Hibernate O/R Mapping using Set and HashSet
In this article, we will study Hibernate O/R collection mappings and learn how to map a Set and HashSet collection to entities stored in a database.
Hibernate is a complete solution for the persistence module in a Java project. It handles the application interaction with the database, leaving the developer free to concentrate more on business logic and solving complex problems.
So far, we have seen basic O/R mapping using hibernate but there are more important mappings like inserting Java Collections in Database.
Collection Mapping
If an entity or class has multiple values for a particular property or variable, then we can map those values using one of the collection interfaces available in Java. Hibernate can persist instances of java.util.Map, java.util.Set, java.util.SortedMap, java.util.SortedSet, java.util.List, and any array of persistent entities or values.
The collection we want to use depends on the behavior we want to introduce like if we want to store only unique values, we will need to use a Set collection etc.
Let’s look at each of these with examples here.
java.util.Set
A Set is a Java collection that does not contain any duplicate elements. More formally, sets contain no pair of elements b1 and b2 such that b1.equals(b2), and at most one null element. So objects to be added to a set must implement both the equals() and hashCode() methods so that Java can determine whether any two elements/objects are identical or not.
A Set is mapped with a <set> element in the mapping table and initialized with java.util.HashSet. We can use Set collection in our class when we need to keep only unique elements in our collection.
Defining RDBMS table
We start by defining our RDBMS table. We will again use our Student table here:
create table STUDENT (
id INT NOT NULL auto_increment,
first_name VARCHAR(20) default NULL,
last_name VARCHAR(20) default NULL,
grade DECIMAL(3,1) default NULL,
PRIMARY KEY (id)
);
Now, let us assume that each student can have multiple certifications involved. So we will store certificate related information in a separate table which has the following schema:
create table CERTIFICATE (
id INT NOT NULL auto_increment,
certificate_name VARCHAR(30) default NULL,
student_id INT default NULL,
PRIMARY KEY (id)
);
POJO for Entities
Let us have a look at our Student entity POJO once again:
public class Student {
private int id;
private String firstName;
private String lastName;
private float grade;
private Set certificates;
public Student() {}
public Student(String fname, String lname, float grade) {
this.firstName = fname;
this.lastName = lname;
this.grade = grade;
}
public int getId() {
return id;
}
public void setId( int id ) {
this.id = id;
}
public String getFirstName() {
return firstName;
}
public void setFirstName( String first_name ) {
this.firstName = first_name;
}
public String getLastName() {
return lastName;
}
public void setLastName( String last_name ) {
this.lastName = last_name;
}
public int getGrade() {
return grade;
}
public void setGrade( float grade ) {
this.grade = grade;
}
public Set getCertificates() {
return certificates;
}
public void setCertificates( Set certificates ) {
this.certificates = certificates;
}
}
Next, we define our second POJO class for the CERTIFICATE table so that certificate objects can be saved and read from the CERTIFICATE table. This class should implement both the equals() and hashCode() methods so that Java can determine if two elements/objects are equal.
public class Certificate {
private int id;
private String name;
public Certificate() {}
public Certificate(String name) {
this.name = name;
}
public int getId() {
return id;
}
public void setId( int id ) {
this.id = id;
}
public String getName() {
return name;
}
public void setName( String name ) {
this.name = name;
}
public boolean equals(Object obj) {
if (obj == null) return false;
if (!this.getClass().equals(obj.getClass())) return false;
Certificate obj2 = (Certificate)obj;
if((this.id == obj2.getId()) && (this.name.equals(obj2.getName())))
{
return true;
}
return false;
}
public int hashCode() {
int tmp = 0;
tmp = ( id + name ).hashCode();
return tmp;
}
}
Now, as our POJO classes are ready, we define our Hibernate mapping file.
Hibernate Mapping file
In this file, the <set> element will be used to define the rule for Set collection used.
<?xml version="1.0" encoding="utf-8"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name="Student" table="STUDENT">
<meta attribute="class-description">
This class contains the student detail.
</meta>
<id name="id" type="int" column="id">
<generator class="native"/>
</id>
<set name="certificates" cascade="all">
<key column="student_id"/>
<one-to-many class="Certificate"/>
</set>
<property name="firstName" column="first_name" type="string"/>
<property name="lastName" column="last_name" type="string"/>
<property name="grade" column="grade" type="float"/>
</class>
<class name="Certificate" table="CERTIFICATE">
<meta attribute="class-description">
This class contains the certificate records.
</meta>
<id name="id" type="int" column="id">
<generator class="native"/>
</id>
<property name="name" column="certificate_name" type="string"/>
</class>
</hibernate-mapping>
The <set> element is new in this file and has been introduced to set the relationship between Certificate and Student classes. We used the cascade attribute in the <set> element to tell Hibernate to persist the Certificate objects at the same time as the Student objects. The name attribute is set to the defined Set variable in the parent class, in our case it is certificates. For each set variable, we need to define a separate set element in the mapping file.
The <one-to-many> element indicates that one Student object relates to many Certificate objects and, as such, the Certificate object must have a Student parent object associated with it. We can use either <one-to-one>, <many-to-one> or <many-to-many> elements based on our requirement.
Application class
Finally, we will create our application class with the main() method to run the application. We will use this application to save a few Student's records along with their certificates and then we will apply CRUD operations on those records.
HashSet set1 = new HashSet();
set1.add(new Certificate("MCA"));
set1.add(new Certificate("MBA"));
set1.add(new Certificate("PMP"));
/* Add student records in the database */
Integer studentID1 =
ManageStudent.addStudent("Liran", "Heim", 4000, set1);
Clearly, it was simple to create Set elements and store it in the database.
Now, if we check our database, we will have following results in our Student and Certificate tables.
mysql> select * from student;
+----+------------+-----------+--------+
| id | first_name | last_name | grade |
+----+------------+-----------+--------+
| 1 | Liran | Heim | 5.1 |
+----+------------+-----------+--------+
1 row in set (0.00 sec)
mysql> select * from certificate;
+----+------------------+-------------+
| id | certificate_name | student_id |
+----+------------------+-------------+
| 1 | MBA | 1 |
| 2 | PMP | 1 |
| 3 | MCA | 1 |
+----+------------------+-------------+
3 rows in set (0.00 sec)
Conclusion
In this article, we studied Hibernate O/R mappings using Set & HashSet and built the last example once again but this time, using Hibernate collection Set mappings.
In a later article, we will build upon these concepts to learn about Hibernate collections using SortedSet mappings.
Recent Stories
Top DiscoverSDK Experts
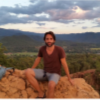
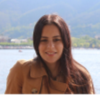
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment