ES2017 - Padding
This feature, which is really very simple, has a story that starts with a pretty significant scandal in the world of JavaScript. In order not to bore those of you who are not interested in the juicy story behind this feature, I’ll explain how the feature works first, and then tell the story of how it came to be.
The feature is an addition to the String prototype method, which is used to add text to the left of existing text. For instance, if I have a text string called x, but I want it to be ____x (a string of five characters in length with four underscores before the x) I can write something like this:
let myString = 'x'
let padMyString = myString.padStart(5, '_')
console.log(padMyString); //____x
The padStart method is unique to text string objects and adds to the left side of the text whatever we tell it to. In this case it takes two arguments. The second is the character or characters that we want to add, and the first is the total length of the string that we want in the end. Here’s another example:
let myString = 'x'
let padMyString = myString.padStart(5, 'abc')
console.log(padMyString); // abcax
Here the first argument is 5, meaning the final string will be five characters in length. The text string we want to add before is abc. This text string will repeat itself until the final string gets to five characters. So here, there’s the first abc (the first three characters), then another a which is the abc repeating (now we have four characters) and then the original string x, which is of course one character in length. Altogether, five characters.
The second argument is optional. If we don’t put anything in it, it will put a space for us. Here’s an example:
let myString = 'x'
let padMyString = myString.padStart(5)
console.log(padMyString); // x
And just like we have padStart, we also have padEnd. It works exactly the same except it adds to the right of the text. Check it out:
let myString = 'x'
let padMyString = myString.padEnd(5, 'abc')
console.log(padMyString); // xabca
You can mess around with it in this codepen I made:
Because we’re talking about a new object to string object, if we want to use it then a Babel plugin won’t help us—we’ll need to use a polyfill.
And now for the juicy story…
If you pry through the TC39 documents or through the high-brow discussions surrounding this feature, which were conducted by respected engineers and researchers, you won’t find the real and rather embarrassing reason for this feature. It all started in 2016 when Kik interactive contacted a Node.js developer by the name of Azer Koçulu, and demanded that he remove one of the modules he developed which was used in npm (the repository of modules for Node.js). The reason was that his module was also called Kik. The module was older than the company, and Azer, justifiably so IMHO, told Kik to go to hell, more or less.
Kik’s lawyer turned to npm and demanded the removal of the module. Npm, sadly enough, met all of Kik’s demands instead of telling them where to stick it. Azer, who explained his side of things in a nice post, decided to remove all of his modules from npm.
One of his modules was leftpad. A simple module that does exactly what padStart does in 11 lines of code. The problem was that almost every module of npm used it (or used a module that used it) because it’s simple and easy. The removal of this module from npm caused pretty much every module in the entire world to stop working. Fun!
Then the real shit-storm started—hundreds of thousands of builds and installations of products and services around the world started to break, one after the other. Even the product of your faithful author stopped working at the time. And one of the services that stopped working, quite ironically, was Kik’s! Npm’s managers needed to find a solution to replace the missing module. It caused a pretty big embarrassment in the JS world, with the rage of many a developer aimed squarely at Kik, which has apologized by now and also shoot the stupid lawyer that started the whole trouble. (I probably made up that part about them shooting the lawyer.) “Surprisingly,” one of the first things to go into the 2017 standard was the ability to inherently use left or right padding in the language.
If you don’t believe me, just check it out on Google yourself. Or check out this article that sums it all up.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
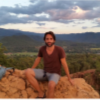
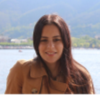
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment