Delegation in Kotlin
According to Wikipedia, delegation refers to evaluating a member (property or method) of one object (the receiver) in the context of another original object (the sender). Kotlin lets us use the delegation design pattern, which we’ll demonstrate in this article.
The keyword to use delegation in Kotlin is ‘by.’ Using this keyword allows the derived class to access implemented public methods of an interface via a specific object. Let’s look at an example of delegation in action:
interface Base {
fun printMe() //an abstract method
}
class BaseImpl(val y: Int) : Base {
override fun printMe() { println(y) } //method implementation
}
class Derived(b: Base) : Base by b // delegating the public method on the object b
fun main(args: Array<String>) {
val b = BaseImpl(15)
Derived(b).printMe() // accesses the printMe() method and prints 15
}
In our example above, the interface Base has an abstract method called ‘printme()’. We implement ‘printme()’ from the class BaseImpl. Afterward, we use this implementation from another class by using the Kotlin keyword ‘by.’ Running this block of code will give you the following output:
15
Property Delegation in Kotlin
Using the keyword ‘by’ isn’t the only way to use delegation in Kotlin. In fact, the Kotlin library offers some other methods for the delegation of properties; we’ll have a look at two of them now.
Delegation is all about passing the responsibility from one method or class to another. If a property has been declared somewhere, we can reuse the code to initialize it. There are several delegation methods that Kotlin offers us. Let’s explore two of them here with a few examples.
Using Lazy() in Kotlin
Lazy is a lambda function that takes a property as an input and in return gives an instance of Lazy<T>, where <T> is more or less the type of the properties that it’s using. Here's an example that will allow us to understand it better:
val myVar: String by lazy {
"Sometimes ... "
}
fun main(args: Array<String>) {
println(myVar +"It pays to be lazy :)")
}
In this block of code, we start by passing the variable myVar to the function Lazy. This then assigns a value to its object and then returns that value to the main function. Here is what you’ll see we you run this little code block:
Sometimes ... It pays to be lazy :)
Delegetion.Observable() in Kotlin
The other method we’ll look at here is observable. Observable() takes two arguments to initialize the object and then returns it to the called function. In our example, we’ll use the Observable() method to implement our Kotlin delegation:
import kotlin.properties.Delegates
class User {
var name: String by Delegates.observable("Welcome to Tutorialspoint.com") {
prop, old, new ->
println("$old -> $new")
}
}
fun main(args: Array<String>) {
val user = User()
user.name = "first"
user.name = "second"
}
This code block will return the following:
Welcome to DiscoverSDK.com -> first
first -> second
For the most part, the syntax is the expression after the “by” keyword is delegated. The p variable’s get() and set() methods will be delegated to its getValue() and setValue() methods that are defined in the class Delegate. Have a look at this:
class Example {
var p: String by Delegate()
}
For this bit of code, we need a delegate class to assign the value in the p variable. Here is what that might look like:
class Delegate {
operator fun getValue(thisRef: Any?, property: KProperty<*>): String {
return "$thisRef, thanks for delegating '${property.name}' to me!"
}
operator fun setValue(thisRef: Any?, property: KProperty<*>, value: String) {
println("$value has been assigned to '${property.name} in $thisRef.'")
}
}
While reading, the getValue() method is called, and while setting, the variable setValue() method is called.
That's all for this time. Check back again soon for the next article in our Kotlin series.
Previous Article: Generics in Kotlin | Next Article: Functions in Kotlin |
Recent Stories
Top DiscoverSDK Experts
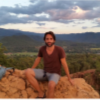
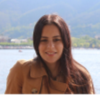
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment