important is that you understand how label statements work. All loops can be given a name which we can then use with break or continue from anywhere within the internal loop.
It’s definitely an interesting feature and worth knowing about.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen
Recent Stories
Top DiscoverSDK Experts
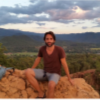
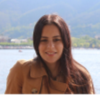
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment