Create Clean Javascript code with Self-Executing Functions
Sometimes JavaScript code can be messy since it lacks the versatility associated with a traditional “Object Oriented” language. One work around is to use of self-executing functions. But what are they? If you are new to JavaScript then you may have stumbled upon this concept and asked yourself how do I use it? What does it do? And how does it help my JavaScript code?
We’ll the answer is simple and has to do with anonymous functions, which are functions created during runtime. They are called anonymous for a reason since they function normally, in a sense that they look ordinary and you might have just see them in someone else’s code without you knowing it.
Let’s compare an ordinary function and an anonymous function.
Here we have a normal function that when invoked will print the words “bark bark”.
function callDog() {
console.log(“bark bark”);
}
callDog();
Here’s the same example created with an anonymous function.
var callDog = function() {
console.log(“bark bark”);
}
callDog();
Based from the two examples above, you can quickly spot that an anonymous function is being assigned to a variable. You might ask yourself if this is even possible? Technically yes, and that is why it is called an anonymous function considering it has no name associated with it.
Let’s put it this way. If you happen to find the keyword “function” appearing first before the function name then you can say it is being created as a function declaration.
On the other hand, anonymous functions tend to appear elsewhere in the code. In the second example, first we declared the variable, then assigned it with the function operator. So when the function operator is called, it creates a new function object. This is possible since functions in JavaScript are considered to be objects.
Once the function is stored as a variable you can now invoke it as a normal function.
callDog();
Here’s another example of an anonymous function.
(function() {
console.log("Hi");
})
Look again at the example above. Have you noticed that the function has no name? Precisely, it is an anonymous function. So you might be scratching your head right now. How am I going to call this anonymous function considering it is not stored in a variable? This is where the “self-execution” begins. To make the function work we add the (); at the end of it.
(function() {
console.log("Hi");
})();
A Function with no name is generated at runtime
The last two parentheses act as the self-executing part; they execute during runtime. The function operator can be used anywhere as long it is a valid expression.
However, function declarations are a bit different. They are not used to create anonymous functions because they already have a name.
Here’s another example of self-executing anonymous function.
(function() {
var Dog = {
bark: function() {
console.log("Bark Bark");
}
}
console.log("Hi");
})();
In this example we have an anonymous function with an object inside having its own anonymous function. Anonymousception right? It might have gotten you a bit confused there but actually it’s not that difficult to understand. Let’s head over to line 4. We have the variable Dog with some braces attached to it. Inside, the variable Dog is another anonymous function with the name bark, but it is written as an object literal in JavaScript. We can easily identify it as an object literal with the curly brackets. The bark function is an anonymous function inside the Dog object. When we run the code above we would be able to print out “Hi” in the console. Your question now should be how it’s able to run the bark function inside the Dog object?
Let’s modify the code.
(function() {
var Dog = {
bark: function() {
console.log("Bark Bark");
}
}
Dog.bark();
})();
Now if we run the code above, it will now execute the method bark since it is telling the Dog object to execute the said method. Let us modify the anonymous function even more.
(function() {
var Dog = {
init: function() {
this.bark();
},
bark: function() {
console.log("Bark Bark");
}
}
Dog.init();
})();
In this example an additional two methods were added: init() and play(). In line 18 we can see that the Dog person is calling the init() method. The same to what we did with the Dog.bark() method. As soon as the init() method is called, the bark() method is also called.
Additionally, passing parameters to self-invoking functions is possible.
(function(c, p, d) { //Body Code
}(Cat, Dog, Person);
In this case we are passing 3 objects, namely Cat, Dog, and Person. Here we hace 3 parameters being accepted when the anonymous function is executed. If you happen to be using a lot of jQuery plugins, you can see most of them are using self-executing functions in creating their plugins.
Anonymous functions are useful since they allow convenience, and in most cases you don’t have to take note of the name of the function. Every JavaScript programmer should learn to use them in most of their programs. It is a nice way to make your code cleaner and more easily readable.
Recent Stories
Top DiscoverSDK Experts
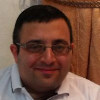
Compare Products
Select up to three two products to compare by clicking on the compare icon () of each product.
{{compareToolModel.Error}}
{{CommentsModel.TotalCount}} Comments
Your Comment