Creating Your Own JavaScript Library
JavaScript became very popular on the past years. Developers write and use many javascript libraries / frameworks for their web development projects, command line tools and also embedded systems (IOT). Popular tools like GitHub and NPM make it easy to collaborate and share the work between developers
In this post I will go over the steps to create a new javascript library, host it and manage it using github, npm.
Steps:
- Create the local git and GitHub repository
- Create the NPM package
- Write and test the library locally
- Publish the code and the package
- Test your library
Create the local git and GitHub repository
Go to github.com and sign up to create an account
On the web interface create a new repository ( click the + sign on the top right)
On the loacl shell run the following commands:
echo "# minlibjs" >> README.md
git init
git add README.md
git commit -m "first commit"
git remote add origin https://github.com/DiscoverSDK/minlibjs.git
git push -u origin master
This will create and push the first (empty) version of your library minlibjs
Create the NPM package
Go to npmjs.com and sign up to create account
To work with npm you need to install node. Also on the first time you need to create a user profile file .npmrc (located on the home directory) to create it run the following commands:
npm set init-author-name 'Liran BH'
npm set init-author-email 'liran@discoversdk.com'
npm set init-author-url 'http://www.discoversdk.com'
npm set init-license 'MIT'
npm set save-exact true
To connect to the remote package manager you need to run:
npm adduser
Then create the package.json file for your library run the following command:
npm init
Enter all the information - name, version, description, entry point, test command ,git repository, keywords and license - you can add the default values. this command will create a package.json file you can always edit
{
"name": "minlibjs",
"version": "1.0.0",
"description": "simple lib",
"main": "src/index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"repository": {
"type": "git",
"url": "git+https://github.com/DiscoverSDK/minlibjs.git"
},
"keywords": [
"math"
],
"author": "Liran BH <liran@discoversdk.com> (http://www.discoversdk.$
"license": "MIT",
"bugs": {
"url": "https://github.com/DiscoverSDK/minlibjs/issues"
},
"homepage": "https://github.com/DiscoverSDK/minlibjs#readme"
}
Write and test the library
Create the source file src/index.js
var math = require('mathjs');
module.exports = {
mulNumbers: function (a,b){
return math.chain(a).multiply(b).value;
},
addNumbers: function (a,b){
return a+b;
},
subNumbers: function (a,b){
return a-b;
}
}
For learning purpose we add a dependency on external module (mathjs)
To install the external library run:
npm install --save mathjs
This will install the required library in node_modules directory and update the package.json file:
....
"dependencies": {
"mathjs": "3.4.1"
},
....
Now you are done with the library and can test it using node command line tool:
$ node
> var lib=require('./src/index.js');
> lib.addNumbers(6,7);
13
> .exit
Publish your library to GitHub and NPM
First create another file .gitignore with all the files and directories you dont want to publish (node_modules)
Now to push it to github run the following commands:
git add .
git commit –m 'basic files'
git push
And publish it to npm using the command:
npm publish
Thats it!!! you can use the library now
To creare a relese version run:
git tag 1.0.0
git push –tags
npm publish
Test the library
Create an empty directory and run inside:
npm install minlibjs
This will download and install all the required files and dependencies.
Now create a simple test.js file:
var lib=require('minlibjs');
console.log(lib.addNumbers(2,3));
To test it run using node command line tool:
node ./test.js
Version update
On each version update you should change the version number in package.json file and run the following commands (after changing and testing the code)
git add -A
git commit -m 'new version comment'
git push
git tag 1.1.0
git push --tags
npm publish
If you want to create a beta version tag it with -beta.x for example 2.0.0-beta.1 and publish it to npm with --tag beta option
Recent Stories
Top DiscoverSDK Experts
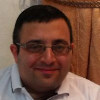
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment