ES2019 Create Objects from Arrays with fromEntries
One of the best new features in ES2019 is fromEntries—a wonderful new method that’s easy to understand and really helps in converting array key/value pairs into objects. But why would we even need to convert an array into objects? Well, if you’re interfacing to various APIs with some Node.js based Middleware, then you would need to make this type of conversion. Or, if you’re interfacing in a client application to an API that you don’t have control over, that’s another example of when you’d need to convert an array to objects. If you’re importing components to a certain place (doesn’t matter if it’s in Angular, React, or Vue) then you need this type of conversion.
The latest additions from the ES6 standard and on contain have a few important additions to conversions which until now have been housed in the Lodash library (an ultra-popular library that you should really get to know as it contains lots of useful JavaScript tools). One of these important additions is fromEntries. What it does is to take an array and return an object. Easy as could be:
const children = [['Omri', 18], ['kfir', 12], ['Daniel', 9], ['Michal', 7]];
const childrenObject = Object.fromEntries(children);
console.log(childrenObject);
/*
Object {
Daniel: 9,
kfir: 12,
Michal: 7,
Omri: 18
}
*/
I pass an array and an object is created from it. So fun, so easy…
You can use this, for example, when filtering an object. Now, it’s true there are actually lots of methods for filtering, sorting, and mutation of arrays. How do we work with them with objects? Convert the object to an array using entries or keys. With fromObject we can convert it back easily.
Here’s an example in which I make a search function in an object that returns whatever I want inside a client object. You can see how I convert the object to an array so that I’ll be able to work with it in the array methods filter and entries, and then convert it back using fromObject:
const customer = {
firstName: 'Ran',
lastName: 'Bar-Zik',
street: 'Kaplan St',
city: 'Petah Tiqwa',
age: 42,
}
function pick(object, ...keys) {
const objectEntries = Object.entries(object);
// [ ["firstName", "Ran"], ["lastName", "Bar-Zik"], ["street", "Kaplan St"], ["city", "Petah Tiqwa"], ["age", 42] ]
const filteredEntries = objectEntries.filter(
// This arrow function syntax returns the result
([key]) => keys.includes(key)
);
// [["firstName", "Ran"], ["lastName", "Bar-Zik"]]
return Object.fromEntries(filteredEntries);
}
let result = pick(customer, 'firstName', 'lastName'); // {firstName: "Ran", lastName: "Bar-Zik"}
result = pick(customer, 'city', 'street'); // {street: "Kaplan St", city: "Petah Tiqwa"}
result = pick(customer, 'age'); // {age: 42}
It’s really simple and easy. Just remember: Do you have an array? Do you want to convert it to an object? You can do it easily with what’s been added to ES2019.
One final important note: If it’s not working on Chrome for you, make sure you’re on Chrome version 73 or higher. In the real world, you should of course be using Babel.
That’s all for now. More ES2019 coming soon.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen
Recent Stories
Top DiscoverSDK Experts
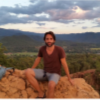
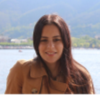
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment