Java Spring - Boot and Logging
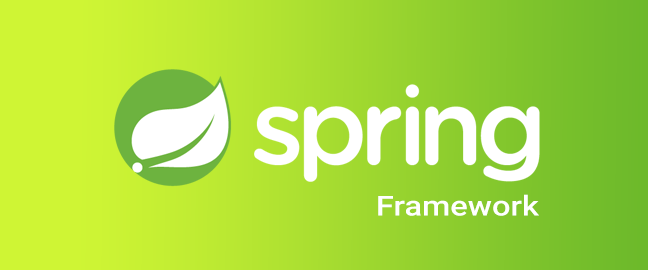
In this tutorial we will learn about Spring Boot, which is a great addition to Spring, and about logging common messages using SL4J library.
As already mentioned in our previous articles of the series, Spring framework is an open source Java platform that provides MVC infrastructure support for developing robust Java applications very easily and very rapidly using recommended MVC pattern.
Spring Boot
Spring Boot makes it easy to create stand-alone and production-grade apps which we can run with just a ‘main’ method. Most Spring Boot applications need very little Spring configuration.
Features
- Create stand-alone Spring applications
- Embed Tomcat, Jetty or Undertow directly (no need to deploy WAR files)
- Provide opinionated 'starter' POMs to simplify your Maven configuration
- Automatically configure Spring whenever possible
- Provide production-ready features such as metrics, health checks and externalized configuration
- Absolutely no code generation and no requirement for XML configuration
- Create JAR instead of heavy WARs
The only dependency in Gradle you need is:
dependencies {
compile("org.springframework.boot:spring-boot-starter-web:1.5.1.RELEASE")
}
With the above dependency included, a typical build.gradle file will look like:
plugins {
id 'org.springframework.boot' version '1.5.1.RELEASE'
id 'java'
}
jar {
baseName = 'myproject'
version = '0.0.1-SNAPSHOT'
}
repositories {
jcenter()
}
dependencies {
compile("org.springframework.boot:spring-boot-starter-web")
testCompile("org.springframework.boot:spring-boot-starter-test")
}
With maven build system, dependencies will look like:
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.5.1.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
A sample controller in a class with a main() method will look like:
import org.springframework.boot.*;
import org.springframework.boot.autoconfigure.*;
import org.springframework.stereotype.*;
import org.springframework.web.bind.annotation.*;
@Controller
@EnableAutoConfiguration
public class SpringBootController {
@RequestMapping("/")
@ResponseBody
String home() {
return "Hello Spring Boot.";
}
public static void main(String[] args) throws Exception {
SpringApplication.run(SpringBootController.class, args);
}
}
That’s it actually. With just the above code and added dependency in your build system, we have already made a Spring API.
In short, Spring framework helps you build web applications. It takes care of dependency injection, handles transactions, implements an MVC framework and provides a foundation for the other Spring frameworks (including Spring Boot).
Spring Boot consists of several (optional) modules:
- CLI: A command line interface, based on Groovy, to start/stop spring boot created applications.
- Core: The base for other modules, but it also provides some functionality that can be used on its own, for example using command line arguments and YAML files as Spring Environment property sources and automatically binding environment properties to Spring bean properties (with validation).
- Autoconfigure: Module to autoconfigure a wide range of Spring projects. It will detect the availability of certain frameworks (Spring Batch, Spring Data JPA, Hibernate, JDBC). When detected it will try to autoconfigure that framework with some sensible defaults, which in general can be overridden by configuration in an application.properties/yml file.
- Actuator: This project, when added, will enable certain enterprise features (Security, Metrics, Default Error pages) to your application. As the auto configure module it uses autodetection to detect certain frameworks/features of your application.
- Starters: Different quickstart projects to include as a dependency in your maven or gradle build file. It will have the needed dependencies for that type of application. Currently, there are starter projects for a web project (tomcat and jetty based), Spring Batch, Spring Data JPA, Spring Integration, Spring Security exist. In the (near) future more may be expected.
- Tools: The Maven and Gradle build tool as well as the custom Spring Boot Loader (used in the single executable jar/war) is included in this project.
@SpringBootApplication
Many times, main classes are annotated with @Configuration, @EnableAutoConfiguration and @ComponentScan. Since these annotations are so frequently used together, Spring Boot provides a convenient @SpringBootApplication alternative.
The @SpringBootApplication annotation is equivalent to using @Configuration, @EnableAutoConfiguration and @ComponentScan with their default attributes.
Logging
Spring Boot has no logging dependency except for the Commons Logging API, of which there are many implementations to choose from.
Let’s include SL4J dependency in maven, as shown:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
To enable logging, create a application.properties file in the root of the resources folder.
logging.level.org.springframework.web=ERROR
logging.level.com.discoversdk=DEBUG
It’s done. With its simple implementation, now you can use various log levels like debug, info, error etc.
Profile-specific logging
The <springProfile> tag allows us to optionally include or exclude sections of configuration based on the active Spring profiles. Profile sections are supported anywhere within the <configuration> element. We can use the name attribute to specify which profile accepts the configuration. Multiple profiles can be specified using a comma-separated list.
Let’s look at an example:
<springProfile name="staging">
<!-- configuration to be enabled when the "staging" profile is active -->
</springProfile>
<springProfile name="dev, staging">
<!-- configuration to be enabled when the "dev" or "staging" profiles are active -->
</springProfile>
<springProfile name="!production">
<!-- configuration to be enabled when the "production" profile is not active -->
</springProfile>
Why Spring Boot?
One can find several reasons to recommend using Spring Boot:
- With Spring boot we get the benefit of very nice and useful features such as actuator and remote shell for managing and monitoring, that improves our application with production ready feature very useful.
- Very nice and powerful properties and configuration controls. We can configure our application with application.properties/yml and extend the boot in a very simple and impressive way, even the management in term of overriding is very powerful.
- It is one of the first micro-service ready platform, and in my opinion today is the best. But even if you don't build micro-service projects with boot you can benefit from using a modern approach in which you have a auto-consistent jar that can benefit from all the features that I described above, or if you prefer you can impose the packaging as a classical war and deploy your war in any container that you want.
- Spring initializer is a cool tool attainable at the link: https://start.spring.io/ It’s is a very cool tool to create a project very quickly.
Without a web container
Simply start your spring boot app in a non-web environment:
new SpringApplicationBuilder()
.sources(SpringBootApp.class)
.web(false)
.run(args);
Also obviously, we should not add the spring-boot-starter-web dependency.
By default, spring boot launches a web container if it finds one in the classpath. Using web(false)ensures that it does not happen. Tomcat could be included by another dependency without our knowledge, so it's better to disable the web environment if that is the goal.
Conclusion
In this article, we covered Spring Boot and Logging. We studied what advantages Spring Boot offers when we compare it to Spring Web MVC project and its other modules.
Recent Stories
Top DiscoverSDK Experts
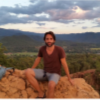
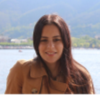
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
0 Comments
Your Comment