Getting Started With Flask
Flask is a web application development framework written in Python. It is not a full stack web application development framework. It is a micro-framework based on Werkzeug.
In this guide I will show you how to setup your environment for development, how to install Flask and a simple web application that will show some text on your browser.
Before You Begin
This guide is for Flask beginners and for the Python beginners. So, make sure that:
- You have good experience in coding in Python
- You understand the concept of decorators. To learn about the decorators, you can read our series on that topic.
- You have Python 3 installed on your system
- You are familiar with working with command line available on your system
Preparing Your Working Environment
To keep the Python script files you write for Flask, choose or create a directory. Create a file named flask_app.py in that directory. Start the command line in the chosen directory or cd into it.
You are free to choose any plain text editor, code editor, or Python supported IDE for writing and editing Python code shown in this guide. PyCharm can be a better choice for you, but it all depends on your taste.
Setting a Virtual Environment
When developing a Python application that has external library dependency or needs a different version of Python among the various versions installed on your system, you should create a virtual environment.
To install a virtual environment, run the following command from the command line
$ pip3 install virtualenv
Now, create a virtual environment in the current directory or anywhere you like. You need to provide a name for the new virtual environment. I am going to call it venv, you are free to name it anything else.
After creating the virtual environment you need to activate it. If you are on a Windows machine you can run the following command to activate it.
venv\Scripts\activate
You will see (venv) on the left side of the prompt of your command line, which indicates that you are inside a virtual environment.
If you are on a UNIX based machine then you can run the following command to do the same:
$ source venv/bin/activate
When you need to go back to the original/default environment provided by your system, just run the following command:
$ deactivate
Installing Flask
After creating and activating your virtual environment run the following command to install Flask in the environment:
pip install flask
It will install all the dependency along with Flask for you.
Writing the First Web Application with Flask
Now, open the flask_app.py file in your favorite editor. The first thing we need to do to import Flask. Here's how:
import flask
You need to create an app by instantiating the Flask class from the flask module. Also pass the name of the current module to it's constructor.
import flask
app = flask.Flask(__name__)
The app is the single source of truth in Flask environment. You need to refer to this in many operations in you Flask application.
Now define a pure Python function that will be responsible for serving requests to the browser when someone visits your web application through their browser.
def home():
return "Welcome to my home page that I made available with the help of flask."
But, wait! How Flask will know that this is your home page? Here's where routing comes into play. With the help of route we will tell Flask which function to run and send the return value from the function invocation when someone visits our web application. Route can be easily defined with the help of the route() decorator present on the application object. We will apply this decorator on our home() function. As an argument to the decorator we will provide the url path. What is the url path for home? Yes, you guessed it right, it is '/'. So, let's do this.
import flask
app = flask.Flask(__name__)
@app.route("/")
def home():
return "Welcome to my home page that I made available with the help of flask."
That's it. Now, go to your browser and you will see what you don't want. Wait, no! I was just joking. You need to start the application with the help of Flask.
Flask will start a development server on your request (by invoking some command on command line) and your application will be available through localhost with certain port number.
You may write your starting Flask python script with any name. How Flask will know which one is the right one? You need to tell that to Flask. You need to set an environment variable called FLASK_APP with the python file name that you want as the entry point and then run the following command.
flask run
So, how to set the environment variable? If you are on an UNIX machine just run the following command on the command line.
$ FLASK_APP=flask_app.py
And if you are on an windows machine, run the following command:
set FLASK_APP=flask_app.py
Now run the application with the aforementioned flask run command.
It will start your application on your localhost at the port number 5000 if that port number is not already consumed by any other application.
Go to you browser and browse to http://127.0.0.1:5000/. There you will see "Welcome to my home page that I made available with the help of flask." Yahoo! Our application is up and running.
The Flask development server will show something like this on the command line:
* Serving Flask app "flask_app"
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
127.0.0.1 - - [30/Nov/2017 22:36:54] "GET / HTTP/1.1" 200 -
127.0.0.1 - - [30/Nov/2017 22:36:54] "GET /favicon.ico HTTP/1.1" 404 -
Conclusion
Flask is an awesome web framework, but unlike Django it is not a full stack framework. Also it is not as complicated as Django for the same reason. If you need a good architecture in the Flask environment you will need to make it yourself. For small applications however this is not necessary. Keep coding your web application in Flask and check back for more Flask guides soon.
Recent Stories
Top DiscoverSDK Experts
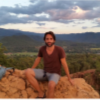
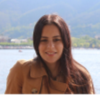
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment