Getting Started with Spring Boot
Spring Boot comes with a lot of starters which help you get up and running quickly, making you less dependent on, well, dependencies!
This tutorial will provide you with an introduction of Spring Initializer, available components provided by Spring Boot, and will also show you how to make a quick Boot project.
In this chapter, we will learn about the following topics:
- Using a Spring Boot template and starter
- Creating a simple application
- Launching an application using Gradle
- Setting up a database connection
- Setting up a data repository service
Using Spring Initializer
We will start our tutorial by creating a basic simple project skeleton and Spring Boot will help us in this:
- Visit Spring Initializer.
- Fill out a simple form with the details about the project.
- Clicking on “Generate Project” will provide a preconfigured project structure for us to begin with.
With Spring Initializer, we can see the Project Dependencies section, where we can select the kind of functionalities that our app can perform: will it connect to a database, will it have a web interface, do we plan to integrate with any of the social network for authentication, provide JUnit tests capabilities, and so on. By selecting the desired technologies, the appropriate starter libraries will be added automatically to the dependency list of our pre-generated project template.
What is a Spring Boot Starter?
Spring Boot aims to simplify the process of getting started with an app as quickly as possible. Spring Boot starters are bootstrap libraries that contain a collection of all the relevant transitive dependencies that are needed to start a particular functionality.
This means that if a library depends on another library, Spring Boot starter will bring all the required dependencies automatically.
For example, spring-boot-starter-test starter will automatically bring spring-test, spring-boot, junit, mockito, and hamcrest-library. Isn’t that awesome?
With more than 50 starters provided and with the ongoing community support increasing the list, it is very likely that should we find ourselves with the need to integrate with a fairly common or popular framework, there is already a starter out there that we can consume.
Creating a simple app
Now, let us get started with the project and what starters we selected with the Spring Initializer.
The application that we are going to create is a student management system. It will keep a record of student that were enrolled, who were the teachers, the reviewers, schools, and so forth. We will name our project DiscoverStudent, and apply the following steps:
- Use the default proposed Group name: com.discoverstudent.
- Enter discoverstudent for an Artifact field.
- Provide DiscoverStudent as a Name for the application.
- Specify com.discoversdk as our Package Name.
- Choose Gradle Project.
- Select Jar as Packaging.
- Use Java Version as 1.8.
- Use Spring Boot Version as 1.5.3.
- Select the H2, JDBC, and JPA starters from the Project Dependencies selection so that we can get the needed artifacts in our build file in order to connect to an H2 database
- Click Generate Project to download the project archive.
When we hit the “Generate Project” button, the project will begin to download. In the project, we can see the “build.gradle” file with following code,
dependencies {
compile("org.springframework.boot:spring-boot-starter-data-jpa")
compile("org.springframework.boot:spring-boot-starter-jdbc")
runtime("com.h2database:h2")
testCompile("org.springframework.boot:spring-boot-starter-test")
}
>>> Which language does the Gradle build system use?Time for an exercise
While making the selection, we added the following starters:
- org.springframework.boot:spring-boot-starter-data-jpa pulls in the JPA dependency.
- org.springframework.boot:spring-boot-starter-jdbc pulls in the JDBC supporting libraries.
- com.h2database:h2 is a particular type of database implementation, namely H2 (an in-memory database).
Database is of prime importance here. Connection to the database automatically configured here when the app runs.
Let's start by looking at our newly created application template, specifically at DiscoverStudentApplication.java located in the src/main/java/org/test/discoverstudent directory in the root of the project, as follows:
package com.discoversdk.discoverstudent;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DiscoverstudentApplication {
public static void main(String[] args) {
SpringApplication.run(DiscoverstudentApplication.class, args);
}
}
This is our entire and fully runnable application. Yes that’s right, our Spring Boot app can run with a single class.
The @SpringBootApplication annotation does many things. It also includes many annotations inside it. Let’s look at them next:
- @Configuration tells Spring that the annotated class contains Spring configuration definitions such as the @Bean, @Component, and @Service declarations, and others.
- @ComponentScan tells Spring that we want to scan our application packages, starting from the package of our annotated class as a default package root.
- @EnableAutoConfiguration is a part of the Spring Boot annotation, which is a meta-annotation on its own. It imports the EnableAutoConfigurationImportSelector and AutoConfigurationPackages. These are registrar classes that effectively instruct Spring to automatically configure the conditional beans depending on the classes available in the classpath.
Just for demonstration, here is the file structure of our app:
Launching the app using Gradle
Now that we know our Spring Boot app is ready, let us run it.
Open terminal and change the directory to the root of the extracted project directory and execute the following command from the command line:
$ ./gradlew clean bootRun
This will build and run the application and produce the following output:
Downloading https://services.gradle.org/distributions/gradle-3.4.1-all.zip
...
Unzipping /Users/DiscoverSDK/.gradle/wrapper/dists/gradle-3.4.1-all/c3ib5obfnqr0no9szq6qc17do/gradle-3.4.1-all.zip to /Users/DiscoverSDK/.gradle/wrapper/dists/gradle-3.4.1-all/c3ib5obfnqr0no9szq6qc17do
Set executable permissions for: /Users/DiscoverSDK/.gradle/wrapper/dists/gradle-3.4.1-all/c3ib5obfnqr0no9szq6qc17do/gradle-3.4.1/bin/gradle
Starting a Gradle Daemon (subsequent builds will be faster)
:clean UP-TO-DATE
:compileJava
:processResources
:classes
:findMainClass
:bootRun
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v1.5.3.RELEASE)
2017-05-14 07:53:12.304 INFO 2971 --- [ main] c.d.d.DiscoverstudentApplication : Starting DiscoverstudentApplication on DiscoverSDK.local with PID 2971 (/Users/DiscoverSDK/discoverstudent/build/classes/main started by DiscoverSDK in /Users/DiscoverSDK/discoverstudent)
2017-05-14 07:53:12.307 INFO 2971 --- [ main] c.d.d.DiscoverstudentApplication : No active profile set, falling back to default profiles: default
2017-05-14 07:53:12.413 INFO 2971 --- [ main] s.c.a.AnnotationConfigApplicationContext : Refreshing org.springframework.context.annotation.AnnotationConfigApplicationContext@51b279c9: startup date [Sun May 14 07:53:12 IST 2017]; root of context hierarchy
2017-05-14 07:53:13.976 INFO 2971 --- [ main] j.LocalContainerEntityManagerFactoryBean : Building JPA container EntityManagerFactory for persistence unit 'default'
2017-05-14 07:53:14.011 INFO 2971 --- [ main] o.hibernate.jpa.internal.util.LogHelper : HHH000204: Processing PersistenceUnitInfo [
name: default
...]
2017-05-14 07:53:14.104 INFO 2971 --- [ main] org.hibernate.Version : HHH000412: Hibernate Core {5.0.12.Final}
2017-05-14 07:53:14.109 INFO 2971 --- [ main] org.hibernate.cfg.Environment : HHH000206: hibernate.properties not found
2017-05-14 07:53:14.111 INFO 2971 --- [ main] org.hibernate.cfg.Environment : HHH000021: Bytecode provider name : javassist
2017-05-14 07:53:14.288 INFO 2971 --- [ main] o.hibernate.annotations.common.Version : HCANN000001: Hibernate Commons Annotations {5.0.1.Final}
2017-05-14 07:53:14.461 INFO 2971 --- [ main] org.hibernate.dialect.Dialect : HHH000400: Using dialect: org.hibernate.dialect.H2Dialect
2017-05-14 07:53:14.801 INFO 2971 --- [ main] org.hibernate.tool.hbm2ddl.SchemaExport : HHH000227: Running hbm2ddl schema export
2017-05-14 07:53:14.812 INFO 2971 --- [ main] org.hibernate.tool.hbm2ddl.SchemaExport : HHH000230: Schema export complete
2017-05-14 07:53:14.867 INFO 2971 --- [ main] j.LocalContainerEntityManagerFactoryBean : Initialized JPA EntityManagerFactory for persistence unit 'default'
2017-05-14 07:53:15.269 INFO 2971 --- [ main] o.s.j.e.a.AnnotationMBeanExporter : Registering beans for JMX exposure on startup
2017-05-14 07:53:15.296 INFO 2971 --- [ main] c.d.d.DiscoverstudentApplication : Started DiscoverstudentApplication in 13.454 seconds (JVM running for 14.045)
2017-05-14 07:53:15.316 INFO 2971 --- [ Thread-3] s.c.a.AnnotationConfigApplicationContext : Closing org.springframework.context.annotation.AnnotationConfigApplicationContext@51b279c9: startup date [Sun May 14 07:53:12 IST 2017]; root of context hierarchy
2017-05-14 07:53:15.319 INFO 2971 --- [ Thread-3] o.s.j.e.a.AnnotationMBeanExporter : Unregistering JMX-exposed beans on shutdown
2017-05-14 07:53:15.320 INFO 2971 --- [ Thread-3] j.LocalContainerEntityManagerFactoryBean : Closing JPA EntityManagerFactory for persistence unit 'default'
2017-05-14 07:53:15.320 INFO 2971 --- [ Thread-3] org.hibernate.tool.hbm2ddl.SchemaExport : HHH000227: Running hbm2ddl schema export
2017-05-14 07:53:15.321 INFO 2971 --- [ Thread-3] org.hibernate.tool.hbm2ddl.SchemaExport : HHH000230: Schema export complete
BUILD SUCCESSFUL
Total time: 58.317 secs
Setting Up Database connection
Let's change the defaults in order to create an embedded H2 database that will not store data in-memory, but rather use a file to persist the data in between application restarts.
- Open the file named application.properties under the src/main/resources directory from the root of our project and add the following content:
spring.datasource.url = jdbc:h2:~/test;DB_CLOSE_DELAY=-1;DB_CLOSE_ON_EXIT=FALSE
spring.datasource.username = username
spring.datasource.password = password
- Start the application by executing ./gradlew clean bootRun from the command line.
This configures the H2 database but H2 is hardly used in any production based apps. You will want to configure MySQL, most probably, with a production grade application. Here are the properties required to connect to a MySQL based database:
spring.datasource.driver-class-name: com.mysql.jdbc.Driver
spring.datasource.url: jdbc:mysql://localhost:3306/discoversdk
spring.datasource.username: root
spring.datasource.password: password
Creating Data Repositories
Now, let’s move forward to saving data in our database, irrespective of it being H2 or MySQL.
- Create a new package folder named entity under the src/main/java/com/discoversdk directory from the root of our project.
- Add a class called Student.java with following content:
@Entity
public class Student {
@Id
@GeneratedValue
private Long id;
private String name;
//setters and getters
}
That’s not much and it should get us started.
@Entity indicates that the annotated class should be mapped to a database table.
- Finally, we create our repository.
@Repository
public interface StudentRepository extends JpaRepository<Student, Long> {
Student findByName(String name);
}
@Repository indicates that the interface is intended to provide you with the access and manipulation of data for a database.
As you have probably noticed, we didn't write a single line of SQL or even mentioned anything about database connections, building queries, or things like that.
The JpaRepository constructs SQL queries based on interface methods we create which should follow a particular convention.
Now, we can modify our DiscoverstudentApplication class to perform simple CRUD:
package com.discoversdk.discoverstudent;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class DiscoverstudentApplication {
@Autowired
private StudentRepository repository;
public static void main(String[] args) {
SpringApplication.run(DiscoverstudentApplication.class, args);
Student student = new Student();
student.setName(“Liran”);
repository.save(student);
}
}
As StudentRepository extends from JpaRepository, it has some built in methods to perform CRUD operations on an entity. This is an example.
Conclusion
We will conclude our journey for a simple Spring app with database connection here. We discovered a lot of Spring Boot powers here. We will continue our journey to follow some Spring Web apps in the coming articles.
Recent Stories
Top DiscoverSDK Experts
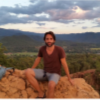
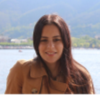
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment