ES2018 - RegEx Named Capture Groups
As you know (or maybe don’t know), the new ES2018 standard of JavaScript contains more than a few new features connected to regular expressions. RegEx is used quite a bit in JavaScript, especially in Node.js, and the new standard has lots of help in this area. This feature is one of the better ones and I believe we’ll be seeing it used in a lot of code.
But first off, let’s talk about groups! Let’s say I get some text that is a name—John Smith, or Ran Bar-Zik for example. How do I extract the first name and last name? Easy—the first part of the text up until the space is the first name, and text that comes after the space is the last name. And how do I say to RegEx that I want the first part which is all the letters from the first one until the first space, and then the second part which is everything after the space to the end? It’s pretty easy using groups in RegEx. Here’s an example:
^([a-zA-Z]+) (.+)$
OK now, don’t panic. It’s actually easier than it looks. We’ll start with the ‘^’ which is the start of the line and then the expression ‘[a-zA-Z]’, which marks all letters, lowercase and capital, in English. After that? The ‘+’ is for one or more, in this case of the characters of the expression it proceeds. A space is a space, and then a ‘.’ is for any character (note that in last names we may find a space, a hyphen, apostrophe, etc.) and then the ‘+’ is again for one or more. Then we wrap it up with a ‘$’ to end the line. The parentheses mark the groups. Need more explanation?
What’s important here is the groups. How can I extract them with JavaScript? In the past we needed to do something like this:
const name = 'Ran Bar-Zik';
const matchObj = /^([a-zA-Z]+) (.+)$/.exec(name);
const firstName = matchObj[1]; // Ran
const lastName = matchObj[2]; // Bar-Zik
console.log(matchObj)
In the code above we have to use the method exec search for a match, and then from that, we can find the groups. It seems a little like voodoo here and the code is not so readable. That was the situation up until recently. But now, I can designate each group with a….. name!
How does it work? Easy peasy lemon squeezy. All we need is a:
?
By inserting a ? into the expression, we can give it a name. We’ll also use the groups property from match.
This example should clear it up:
const name = 'Ran Bar-Zik';
const matchObj = /^(?<firstname>[a-zA-Z]+) (?<lastname>.+)$/.exec(name);
const firstName = matchObj.groups.firstName; // Ran
console.log(firstName);
const lastName = matchObj.groups.lastName; // Bar-Zik
console.log(lastName);
</lastname></firstname>
Amazing, no? If the group doesn’t exist we get back undefined, which is for debugging. It’s much easier to understand something like matchObj.groups.lastName rather than [matchObj[2, no?
Check back soon for more of the latest JavaScript features in ES2018.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen
Recent Stories
Top DiscoverSDK Experts
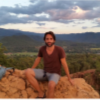
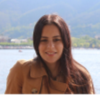
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment