Hibernate - CRUD example
In this article, we’ll be learning about Hibernate mapping types and implementing a CRUD application by specifying each step on how an application in Hibernate can be implemented.
Hibernate is a complete solution for the persistence module in a Java project. It handles the application interaction with the database, leaving the developer free to concentrate more on business logic and solving complex problems.
Mapping Types
In the last article, we saw an example of mapping Java fields to RDBMS table attributes. The types declared and used in the mapping files are not Java data types and are not SQL database types either. These types are called Hibernate mapping types, which can translate from Java to SQL data types and vice versa.
Here, we will list all the basic, date and time, large object, and various other built-in mapping types.
Primitive types:
Mapping type |
Java type |
ANSI SQL Type |
integer |
int or java.lang.Integer |
INTEGER |
long |
long or java.lang.Long |
BIGINT |
short |
short or java.lang.Short |
SMALLINT |
float |
float or java.lang.Float |
FLOAT |
double |
double or java.lang.Double |
DOUBLE |
big_decimal |
java.math.BigDecimal |
NUMERIC |
character |
java.lang.String |
CHAR(1) |
string |
java.lang.String |
VARCHAR |
byte |
byte or java.lang.Byte |
TINYINT |
boolean |
boolean or java.lang.Boolean |
BIT |
yes/no |
boolean or java.lang.Boolean |
CHAR(1) ('Y' or 'N') |
true/false |
boolean or java.lang.Boolean |
CHAR(1) ('T' or 'F') |
Binary and large object types:
Mapping type |
Java type |
ANSI SQL Type |
binary |
byte[] |
VARBINARY (or BLOB) |
text |
java.lang.String |
CLOB |
serializable |
any Java class that implements java.io.Serializable |
VARBINARY (or BLOB) |
clob |
java.sql.Clob |
CLOB |
blob |
java.sql.Blob |
BLOB |
Date and time types:
Mapping type |
Java type |
ANSI SQL Type |
date |
java.util.Date or java.sql.Date |
DATE |
time |
java.util.Date or java.sql.Time |
TIME |
timestamp |
java.util.Date or java.sql.Timestamp |
TIMESTAMP |
calendar |
java.util.Calendar |
TIMESTAMP |
calendar_date |
java.util.Calendar |
DATE |
JDK-related types:
Mapping type |
Java type |
ANSI SQL Type |
class |
java.lang.Class |
VARCHAR |
locale |
java.util.Locale |
VARCHAR |
timezone |
java.util.TimeZone |
VARCHAR |
currency |
java.util.Currency |
VARCHAR |
Example
Now let’s build upon all the concepts we have read about ORM and Hibernate by making a very simple CRUD command line app.
First step to define any application is to define our entity or persistent classes with relevant fields. Let us consider our Student class with getXXX and setXXX methods to make it a JavaBeans compliant class.
A POJO (Plain Old Java Object) is a Java object that doesn't extend or implement any classes and interfaces respectively required by the EJB framework. All normal Java objects are POJO.
Let us have a look at our Student entity POJO once again:
public class Student {
private int id;
private String firstName;
private String lastName;
private float grade;
public Student() {}
public Student(String fname, String lname, float grade) {
this.firstName = fname;
this.lastName = lname;
this.grade = grade;
}
public int getId() {
return id;
}
public void setId( int id ) {
this.id = id;
}
public String getFirstName() {
return firstName;
}
public void setFirstName( String first_name ) {
this.firstName = first_name;
}
public String getLastName() {
return lastName;
}
public void setLastName( String last_name ) {
this.lastName = last_name;
}
public int getGrade() {
return grade;
}
public void setGrade( float grade ) {
this.grade = grade;
}
}
If the above defined persistent classes needs to be saved to the database, let us first consider an RDBMS table as:
create table STUDENT (
id INT NOT NULL auto_increment,
first_name VARCHAR(20) default NULL,
last_name VARCHAR(20) default NULL,
grade DECIMAL(3,1) default NULL,
PRIMARY KEY (id)
);
Let us define our mapping document based on the POJO we defined earlier and the RDBMS table we just wrote:
<?xml version="1.0" encoding="utf-8"?>
<!DOCTYPE hibernate-mapping PUBLIC
"-//Hibernate/Hibernate Mapping DTD//EN"
"http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd">
<hibernate-mapping>
<class name="Student" table="STUDENT">
<meta attribute="class-description">
This class contains the student detail.
</meta>
<id name="id" type="int" column="id">
<generator class="native"/>
</id>
<property name="firstName" column="first_name" type="string"/>
<property name="lastName" column="last_name" type="string"/>
<property name="grade" column="grade" type="float"/>
</class>
</hibernate-mapping>
It is important to remember naming the file as <classname>.hbm.xml. I saved this file as Student.hbm.xml.
Let’s quickly understand these attributes once again here:
- The mapping document is an XML document having <hibernate-mapping> as the root element which contains all the <class> elements.
- The <class> elements are used to define specific mappings from a Java classes to the database tables.
- The <meta> element is optional element and can be used to create the class description.
- The <id> element maps the unique ID identifier in class to the primary key of the DB table.
- The <generator> element within the id element is used to automatically generate the primary key values.
- The <property> element is used to map a Java class property to a column in the database table. The type attribute holds the hibernate mapping type, this mapping types will convert from Java to SQL data type.
The Application main() class
Finally, we will create our application class with the main() method to run the application. We will use this application to save few Student's records and then we will apply CRUD operations on those records.
import java.util.List;
import java.util.Date;
import java.util.Iterator;
import org.hibernate.HibernateException;
import org.hibernate.Session;
import org.hibernate.Transaction;
import org.hibernate.SessionFactory;
import org.hibernate.cfg.Configuration;
public class ManageStudent {
private static SessionFactory factory;
public static void main(String[] args) {
try{
factory = new Configuration().configure().buildSessionFactory();
}catch (Throwable ex) {
System.err.println("Failed to create sessionFactory object in Student class." + ex);
throw new ExceptionInInitializerError(ex);
}
ManageStudent MS = new ManageStudent();
/* Add few student records in database */
Integer studentID1 = MS.addStudent("Shubham", "Aggarwal", 9);
Integer studentID2 = MS.addStudent("Liran", "Heim", 9.5);
Integer studentID3 = MS.addStudent("Zach", "Little", 4);
/* List down all the students */
ME.listStudents();
/* Update student's records */
ME.updateStudent(studentID1, 9.1);
/* Delete an student from the database */
ME.deleteStudent(studentID3);
/* List down new list of the students */
ME.listStudents();
}
/* Method to CREATE an student in the database */
public Integer addStudent(String fname, String lname, float grade){
Session session = factory.openSession();
Transaction tx = null;
Integer studentID = null;
try{
tx = session.beginTransaction();
Student student = new Student(fname, lname, grade);
studentID = (Integer) session.save(student);
tx.commit();
}catch (HibernateException e) {
if (tx!=null) tx.rollback();
e.printStackTrace();
}finally {
session.close();
}
return studentID;
}
/* Method to READ all the students */
public void listStudents( ){
Session session = factory.openSession();
Transaction tx = null;
try{
tx = session.beginTransaction();
List students = session.createQuery("FROM Student").list();
for (Iterator iterator =
students.iterator(); iterator.hasNext();){
Students student = (Student) iterator.next();
System.out.print("First Name: " + student.getFirstName());
System.out.print(" Last Name: " + student.getLastName());
System.out.println(" Grade: " + student.getGrade());
}
tx.commit();
}catch (HibernateException e) {
if (tx!=null) tx.rollback();
e.printStackTrace();
}finally {
session.close();
}
}
/* Method to UPDATE grade for a student */
public void updateStudent(Integer studentID, float grade ){
Session session = factory.openSession();
Transaction tx = null;
try{
tx = session.beginTransaction();
Student student =
(Student) session.get(Student.class, studentID);
student.setGrade( grade );
session.update(student);
tx.commit();
}catch (HibernateException e) {
if (tx!=null) tx.rollback();
e.printStackTrace();
}finally {
session.close();
}
}
/* Method to DELETE an student from the records */
public void deleteStudent(Integer studentID){
Session session = factory.openSession();
Transaction tx = null;
try{
tx = session.beginTransaction();
Student student =
(Student)session.get(Student.class, studentID);
session.delete(student);
tx.commit();
}catch (HibernateException e) {
if (tx!=null) tx.rollback();
e.printStackTrace();
}finally {
session.close();
}
}
}
Now, to compile and run the above mentioned application. Make sure you have set PATH and CLASSPATH appropriately before proceeding for the compilation and execution.
- Create hibernate.cfg.xml configuration file as explained above.
- Create Student.hbm.xml mapping file as shown above.
- Create Student.java source file as shown above and compile it.
- Create ManageStudent.java source file as shown above and compile it.
- Execute ManageStudent binary to run the program.
Conclusion
In this article, we studied Hibernate mapping types and also learned how to define Hibernate persistent classes by defining appropriate mapping documents and making a simple app to perform simple CRUD operations on this entity.
In a later article, we will build upon these concepts to learn about O/R mappings and study Hibernate annotations.
Recent Stories
Top DiscoverSDK Experts
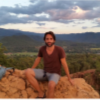
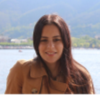
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment