Java - Socket Programming
This article will guide you to Socket programming in Java. We will create a simple program to mock a Client Server architecture behavior using Sockets.
What are Sockets?
Directly from the docs, A socket is one endpoint of a two-way communication link between two programs running on the network. A socket is bound to a port number so that the TCP layer can identify the application that data is destined to be sent to.
An endpoint is a combination of an IP address and a port number. Every TCP connection can be uniquely identified by its two endpoints. That way you can have multiple connections between your host and the server.
A socket consists of three things:
- An IP address
- A transport protocol
- A port number
Usually, socket is confused with Ports but a port is a number between 1 and 65535 inclusive that signifies a logical gate in a device. Every connection between a client and server requires a unique socket.
For example:
- 1030 is a port.
- (10.1.1.2 , TCP , port 1030) is a socket.
Socket Programming
Sockets provide the communication mechanism between two computers using TCP protocol.
Basic steps of communication can be summarised as:
- Open a socket.
- Open an input stream and output stream to the socket.
- Read from and write to the stream according to the server's protocol.
- Close the streams.
- Close the socket.
Only step 3 differs from client to client, depending on the server. The other steps remain largely the same.
The java.net.Socket class indicates a socket, and the java.net.ServerSocket class gives a mechanism for the server program to listen for clients and establish connections with the clients.
To explain, steps which occur while establishing a TCP connection between two computers using sockets:
- The server instantiates a ServerSocket object, denoting which port number communication is to occur on.
- The server invokes the accept() method of the ServerSocket class. This method waits until a client connects to the server on the given port.
- After the server is waiting, a client instantiates a Socket object, specifying the server name and the port number to connect to.
- The constructor of the Socket class attempts to connect the client to the specified server and the port number. If communication is established, the client now has a Socket object capable of communicating with the server.
- On the server side, the accept() method returns a reference to a new socket on the server that is connected to the client's socket.
After the connections are established, communication can occur using I/O streams. Each socket has both an OutputStream and an InputStream. The client's OutputStream is connected to the server's InputStream, and the client's InputStream is connected to the server's OutputStream.
TCP is a two-way communication protocol, hence data can be sent across both streams at the same time. Following are the useful classes providing a complete set of methods to implement sockets.
Now, let’s dive into some code.
Socket Client Example
The following GreetingClient is a client program that connects to a server by using a socket and sends a greeting, and then waits for a response.
// File Name WelcomeClient.java
import java.net.*;
import java.io.*;
public class WelcomeClient {
public static void main(String [] args) {
String serverName = args[0];
int port = Integer.parseInt(args[1]);
try {
System.out.println("Connecting to " + serverName + " on port " + port);
Socket client = new Socket(serverName, port);
System.out.println("Just connected to " + client.getRemoteSocketAddress());
OutputStream outToServer = client.getOutputStream();
DataOutputStream out = new DataOutputStream(outToServer);
out.writeUTF("Hello from " + client.getLocalSocketAddress());
InputStream inFromServer = client.getInputStream();
DataInputStream in = new DataInputStream(inFromServer);
System.out.println("Server says " + in.readUTF());
client.close();
}catch(IOException e) {
e.printStackTrace();
}
}
}
Socket Server Example
The following WelcomeServer program is an example of a server application that uses the Socket class to listen for clients on a port number specified by a command-line argument −
// File Name WelcomeServer.java
import java.net.*;
import java.io.*;
public class WelcomeServer extends Thread {
private ServerSocket serverSocket;
public WelcomeServer(int port) throws IOException {
serverSocket = new ServerSocket(port);
serverSocket.setSoTimeout(10000);
}
public void run() {
while(true) {
try {
System.out.println("Waiting for client on port " +
serverSocket.getLocalPort() + "...");
Socket server = serverSocket.accept();
System.out.println("Just connected to " + server.getRemoteSocketAddress());
DataInputStream in = new DataInputStream(server.getInputStream());
System.out.println(in.readUTF());
DataOutputStream out = new DataOutputStream(server.getOutputStream());
out.writeUTF("Thank you for connecting to " + server.getLocalSocketAddress()
+ "\nGoodbye!");
server.close();
}catch(SocketTimeoutException s) {
System.out.println("Socket timed out!");
break;
}catch(IOException e) {
e.printStackTrace();
break;
}
}
}
public static void main(String [] args) {
int port = Integer.parseInt(args[0]);
try {
Thread t = new WelcomeServer(port);
t.start();
}catch(IOException e) {
e.printStackTrace();
}
}
}
Compile the client and the server and then start the server as follows −
$ java WelcomeServer 6066
Waiting for client on port 6066...
Check the client program as follows −
Output
$ java WelcomeClient localhost 6066
Connecting to localhost on port 6066
Just connected to localhost/127.0.0.1:6066
Server says Thank you for connecting to /127.0.0.1:6066
Goodbye!
Above simple example demonstrates on use of Sockets.
The java.net package provide support for 2 common network protocols, which are:
- TCP −Transmission Control Protocol, which allows for reliable communication between two applications. TCP is mainly used over the Internet Protocol, which is referred to as TCP/IP.
- UDP − UDP stands for User Datagram Protocol, a connection-less protocol that allows for packets of data to be transmitted between applications.
This article gives a good understanding on the following two topics:
- Socket Programming − This is the most widely used concept in Networking and it has been explained in a lot of detail in this tutorial.
- URL Processing − This would be covered separately.
Just to add, the UDP protocol provides a mode of network communication whereby applications send packets of data, called datagrams, to one another. A datagram is an independent, self-contained message sent over the network whose arrival, arrival time, and content are not guaranteed. The DatagramPacket and DatagramSocket classes in the java.net package implement system-independent datagram communication using UDP.
>>> A datagram arrival is not guaranteed.
Many firewalls and routers are configured not to allow UDP packets. If you have trouble connecting to a service outside your firewall, or if clients have trouble connecting to your service, ask your system administrator if UDP is permitted.
For more knowledge, roughly:
- the IP address is for routing between hosts on the network
- the port is for routing to the correct socket on the host
- It's actually possible to have multiple (usually child) processes all accepting on the same socket.
- However, each time one of the concurrent accept calls returns, it does so in only one process, each incoming connection's socket is unique to one instance of the server
- the socket is the object a process uses to talk to the OS about a particular connection, much like a file descriptor.
Conclusion
In this article, we studied about Socket programming in Java. We also created a simple program to mock a Client Server architecture behavior using Sockets.
Recent Stories
Top DiscoverSDK Experts
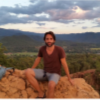
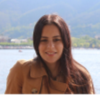
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment