Introduction to ES6 Classes
In the modern age of programming, Object Oriented Programming (OOP) is everywhere. In Object Oriented Programming, paradigm class plays the most fundamental role. Classes are blueprints for objects and they describe how the object will work and behave. JavaScript is a prototype based language and thus not fully object oriented. But JavaScript programmers tried in various ways to code in object oriented manner. It worked for various situations, but JavaScript programmers could not be fully satisfied with the hacking required to to code in an object oriented fashion. A class system was in demand for a long time. Finally in ES6, class was provided. ECMAScript 6 is still a prototype based language and classes are syntactic sugar for working with objects.
In this article I will show you how to work with classes and various aspects of it in ES6.
Prerequisites
To go along with this article you need to have basic knowledge in JavaScript. I have written some some introductory articles on JavaScript on this blog before. Refer to them to brush up on your JavaScript.
Though class is a feature of ECMAScript 6 it is not a necessary to have full knowledge of it—though it will help you a lot if you have prior knowledge in ECMAScript 6 or ES 6.
You will need the latest browsers or NodeJs installed on your system to work with ES6. If you want to run ES6 code on older versions of browsers or NodeJs you can use an ES6 compilers/transpilers, for example, Babel.
Preparing the Environment
In my articles/tutorials/guides I always encouraged you to use NodeJs to run JavaScript over browsers. You can use browsers if you like. I am going to use NodeJs to run our code shown in this article. So, it is expected that you have the latest NodeJs installed on your system.
Choose or create a folder to keep our script files. Create an empty file named es6classes.js in the chosen directory. Run the file with the following command to see if everything is alright.
nodejs es6classes.js
Creating a Class
Let's start our coding with ES6 classes by creating a class. A class has a name like functions have. The name of the class should be preceded with the keyword class. The body of the class will be surrounded in a pair of curly braces. Let's create a class with the name Human:
class Human{}
That is a bare minimum class and it can do nothing at the moment. It is a class with just its name. The body is empty.
Creating an Object from a Class
Though we have an empty class, still it is a class and we can create objects from it. To create an object from a class you need to call the class like a function (a pair of parentheses preceded by the class name) and precede that with the keyword new.
var o1 = new Human();
console.log(o1)
Outputs:
Human {}
Note: Class instantiation is the proper term to refer to class creation in programming.
Creating a Constructor
A constructor is a function (a method to be more accurate) that is called during the creation of an object from a class. This function can accept arguments. The arguments passed to the class during their creation are passed to the constructor. The constructor is not directly called, instead it is called automatically during the class instantiation. The constructor function of a class has a fixed name constructor.
Our blueprint (the class) for objects has no extra information or functionality attached to it at this moment. We want to provide the Human object a name. Let's pass that name to the object with the help of a constructor.
class Human{
constructor(name){
this.name = name
}
}
var o1 = new Human("Sabuj");
console.log(o1.name)
Outputs:
Sabuj
What is 'this':
this is a magic variable automatically available inside class methods that refers to the current object. In our case we have an object o1. When the constructor was called it got a reference to the current object that we are referring with variable o1. So, constructor did set the name we passed during class instantiation. If we create another object the function will refer to that object from inside class with the help of the this variable.
To illustrate the use of this let's try to set the name after creation.
class Human{
constructor(name){
this.name = name
}
setName(n){
this.name = n
}
}
var o1 = new Human("Sabuj");
console.log("Name: "+o1.name);
o1.setName("Unknown Person");
console.log("Name: "+o1.name)
Outputs:
Name: Sabuj
Name: Unknown Person
Class Expressions
Class expression is another way of declaring or creating a class. It is more like a declaring an anonymous function and assigning that with a variable. See the example below:
var HumanClass = class{
constructor(name){
this.name = name
}
setName(n){
this.name = n
}
}
var o1 = new HumanClass("Sabuj");
console.log("Name: "+o1.name);
o1.setName("Unknown Person");
console.log("Name: "+o1.name)
The class can be given a name as we did before with class expression:
var HumanClass = class Human{
constructor(name){
this.name = name
}
setName(n){
this.name = n
}
}
Inheriting a Class
In object oriented programming we can reuse a class for creating a new one. The attributes and methods are reused in the child class. Let's say that we have a class called Animal and we want to create a class Dog from it and use attributes and methods defined in Animal from objects of Dog. To inherit from a class the keyword extends is used after the class name and the class name of that is being inherited.
class Animal{
constructor(name, type){
this.name = name;
this.type = type;
}
myInfo(){
console.log("My name is: " + this.name + " and I am of type: " + this.type);
}
}
class Dog extends Animal{
bark(){
console.log("Woof");
}
}
var d1 = new Dog("Dog 1", "Hybrid");
d1.myInfo();
d1.bark();
Outputs:
My name is: Dog 1 and I am of type: Hybrid
Woof
We called the myInfo() method on the Dog object, but it was not defined in the Dog class, instead it was defined in Animal. It worked fine. Again bark() was defined in the Dog class.
Conclusion
Years of unsatisfied desires were satisfied to a great degree in ES 6 for JavaScript programmers. It is a great addition to the language. To get the full benefit of it try all your object oriented concepts from other object oriented languages in JavaScript with classes, and see what fun you can get out of it.
Next article: | Advancing into ES6 Classes |
About the Author
My name is Md. Sabuj Sarker. I am a Software Engineer, Trainer and Writer. I have over 10 years of experience in software development, web design and development, training, writing and some other cool stuff including few years of experience in mobile application development. I am also an open source contributor. Visit my github repository with username SabujXi.
Recent Stories
Top DiscoverSDK Experts
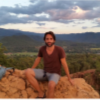
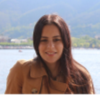
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment