ECMAScript 6 - Using Arguments
In a previous article we talked about lambda functions. In this article I’ll cover a few things that are a little lighter and that you’ll probably be able to use right away.
Default Function Values
One thing that alway bothered me in JavaScript was that you can’t give default values to variables. For example, if I have a function that receives three arguments, I can’t set it so that if the user calles it with one of the arguments, then two arguments will get the default value. I’d always have to use something complex like this:
function myFunction (x, y, z) {
if (y === undefined) {
y = 'moshe';
}
if (z === undefined) {
z = 'yaakov';
}
};
So I’d have to check the additional arguments and if they were undefined, then I would give them the default value. Pretty annoying. OK, but no more! With ECMAScript 6 we can set everything.
function myFunction (x, y = 'moshe', z = 'yaakov') {
console.log(x); //ran
console.log(y); //moshe
console.log(z); //yaakov
};
myFunction('ran');
Feel free to play around with this:
REST Parameters
With ES6, it’s also easier to know what the additional arguments are that the user enters. As we know, in JavaScript we can pass as many arguments as we want to a function. The question is, how do we find them? In the past, we needed to do something like this:
function myFunction(x) {
var additionalArgs = Array.prototype.slice.call(arguments, 1);
console.log(additionalArgs);
};
myFunction('ran', 'moshe', 'yaakov', 'sara'); //["moshe", "yaakov", "sara"]
Here, I need to change the number that appears in slice according to the number of arguments. It’s very nice but also pretty clumsy. In ECMAScript 6 is simple and easy:
function myFunction(x, ...additionalArgs) {
console.log(additionalArgs);
};
myFunction('ran', 'moshe', 'yaakov', 'sara'); //["moshe", "yaakov", "sara"]
Of course we can call the additionalArgs variable whatever we want. Pretty nice, no?
ECMAScript 6 gives us a way to take variables, objects, or strings and convert them into arrays. It’s really quite useful especially when concatenating. For instance, if I have two arrays, now it’s really easy to connect them:
var params = [ "hello", true, 7 ];
var other = [ 1, 2, ...params ];
console.log(other);// [ 1, 2, "hello", true, 7 ]
Spread Operator
We can also take an array and put it inside a variable via the spread operator. Check it!
function myFunction(x, y, z) {
console.log(x); //10
console.log(y); //20
console.log(z); //30
}
var args = [10, 20, 30];
myFunction(...args);
Don’t say that this isn’t nice and easy. It may not be a grand revolution, but without a doubt it makes life, and our code, easier.
Another nice feature in ES6 that is partly related to arguments is a slight improvement to creating objects. For example, objects that have variables inside of them. When we created an object in the past, we needed to do something like this:
var x = 10;
var y = 20;
obj = { x: x, y: y }; //{x: 10,y: 20}
That’s all well and good, but with ES6 we can be a bit more efficient when we create an object. For example:
var x = 10;
var y = 20;
obj = { x, y }; //{x: 10,y: 20}
In other words, if the name of the attribute is the same as that of the variable, then there’s no need to declare the name of the variable. Perhaps a bit esoteric but still a bit nicer.
When we define the name of a function, for instance like this:
obj = {
multiply: function (a, b) {
return a*b;
},
sum: function (x, y) {
return x+y;
}
};
console.log(obj.multiply(2,3)); //6
console.log(obj.sum(2,3)); //5
with ES6 I don’t have to explicitly indicate the function. Here’s an example of the previous code written in ES6:
obj = {
multiply (a, b) {
return a*b;
},
sum (x, y) {
return x+y;
}
};
console.log(obj.multiply(2,3)); //6
console.log(obj.sum(2,3)); //5
It’s not something so critical, but it is still pretty nice to use.
Another strange thing is the ability to use expressions in order to set the names of attributes in objects when still defining the object. Here’s an example that illustrates my opinion of this feature and how it can contribute to more readable code. Though, even if it is useful, I still wouldn’t actually use it.
obj = {
someProperty: 11,
[ "kakaBaleben" + 10 ]: 42
}; //{kakaBaleben10: 42, someProperty: 11}
That’s all for arguments and objects. There’s nothing here that doesn’t fall into the category of syntactic sugar (in other words, these aren’t features that change something essential, but rather are small changes that helps make the language more readable). But what we covered today are things that are certainly nice to know and use.
About the author: Ran Bar-Zik is an experienced web developer whose personal blog, Internet Israel, features articles and guides on Node.js, MongoDB, Git, SASS, jQuery, HTML 5, MySQL, and more. Translation of the original article by Aaron Raizen.
Recent Stories
Top DiscoverSDK Experts
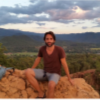
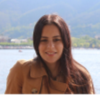
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment