First Steps in Django for Mac
Django is an awesome open-source MTV (Model Template View) framework written in Python for building web applications. The great thing about Django is that it supports common tasks related to web apps straight out of the box.
Django from 10,000 feet
Usually, web applications send data to the server to process, apply some logic, fetch records from a database, and finally provide results which are rendered into HTML (or just JSON in case of pure web services).
For example, with following URL:
https://docs.google.com/document/d/1XdbAA4-jJsIts54kfO-MWbxtUQjLjG_LyDF1XFKXXXX/edit#
The above URL contains certain document ID. When a Django app receives the above URL, it extracts that data out, looks into the the database for the document data and returns it to Google Docs to process the correct HTML with the correct data.
Django MTV Framework
Apart from being an MVC framework, Django follows MTV style. As you get more familiar with Django over time, you’ll come to understand the differences (and similarities) between MVC and MTV style.
Both of these styles of frameworks focuses on one thing: keeping validation, processing, and rendering in separate places called components or modules. With Django, these are called the model, template, and view (MTV).
The view can be considered as a controller and the template as view, so MVC becomes MTV in Django. This might sounds confusing but stick with me on this, everything will be clear once we get our hands dirty with an exercise.
Setting Up Django
To start setting up Django, you first need Python. In this lesson, we will cover installation steps only for Mac. In another lesson, we will cover the installation steps for other Operating Systems as well.
Let us follow a set of simple steps to get our Development environment ready.
- The easiest way to get started with Python is pip. Let’s install it:
The command above will ask for the mac password. Once installation is complete, the following trace can be seen:
- Next, to keep everything related to Development separate, let’s make a directory with command:
mkdir development
A typical location for saving your code is in a folder/directory called "Development" so you can keep it organized and in one place.
- Next, install virtualenv with pip:
What is Virtualenv?
Virtualenv is a tool to create isolated Python environments.
Let's say you're working in 2 different projects, A and B. Project A is a web project and the team is using the following packages:
- Python 2.8.x
- Django 1.6.x
Project B is also a web project but your team is using:
- Python 2.7.x
- Django 1.4.x
If the machine that you're working doesn't have any version of Django, what should you do? Install Django 1.4? Django 1.6? If you install django 1.4 globally, would it be easy to point to Django 1.6 to work in project A?
Virtualenv is your solution! You can create 2 different virtualenv's, one for project A and another for project B. Now, when you need to work in project A, just activate the virtualenv for project A, and vice-versa.
A better tip when using virtualenv is to install virtualenvwrapper to manage all the virtualenv's that you have, easily. It's a wrapper for creating, working, removing virtualenv's.
- Next, make a virtual environment to start working. Observe that we haven’t yet installed Django. After this is done, here is the terminal:
Once this completes, you will see a new directory of virtualenv, in my case, with name Stephen:
- Activate your virtualenv:
source bin/activate
- Once this is done, you’ll see the following:
Finally, we’re ready to install Django. We created a virtual environment first so that we can use separate Django versions for different projects.
See, how easy was that.
Creating a Django Project
Now that we are already using a virtual environment and are have finished installing Django, we can run some simple commands to create a project in Django.
Note that we’re still in our virtualenv. Run the above set of commands to create a project called hello_django and an app inside it, named as ‘hello’. Once this is done, you’ll see the following directory structure:
manage.py is a file that exists in a project environment and is created after starting a project. It's purpose is to control project commands. You can think of the arguments you pass to manage.py as subcommands. It is your tool for executing many Django-specific tasks (starting a new app within a project, running the development server, running your tests...).
Here is the complete content for the manage.py file:
#!/usr/bin/env python
import os
import sys
if __name__ == "__main__":
os.environ.setdefault("DJANGO_SETTINGS_MODULE", "hello_django.settings")
try:
from django.core.management import execute_from_command_line
except ImportError:
# The above import may fail for some other reason. Ensure that the
# issue is really that Django is missing to avoid masking other
# exceptions on Python 2.
try:
import django
except ImportError:
raise ImportError(
"Couldn't import Django. Are you sure it's installed and "
"available on your PYTHONPATH environment variable? Did you "
"forget to activate a virtual environment?"
)
raise
execute_from_command_line(sys.argv)
Understanding this will be a common focus for us in coming lessons as this is one of the most important file in a project, apart from Django-admin.py. Django-admin.py is a script to start a project (you can put it anywhere, or shortcut it via .bash or .profile).
Running the project
Finally, you’re ready to run your project.
To start your project, you need to:
- Apply migrations
- Run the project on a local server
Both of these things can be done using following commands:
python manage.py migrate
python manage.py runserver 8001
Running above commands should like following:
Finally, when you visit the URL http://127.0.0.1:8001/, you should see the following page:
Excellent, it worked!
Summary
In this lesson, we learned how to keep our project modular and even keep separate versions for Django in our various projects using Virtual Environments. Well, this was awesome, and I just realised how much we learned in a single lesson.
I hope I was descriptive as possible here, but feedback is always welcome. Share your thoughts what can be improved in the comments below and don’t be shy to ask any questions, because they show that you actually understood what was taught!
Recent Stories
Top DiscoverSDK Experts
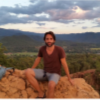
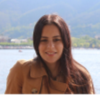
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment