Data Type Conversion In JavaScript
JavaScript is not a type-less language as thought by many people, but rather a loosely typed language. But, all data inside a JavaScript environment has some kind of type associated with it. This means that data type conversion does occasionally come into play. In this article I will show you how to convert from one data type to another.
Getting Started
As in all other tutorials on JavaScript, I am going to use NodeJs to run the code shown in this article. In the introductory article I wrote about why NodeJs is the preferred way of practicing JavaScript.
Install NodeJs on your system if you do not already have that installed. Create a js file called conv.js in your preferred working directory. Execute the file with node conv.js to ensure everything is alright. At this time the file contains nothing and you will not see any output on the screen after executing the script.
You can also run NodeJs in interactive mode for getting a REPL (Read-Eval-Print-Loop) for practicing the code interactively. To get REPL just execute the command node in the command line.
Type Checking
In JavaScript you can check the type of an object or data contained in a variable at runtime. To check the type of some variable, object, or literal value use the typeof operator with the variable, value, or object as the argument to the operator. This operator returns the type as a string.
Note: typeof in JavaScript is not a function—it an operator. But, you can use it like a function. In this case () is not used as a calling operator, but rather as an encapsulation for some code on a single line.
For example, passing "I am obviously a string" will return "string".
var val = "I am obviously a string";
var type = typeof val;
console.log(type);
Running the script from the command line with node conv.js will output the following result:
string
Below is a list of the results we get by passing different types of things to the typeof operator.
- Undefined variables or values returns "undefined"
- Null variables or values returns "object"
- Boolean variables or values returns "boolean"
- Number variables or values returns "number"
- String variables or values returns "string"
- Symbol returns "symbol"
- Any other object returns "object"
Implicit Conversion
JavaScript provides automatic type conversions. But that doesn't mean that you can sit idle and do nothing explicitly. Again, it is a best practice to convert data types explicitly to avoid bugs in your program. Often, for automatic or implicit conversion you will not get your expected result. JavaScript is a forgiving language. It will not reject the values directly and will not stop further execution. It will convert them automatically and provide you with some result. That means JavaScript coerces data types to be converted when they need to be in another form. Look at the examples below to see why it is bad to fall back to automatic type conversion or automatic coercion.
2 is a number and "2" is a string. If we add them together with the + operator JavaScript will try to coerce them to one type and then apply the operation. If JavaScript converts both of them to number then we get 4, but if JavaScript coerces them to string then we get a string "22". Fire up NodeJs REPL to run this small code:
2 + "2"
What do you see as a result? It provided us with '22'. But that behavior is not always expected or tolerated. In the example above it can be assumed that in JavaScript mathematical operations on a mix of numbers and strings will return a string. Wrong! That is not always the case.
Let's multiply 2 and "2" to prove that our initial hypothesis is wrong. Put the following code in your REPL again and see the output:
2 * "2"
Outputs:
4
This time it returned a number instead of string.
Without just assuming, let's check with the typeof operator the type of the output.
typeof (2 * "2")
Outputs:
'number'
So, automatic type conversion or type coercion is not always a good thing. But, in many places inside JavaScript environments we only need the string value and we can easily understand what the result is gonna be. For example, in a browser environment calling alert() with some value inside, it will convert the value to a string and display it to the user. After evaluating the lines of code or the statements, alert() gets a single value at the end. So, it is safe to convert a single value to a string without worrying unless otherwise warned or specified.
Explicit Conversion
As discussed in the previous section, explicit conversion is the best and safest way to go. In this section we will see different ways of converting different types of values.
Converting Values to Strings:
String is the most widely used type to which we convert different data. To send data over the network or to save it into a file we cannot send or write it as a pure original JavaScript type. We need to convert it to a string and the receiving system can optionally convert it to bytes or resort to that system's native type system.
To convert other data to string data type we can call String() with the value or variable of that data, or call the toString() method on that variable or object. Let's say we want to convert number 100 to a string. Below are demonstration of the both ways described above.
String(100)
Outputs:
'100'
With the second method:
var i = 100;
i.toString();
Or more concisely:
(100).toString();
Will output:
'100'
Converting Values to Numbers:
To convert any value to a number call Number() with the data you want to be converted to a number as the parameter. Let's say that we want to convert the string "200" to a number.
Number("200")
Outputs:
200
Another example with boolean value:
Number(true)
Will output:
1
By looking at the above example you cannot think that everything is convertible to numbers. For example you cannot convert the string "some random text" to a number. Let's try with it.
Number("some random text")
Outputs:
NaN
NaN means Not A Number—it's a way of telling us that the result is invalid but as a number was requested, this special value was returned to indicated that a number was not obtainable.
So, to convert things to numbers they need to have some characteristics that could represent a number. For example, a string with only digits is convertible to a number.
Converting Values to Booleans:
Converting values to booleans is as easy as converting values to numbers or strings. Just call Boolean() with the value as the parameter. Everything is convertible to booleans. Calling Boolean() will either return true or false. Non zero numbers, non empty strings, objects will return true. Empty stings, 0, undefined, NaN, null will result in false.
Boolean(0);
Outputs:
false
Boolean("0");
Outputs:
true
Notice the last example, "0" is a string and it is a non-empty string. So, it returned true.
Conclusions
As the final word let me suggest that you never let your code convert data implicitly, unless it is safe like when passing it to console.log(), alert(), etc.
Stop by the homepage for hundreds of JavaScript libraries, API, and SDKs.
Recent Stories
Top DiscoverSDK Experts
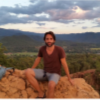
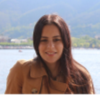
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment